Write a program that reads the student information from a tab separated values (tsv) file. The program then creates a text file that records the course grades of the students. Each row of the tsv file contains the Last Name, First Name, Midterm1 score, Midterm2 score, and the Final score of a student. A sample of the student information is provided in StudentInfo.tsv. Assume the number of students is at least 1 and at most 20. The program performs the following tasks: Read the file name of the tsv file from the user. Open the tsv file and read the student information. Compute the average exam score of each student. Assign a letter grade to each student based on the average exam score in the following scale: A: 90 =< x B: 80 =< x < 90 C: 70 =< x < 80 D: 60 =< x < 70
PYTHON:
Write a program that reads the student information from a tab separated values (tsv) file. The program then creates a text file that records the course grades of the students. Each row of the tsv file contains the Last Name, First Name, Midterm1 score, Midterm2 score, and the Final score of a student. A sample of the student information is provided in StudentInfo.tsv. Assume the number of students is at least 1 and at most 20.
The program performs the following tasks:
- Read the file name of the tsv file from the user.
- Open the tsv file and read the student information.
- Compute the average exam score of each student.
- Assign a letter grade to each student based on the average exam score in the following scale:
- A: 90 =< x
- B: 80 =< x < 90
- C: 70 =< x < 80
- D: 60 =< x < 70
- F: x < 60
- Compute the average of each exam.
- Output the last names, first names, exam scores, and letter grades of the students into a text file named report.txt. Output one student per row and separate the values with a tab character.
- Output the average of each exam, with two digits after the decimal point, at the end of report.txt. Hint: Use the format specification to set the precision of the output.
Ex: If the input of the program is:
StudentInfo.tsv
and the contents of StudentInfo.tsv are:
Barrett Edan 70 45 59 Bradshaw Reagan 96 97 88 Charlton Caius 73 94 80 Mayo Tyrese 88 61 36 Stern Brenda 90 86 45
the file report.txt should contain:
Barrett Edan 70 45 59 F Bradshaw Reagan 96 97 88 A Charlton Caius 73 94 80 B Mayo Tyrese 88 61 36 D Stern Brenda 90 86 45 C Averages: midterm1 83.40, midterm2 76.60, final 61.60
file = open('StudentInfo.tsv', 'r')
sg = []
for lines in file:
grades = []
line = lines.split(' ')
for i in line:
grades.append(i.rstrip())
sg.append(grades)
file.close()
f1 = open('report.txt','w')
midterm1 = 0
midterm2 = 0
final = 0
for i in range(len(sg)):
string = ""
average = (int(sg[i][2])+int(sg[i][3])+int(sg[i][4]))/3
midterm1+=int(sg[i][2])
midterm2 += int(sg[i][3])
final += int(sg[i][4])
for j in range(len(sg[i])):
string += sg[i][j]+" "
if average>=90:
string+="A\n"
elif average>=80:
string+="B\n"
elif average>=70:
string+="C\n"
elif average>=60:
string+="D\n"
elif average<60:
string+="F\n"
f1.writelines(string)
f1.writelines("Averages: Midterm1 "+str(midterm1/len(sg))+", Midterm2 "+str(midterm2/len(sg))+", Final "+str(final/len(sg))+"\n")
f1.close()
I am getting an index out of range error on this particular line.
average = (int(sg[i][2])+int(sg[i][3])+int(sg[i][4]))/3
I can't see where the error is though. Can someone give me a clue so I know where to look please?

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

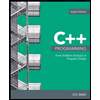
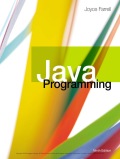
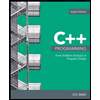
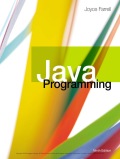