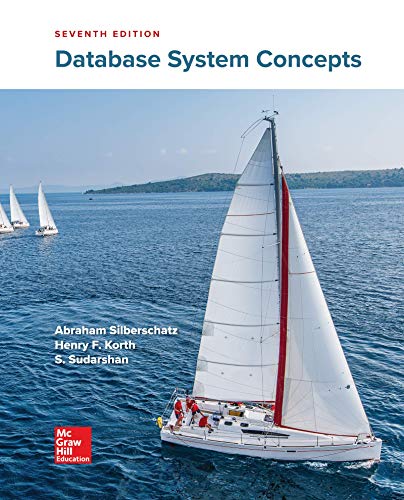
Write a program that determines how many terms 10 students need to graduate.
Step 1. Create an
Step 2. Code the program in Eclipse and ensure the following steps are accomplished.
- Define a two-dimension array with 10 rows and 2 columns.
- Prompt the user to enter the number of courses they have left to graduate (value must be between 1 and 20) and how many courses they plan to take each term (value must be between 1 and 5). If the user enters either value out of range then the user must be prompted to reenter.
- The program must store the number of courses a student has left and how many courses they plan to take each term in a different row of the array, i.e. the first student’s number of courses and how many they plan to take each term will be stored in first row of array. The second student’s info will be in second row, and so on.
- The program must compute the number of terms a student has remaining, and this should be an integer. For example, if a student has 15 courses remaining and plans to take 2 a term then they need 8 terms to complete the courses. In other words, you can have 7.5 terms because the student must attend an entire term.
Step 3. Test your program using this data.
Student 1, 8 courses and plans to take 2 a term
Student 2, 15 courses and plans to take 3 a term
Student 3, 6 courses and plans to take 1 a term
Student 4, 12 courses and plans to take 6 a term note: error because 6 is too many courses per term
Student 4, 12 courses and plans to take 5 a term
Student 5, 13 courses and plans to take 2 a term
Student 6, 7 courses and plans to take 3 a term
Student 7, 9 and plans to take 4 a term
Student 8, 3 and plans to take 2 a term
Student 9, 5 courses and plans to take 4 a term
Student 10, 18 courses and plans to take 3 a term
Your output should look like the sample below. (See attached Image)
![<terminated> C8_Project (1) [Java Application] C:\Program Files\Javaljre1.8.0_131\bin\javaw.exe (Mar 26, 2019, 12:55:57 PM)
Enter classes remaining and taking each term for student 1: 8 2
Enter classes remaining and taking each term for student 2: 15 3
Enter classes remaining and taking each term for student 3: 6 1
Enter classes remaining and taking each term for student 4: 12 6
The number of classes per term for student 4 is not valid!
Enter classes remaining and taking each term for student 4: 12 5
Enter classes remaining and taking each term for student 5: 13 2
Enter classes remaining and taking each term for student 6: 7 3
Enter classes remaining and taking each term for student 7: 9 4
Enter classes remaining and taking each term for student 8: 3 2
Enter classes remaining and taking each term for student 9: 5 4
Enter classes remaining and taking each term for student 10: 18 3
AMPLE.
Student 1 needs 4 tess to graduate
Student 2 needs 5 terms to graduate
Student 3 needs 6 terms to graduate
Student 4 needs 3 terms to graduate
Student 5 needs 7 terms to graduate
Student 6 needs 3 terms to graduate
Student 7 needs 3 terms to graduate
Student 8 needs 2 terms to graduate
Student 9 needs 2 terms to graduate
Student 10 needs 6 terms to graduate](https://content.bartleby.com/qna-images/question/fc1fe66b-5fc7-4545-9708-ad9df92717d9/9eaaff8a-eab1-4a4e-9889-0ca374cb6e34/27cpz3i_thumbnail.png)

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 2 images

- JAVA Your teacher has created two arrays, each holding the results of tests, say Test 1 and Test 2. You need to create a new array which holds the averages of these two tests. You may assume that the first element of the first test array refers to the student who also has the grade in the first element of the second test, and the last element in each array are the grades the last student earned for each test. All students received grades for both tests, meaning both test arrays are of the same length. Complete the method, named makeAverage, in the class named Grades.java. There are two parameters to this method: the first is the integer array representing the grades of the first test, and the second is the array containing the grades of the second test. The new average array should be returned by the method. The grades should be treated as double variables. For example, consider the test grades for the five students in the following arrays: [ 87 ] [ 91 ] [ 76 ] [ 76 ] [ 94 ]…arrow_forwardTask - Median elements (C Langugage) Given an array of integer elements, the median is the value that separates the higher half from the lower half of the values. In other words, the median is the central element of a sorted array. Since multiple elements of an input array can be equal to the median, in this task you are asked to compute the number of elements equal to the median in an input array of size N, with N being an odd number. Requirements Name your program project4_median.c. Follow the format of the examples below. The program will read the value of N, then read in the values that compose the array. These values are not necessarily sorted. The program should include the following function. Do not modify the function prototype. int compute_median(int *a, int n); a represents the input array, n is the length of the array. The function returns the median of the values in a. This function should use pointer arithmetic– not subscripting – to visit array elements. In other…arrow_forwardWhich of the following statements are true. An array is one name for several memory locations. Memory for elements of an array is contiguous, i.e. the memory locations are side by side. Arrays may be 1, 2, 3 or even higher dimensional. All of the above statements are true.arrow_forward
- PLEASE HELP ME WITH THIS! THANK YOU! Develop a program that will define and store a student record. The data will be entered by the user. Afterwards, calculate and display the GPA of each student. You will need to use structure with array. In case you don't know how to calculate GPA, here's the formula: Sample Console Input Expected Console Output 2John Anthony10912345ECEPhysics1003Mathematics702Geology501Jose10854321CPEPhysics803Mathematics602Geology901 Enter number of students to record: 2student name: John AnthonyID number: 10912345degree: ECEcourse 1 name: Physicscourse 1 grade: 100course 1 total units: 3course 2 name: Mathematicscourse 2 grade: 70course 2 total units: 2course 3 name: Geologycourse 3 grade: 50course 3 total units: 1student name: JoseID number: 10854321degree: CPEcourse 1 name: Physicscourse 1 grade: 80course 1 total units: 3course 2 name: Mathematicscourse 2 grade: 60course 2 total units: 2course 3 name: Geologycourse 3 grade: 90course 3 total units:…arrow_forward• • Asks the user for their name and store it in an array Asks the user for their age and store it in a variable Determines how many years before they can retire at age 67. If user is 67 then it will indicate that the user should retire now. If user is over 67 it will indicate that the user should have already retired If the user is under 67 then it will indicate number of years before retirement Ends with a thank you message. Example of Output Welcome to nameage Please enter name: (user enters a value like Pat) Please enter your age: (user enters a value like 69) Hi Pat. You should already be retired. Thank you for using nameage. Bye!arrow_forwardProblem Description - JAVA PROGRAMMING Use a Two-dimensional (3x3) array to solve the following problem: Write an application that inputs nine numbers, each of which is between 1 and 10, inclusive. Display the array after the user inputs each value. Rotate/flip the array by changing places. Make the rows columns and vice versa. You have to move the elements to their new locations. Remember to validate the input and display an error message if the user inputs invalid data. Documentation and the screenshot(s) of the results. Example: 1 2 3 4 5 6 7 8 9 the result will be : 1 4 7 2 5 8 3 6 9arrow_forward
- Take arr as any integer array of any size.arrow_forwardConsider the following program specification: Write a program that will prompt the user for 10 integer values and stores them in an array. The values entered by the user only be between 0 and 100 and the program should validate these appropriately. The program should then: Display the average of the values Display the highest value in the array Display the lowest value in the array Discuss how the values should be validated. What control structures could be used? Using eclipsearrow_forwardAlert dont submit AI generated answer.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
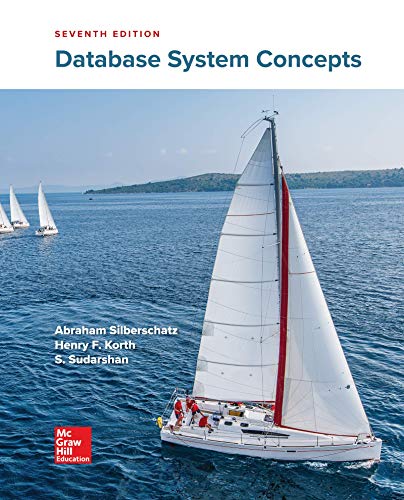
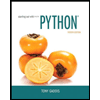
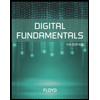
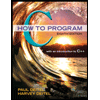
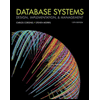
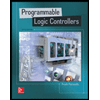