Write a program that creates an integer class Vector which represents an array of N integers allocated on the heap . The class should contain the following member data and member/friend functions: Data: Integer pointer that points where the data is stored on the heap Size of the vector (i.e. #of valid data points) Capacity of the vector (total #of data points available) Member functions Default constructor – defines size(0), capacity = size + 5 & allocates memory on heap Parametrized constructor – defines size(N), capacity = N + 5, allocates memory on heap and initializes all data to a default value X or 0 Copy constructor Destructor which removes data from heap Overloaded assignment operator=( ) Overloaded multiply operator*( ) which multiplies pairwise all the elements of two vectors; V1*V2 Overloaded scalar multiply operator*(int scalar) that multiplies all elements of a vector by scalar; V1*scalar friend function operator(int scalar, Vector &rhs); scalar*V1 Overloaded operator[K] – returns the Kth element of the vector Overloaded operator[K] – which sets the Kth element to a value X friend function ostream& operator<<(ostream &os, const Vector &rhs) – which outputs all the valid elements of the vector All constructors and destructors should print respective message: “default constructor”, “parametrized constructor”, “destructor”, “copy constructor” Demonstrate the following items: Vector V1(8, 5), V2(8, 7), V3, V4(10, 8) Print out V1, V2, V3, V4 (nicely formatted) V3 = V1*V2; //prints the contents of V3 V3 = V1*10; //print the contents of V3 V3 = 10*V1; //prints the contents of V3 V4 = V1; V2[6] = 25; //prints the contents of V2 V3[i] = V2[i]; //for i = 0; i < N V2[8] = 44; //append the value 44 to V2 & print a updated V2 -----------------------------------------------------------
Types of Linked List
A sequence of data elements connected through links is called a linked list (LL). The elements of a linked list are nodes containing data and a reference to the next node in the list. In a linked list, the elements are stored in a non-contiguous manner and the linear order in maintained by means of a pointer associated with each node in the list which is used to point to the subsequent node in the list.
Linked List
When a set of items is organized sequentially, it is termed as list. Linked list is a list whose order is given by links from one item to the next. It contains a link to the structure containing the next item so we can say that it is a completely different way to represent a list. In linked list, each structure of the list is known as node and it consists of two fields (one for containing the item and other one is for containing the next item address).
- Write a
program that creates an integer class Vector which represents an array of N integers allocated on the heap . The class should contain the following member data and member/friend functions:
- Data:
- Integer pointer that points where the data is stored on the heap
- Size of the vector (i.e. #of valid data points)
- Capacity of the vector (total #of data points available)
- Member functions
- Default constructor – defines size(0), capacity = size + 5 & allocates memory on heap
- Parametrized constructor – defines size(N), capacity = N + 5, allocates memory on heap and initializes all data to a default value X or 0
- Copy constructor
- Destructor which removes data from heap
- Overloaded assignment operator=( )
- Overloaded multiply operator*( ) which multiplies pairwise all the elements of two
vectors ; V1*V2 - Overloaded scalar multiply operator*(int scalar) that multiplies all elements of a vector by scalar; V1*scalar
- friend function operator(int scalar, Vector &rhs); scalar*V1
- Overloaded operator[K] – returns the Kth element of the vector
- Overloaded operator[K] – which sets the Kth element to a value X
- friend function ostream& operator<<(ostream &os, const Vector &rhs) – which outputs all the valid elements of the vector
- All constructors and destructors should print respective message: “default constructor”, “parametrized constructor”, “destructor”, “copy constructor”
Demonstrate the following items:
- Vector V1(8, 5), V2(8, 7), V3, V4(10, 8)
- Print out V1, V2, V3, V4 (nicely formatted)
- V3 = V1*V2; //prints the contents of V3
- V3 = V1*10; //print the contents of V3
- V3 = 10*V1; //prints the contents of V3
- V4 = V1;
- V2[6] = 25; //prints the contents of V2
- V3[i] = V2[i]; //for i = 0; i < N
- V2[8] = 44; //append the value 44 to V2 & print a updated V2
- -----------------------------------------------------------

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

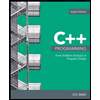
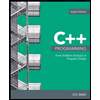