Write a program that takes as input an arithmetic expression. The program outputs whether the expression contains matching grouping symbols (brackets and parentheses). For example, the arithmetic expressions {25 + (3 – 6) * 8} and 7 + 8 * 2 contains matching grouping symbols. However, the expression 5 + {(13 + 7) / 8 - 2 * 9 does not contain matching grouping symbols. You must use a stack and/or queue. USE THE FOLLOWING DATA FILE //Header file: stackADT.h #ifndef H_StackADT #define H_StackADT template class stackADT { public: virtual void initializeStack() = 0; //Method to initialize the stack to an empty state. //Postcondition: Stack is empty virtual bool isEmptyStack() const = 0; //Function to determine whether the stack is empty. //Postcondition: Returns true if the stack is empty, // otherwise returns false. virtual bool isFullStack() const = 0; //Function to determine whether the stack is full. //Postcondition: Returns true if the stack is full, // otherwise returns false. virtual void push(const Type& newItem) = 0; //Function to add newItem to the stack. //Precondition: The stack exists and is not full. //Postcondition: The stack is changed and newItem // is added to the top of the stack. virtual Type top() const = 0; //Function to return the top element of the stack. //Precondition: The stack exists and is not empty. //Postcondition: If the stack is empty, the program // terminates; otherwise, the top element // of the stack is returned. virtual void pop() = 0; //Function to remove the top element of the stack. //Precondition: The stack exists and is not empty. //Postcondition: The stack is changed and the top // element is removed from the stack. }; #endif USE THIS TO TEST YOUR PROGRAM //Program to test the various operations of a stack #include #include "myStack.h" using namespace std; int main() { cout << "Example 18-1 shows how a stack is used." << endl; return 0; }
Write a
For example, the arithmetic expressions {25 + (3 – 6) * 8} and 7 + 8 * 2 contains matching grouping symbols. However, the expression 5 + {(13 + 7) / 8 - 2 * 9 does not contain matching grouping symbols. You must use a stack and/or queue.
USE THE FOLLOWING DATA FILE
//Header file: stackADT.h
#ifndef H_StackADT
#define H_StackADT
template <class Type>
class stackADT
{
public:
virtual void initializeStack() = 0;
//Method to initialize the stack to an empty state.
//Postcondition: Stack is empty
virtual bool isEmptyStack() const = 0;
//Function to determine whether the stack is empty.
//Postcondition: Returns true if the stack is empty,
// otherwise returns false.
virtual bool isFullStack() const = 0;
//Function to determine whether the stack is full.
//Postcondition: Returns true if the stack is full,
// otherwise returns false.
virtual void push(const Type& newItem) = 0;
//Function to add newItem to the stack.
//Precondition: The stack exists and is not full.
//Postcondition: The stack is changed and newItem
// is added to the top of the stack.
virtual Type top() const = 0;
//Function to return the top element of the stack.
//Precondition: The stack exists and is not empty.
//Postcondition: If the stack is empty, the program
// terminates; otherwise, the top element
// of the stack is returned.
virtual void pop() = 0;
//Function to remove the top element of the stack.
//Precondition: The stack exists and is not empty.
//Postcondition: The stack is changed and the top
// element is removed from the stack.
};
#endif
USE THIS TO TEST YOUR PROGRAM
//Program to test the various operations of a stack
#include <iostream>
#include "myStack.h"
using namespace std;
int main()
{
cout << "Example 18-1 shows how a stack is used." << endl;
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

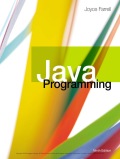
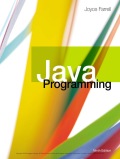