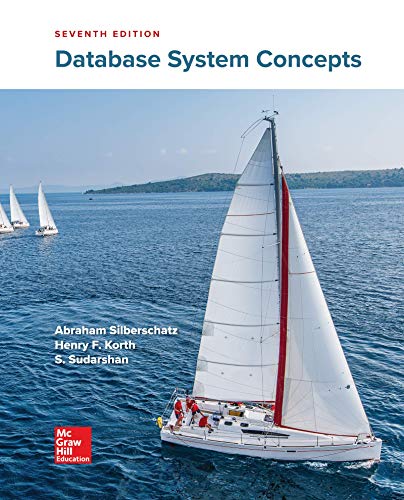
HI, I have written the code, but I get the wrong inputs over and over. I have attached the question as an image, please can anyone help?
Thank you.
//<My_Code>
import java.util.Scanner;
public class Submission {
public static void main(String[] args){
//Input of the number of rows & columns
//Creating a grid to map out the recourses
Scanner input = new Scanner(System.in);
int n = input.nextInt();
int[][] grid = new int[n][n];
for(int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
grid[i][j] = input.nextInt();
}
}
//2D integer arrays of max[num][value] collects from position
//'num' and 'value'
int[][] max = new int[n][n];
for(int j = 0; j < n; j++)
{
max[n -1][j] = grid[n - 1][j];
}
for(int i = n - 2; i >= 0; i--)
{
/***
* Inside the for-loop we insert an if statement to see
* whether they can move downwards towards right and left.
*
* Inside the two 'Else-if' we see whether they it can move only left,
* or only right respectively
*/
for(int j = 0; j < n; j++)
{
if(j - 1 >=0 && j + 1 < n)
{
max[i][j] = Math.max(max[i + 1][j + 1], max[i + 1][j - 1]) + grid[i][j];
}
else if (j - 1 >= 0 && j + 1 >= n)
{
max[i][j] = max[i + 1][j - 1] + grid[i][j];
}
else
{
max[i][j] = max[i + 1][j + 1] + grid[i][j];
}
}
}
/**
* Output of the result after comparing to each points in the first row
*/
int result = 0;
for(int j = 0; j < n; j++)
{
result = Math.max(result, max[0][j]);
}
System.out.println(result);
}
}
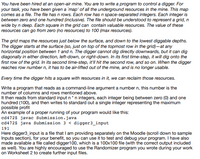

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 4 images

- Im trying ro read a csv file and store it into a 2d array but im getting an error when I run my java code. my csv file contains 69 lines of data Below is my code: import java.util.Scanner; import java.util.Arrays; import java.util.Random; import java.io.File; import java.io.FileNotFoundException; import java.io.FilenameFilter; public class CompLab2 { public static String [][] getEarthquakeDatabase (String Filename) { //will read the csv file and convert it to a string 2-d array String [][] Fileinfo = new String [69][22]; int counter = 0; File file = new File(Filename); try { Scanner scnr = new Scanner(file); scnr.nextLine(); //skips the label in the first row of the file while (scnr.hasNextLine()) { // this while loop will count the number of values in the usgs file counter += 1; // increases by one each time a line is read scnr.nextLine(); } while…arrow_forwardimport java.util.Scanner; public class ParkingFinder {/* Your code goes here */ public static void main(String[] args) { Scanner scnr = new Scanner(System.in); int numVisits; int duration; numVisits = scnr.nextInt(); duration = scnr.nextInt(); System.out.println(findParkingPrice(numVisits, duration)); }}arrow_forwardimport java.util.Scanner; public class LabProgram { public static Roster getInput(){ /* Reads course title, creates a roster object with the input title. Note that */ /* the course title might have spaces as in "COP 3804" (i.e. use nextLine) */ /* reads input student information one by one, creates a student object */ /* with each input student and adds the student object to the roster object */ /* the input is formatted as in the sample input and is terminated with a "q" */ /* returns the created roster */ /* Type your code here */ } public static void main(String[] args) { Roster course = getInput(); course.display(); course.dislayScores(); }} public class Student { String id; int score; public Student(String id, int score) { /* Student Employee */ /* Type your code here */ } public String getID() { /* returns student's id */…arrow_forward
- I need help with this code !! import java.util.Arrays;import java.util.Scanner; public class MaxElement {public static void main(String[] args) { //create an object for Scanner class Scanner x = new Scanner (System.in);System.out.print ("Enter 10 integers: ");// create an arrayInteger[] arr = new Integer[10]; // Execute for loopfor (int i = 0; i < arr.length; i++) {//get the 10 integersarr[i] = x.nextInt(); } // Print the maximum numberSystem.out.print("The max number is = " + max(arr));System.out.print("\n"); } //max method public static <E extends Comparable<E>> E max(E[] arr) {E max = arr[0]; // Execute for loop for (int i = 1; i < arr.length; i++) { E element = arr[i];if (element.compareTo(max) > 0) {max = element;}}return max; }}arrow_forwardHelp me fix the code make it run out like the EXPEarrow_forwardimport java.util.Scanner; public class CircleAndSphereWhileLoop{ public static final double MAX_RADIUS = 500.0; public static void main(String[] args) { Scanner in = new Scanner(System.in); // Step 2: Read a double value as radius using prompt // "Enter the radius (between 0.0 and 500.0, exclusive): " // Step 3: While the input radius is not in the ragne (0.0, 500.0) // Display a message on one line (ssuming input value -1) // "The input number -1.00 is out of range." // Read a double value as radius using the same promt double circumference = 2 * Math.PI * radius; double area = Math.PI * radius * radius; double surfaceArea = 4 * Math.PI * Math.pow(radius, 2); double volume = (4 / 3.0) * Math.PI * Math.pow(radius, 3); // Step 4: Display the radius, circle circumference, circle area, // sphere surface area, and…arrow_forward
- The first one is answered as follows. Help with the second one. package test;import javax.swing.*;import java.awt.*;import java.awt.event.*; public class JavaApplication1 { public static void main(String[] arguments) { JFrame.setDefaultLookAndFeelDecorated(true);JFrame frame = new JFrame("print X and Y Coordinates");frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setLayout(new BorderLayout());frame.setSize(500,400); final JTextField output = new JTextField();;frame.add(output,BorderLayout.SOUTH); frame.addMouseListener(new MouseListener() {public void mousePressed(MouseEvent me) { }public void mouseReleased(MouseEvent me) { }public void mouseEntered(MouseEvent me) { }public void mouseExited(MouseEvent me) { }public void mouseClicked(MouseEvent me) {int x = me.getX();int y = me.getY();output.setText("Coordinate X:" + x + "|| Coordinate Y:" + y);}}); frame.setVisible(true);}}arrow_forwardStringFun.java import java.util.Scanner; // Needed for the Scanner class 2 3 /** Add a class comment and @tags 4 5 */ 6 7 public class StringFun { /** * @param args not used 8 9 10 11 12 public static void main(String[] args) { Scanner in = new Scanner(System.in); System.out.print("Please enter your first name: "); 13 14 15 16 17 18 System.out.print("Please enter your last name: "); 19 20 21 //Output the welcome message with name 22 23 24 //Output the length of the name 25 26 27 //Output the username 28 29 30 //Output the initials 31 32 33 //Find and output the first name with switched characters 34 //All Done! } } 35 36 37arrow_forwardConsider the following method. /** Postcondition: m is displayed in a rectangular matrix for example: 1 2 3 4 5 6 7 8 public static void displayMatrix (int [] [] m) for (int r = 0; r < m.length; r++) for (int c = 0; c < m.length; c++) System.out.print (m[r] [c] + " "); System.out.println (); Will method displayMatrixsatisfy its postcondition? Yes, but only if the row-size and col-size are identical, No, all the numbers will display in one column. Yes, all the numbers will display in a correct rectangular display. No, because the code will generate an IndexArrayoutofBoundsException error. No, all the numbers will display in one row. ОООО Оarrow_forward
- PRACTICE CODE import java.util.TimerTask;import org.firmata4j.ssd1306.MonochromeCanvas;import org.firmata4j.ssd1306.SSD1306;public class CountTask extends TimerTask {private int countValue = 10;private final SSD1306 theOledObject;public CountTask(SSD1306 aDisplayObject) {theOledObject = aDisplayObject;}@Overridepublic void run() {for (int j = 0; j <= 3; j++) {theOledObject.getCanvas().clear();theOledObject.getCanvas().setTextsize(1);theOledObject.getCanvas().drawString(0, 0, "Hello");theOledObject.display();try {Thread.sleep(2000);} catch (InterruptedException e) {throw new RuntimeException(e);}theOledObject.clear();theOledObject.getCanvas().setTextsize(1);theOledObject.getCanvas().drawString(0, 0, "My name is ");theOledObject.display();try {Thread.sleep(2000);} catch (InterruptedException e) {throw new RuntimeException(e);}while (true) {for (int i = 10; i >= 0; i--)…arrow_forwardMy issue: I don't know how to shift left after swapping arrays. My code: import java.util.Scanner;public class StudentScores {public static void main (String [] args) {Scanner scnr = new Scanner(System.in);final int SCORES_SIZE = 4;int[] oldScores = new int[SCORES_SIZE];int[] newScores = new int[SCORES_SIZE];int i; for (i = 0; i < oldScores.length; ++i) {oldScores[i] = scnr.nextInt();} /* Your solution goes here */for (i=0; i < oldScores.length; i++) {newScores[i]= oldScores[i];}// student code ends here for (i = 0; i < newScores.length; ++i) {System.out.print(newScores[i] + " ");}System.out.println();}}arrow_forwardModify this code to make a moving animated house. import java.awt.Color;import java.awt.Font;import java.awt.Graphics;import java.awt.Graphics2D; /**** @author**/public class MyHouse { public static void main(String[] args) { DrawingPanel panel = new DrawingPanel(750, 500); panel.setBackground (new Color(65,105,225)); Graphics g = panel.getGraphics(); background(g); house (g); houseRoof (g); lawnRoof (g); windows (g); windowsframes (g); chimney(g); } /** * * @param g */ static public void background(Graphics g) { g.setColor (new Color (225,225,225));//clouds g.fillOval (14,37,170,55); g.fillOval (21,21,160,50); g.fillOval (351,51,170,55); g.fillOval (356,36,160,50); } static public void house (Graphics g){g.setColor (new Color(139,69,19)); //houseg.fillRect (100,250,400,200);g.fillRect (499,320,200,130);g.setColor(new Color(190,190,190)); //chimney and doorsg.fillRect (160,150,60,90);g.fillRect…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
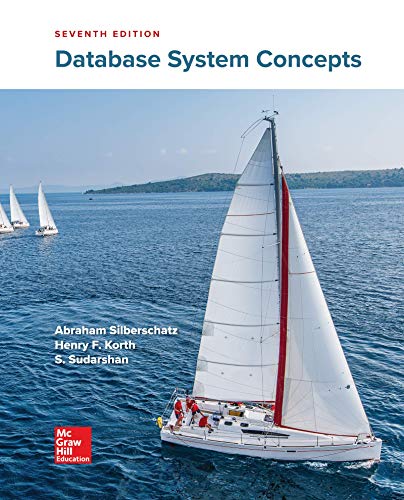
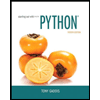
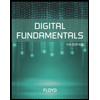
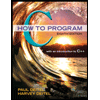
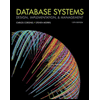
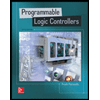