Write a Payroll class that uses the following arrays as fields: • employeeId. An array of seven integers to hold employee identification numbers. The array should be initialized with the following numbers: 5658845, 4520125, 7895122, 8777541, 8451277, 1302850, 7580489 • hours. An array of seven integers to hold the number of hours worked by each employee • payRate. An array of seven doubles to hold each employee’s hourly pay rate • wages. An array of seven doubles to hold each employee’s gross wages The class should relate the data in each array through the subscripts. For example, the num-ber in element 0 of the hours array should be the number of hours worked by the employee whose identification number is stored in element 0 of the employeeId array. That same employee’s pay rate should be stored in element 0 of the payRate array. In addition to the appropriate accessor and mutator methods, the class should have a method that accepts an employee’s identification number as an argument and returns the gross pay
Write a Payroll class that uses the following arrays as fields:
• employeeId. An array of seven integers to hold employee identification numbers. The array should be initialized with the following numbers:
5658845, 4520125, 7895122, 8777541,
8451277, 1302850, 7580489
• hours. An array of seven integers to hold the number of hours worked by each
employee
• payRate. An array of seven doubles to hold each employee’s hourly pay rate
• wages. An array of seven doubles to hold each employee’s gross wages
The class should relate the data in each array through the subscripts. For example, the num-ber in element 0 of the hours array should be the number of hours worked by the employee whose identification number is stored in element 0 of the employeeId array. That same employee’s pay rate should be stored in element 0 of the payRate array. In addition to the appropriate accessor and mutator methods, the class should have a method that accepts an employee’s identification number as an argument and returns the gross pay for that employee.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

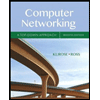
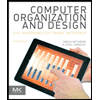
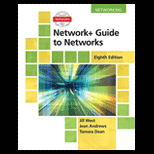
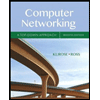
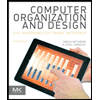
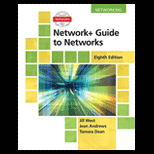
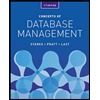
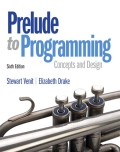
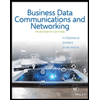