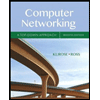
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Write a method to return the reverse order for a list of space separated words.
Example: "A sample sentence is required" would return "required is sentence sample A"
I have this to start:
class Solution:
def reverse_words(self, input):
# Your code goes here
These are the tests:
from solution import Solution
import unittest
class SolutionTests(unittest.TestCase):
def test1(self):
solution = Solution()
self.assertEqual(solution.reverse_words("the quick brown fox"), "fox brown quick the")
if __name__ == '__main__':
unittest.main()
Any help would be appreciated!
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 4 steps with 2 images

Knowledge Booster
Similar questions
- import java.util.HashSet; import java.util.Set; // Define a class named LinearSearchSet public class LinearSearchSet { // Define a method named linearSearch that takes in a Set and an integer target // as parameters public static boolean linearSearch(Set<Integer> set, int target) { // Iterate over all elements in the Set for () { // Check if the current value is equal to the target if () { // If so, return true } } // If the target was not found, return false } // Define the main method public static void main(String[] args) { // Create a HashSet of integers and populate integer values Set<Integer> numbers = new HashSet<>(); // Define the target to search for numbers.add(3); numbers.add(6); numbers.add(2); numbers.add(9); numbers.add(11); // Call the linearSearch method with the set…arrow_forwardA programmer is designing a program to catalog all books in a library. He plans to have a Book class that stores features of each book: author, title, isOnShelf, and so on, with operations like getAuthor, getTitle, getShelf Info, and set Shelf Info. Another class, LibraryList, will store an array of Book objects. The LibraryList class will include operations such as listAll Books, addBook, removeBook, and searchForBook. The programmer plans to implement and test the Book class first, before implementing the LibraryList class. The program- mer's plan to write the Book class first is an example of (A) top-down development. (B) bottom-up development. (C) procedural abstraction. (D) information hiding. (E) a driver program.arrow_forwardWrite a program that lists all Fibonacci numbers that are less than or equal to the number k (k≥2) entered by the user. Definition:The Fibonacci sequence is a sequence of numbers in which each additional term is the sum of the previous two. It is based on the fact that each member of the sequence is formed by the sum of the previous two members, the sequence starting with the numbers 1 and 1. (Example 1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89, 144, 233 , 377, 610, 987 write program in c languagearrow_forward
- Task 2: Write a static method which takes an ArrayList of Strings and an integer and changes the ArrayList destructively to remove all Strings whose length is less than the integer argument. So if the integer argument is 4 and the ArrayList is: tomato cheese chips fruit butter tea buns pie The output tomato cheese chips fruit butter is:arrow_forwardTake the following list of methods: Student () { // Creates a student object with all fields blank Student (String name) { // Creates a student object with a set name } Student (String name, Array []) { } power (int x) { // returns x^2 } power (int x, int y) { // returns x^y } greet (String name). { // returns "Hello, + name + " !"; } "1 a) What are your options if you want to find the value of a number squared? Which would you choose if you wanted the method to say something to the user? b) What 'Student' would you call if you want to create a student when you don't have any input? c) What 'Student' would you call if you had a whole array of data to input into the student object? d) Why do you think this is a capability that Java has? What uses can you imagine for it?arrow_forwardThe weight of an object can be described by two integers: pounds and ounces (where 16 ounces equals one pound). Class model is as follows: public class Weight { private int pounds; private int ounces; public Weight(int p, int o) ( pounds=p+o/16: ounces = 0% 16: } Implement a method called subtract, which subtracts one weight to another. i.e. Weight w1 = new Weight(10.5): Weight w2 = new Weight(5.7): Weight w3= w1.subtract(w2):arrow_forward
- The Delicious class – iterative methods The Delicious class must:• Define a method called setDetails which has the following header: public void setDetails(String name, int price, boolean available) This must find in the ArrayList the Chicken with the given name, change itspricePerKilo to the given price parameter and set whether it is in stock or not. A value of true for the available parameter means the chicken is in stock and a value of false means it is sold out (not in stock). Note that there will only be at most one Chicken object of the given name stored in the ArrayList.• Define a method called removeChicken that takes a single String parameter representing a chicken’s name. This method must remove from the ArrayList the Chicken object (if any) with the given name. The method must return true if a cheese with the given name was found and removed, and false otherwise.The Delicious class – challenge method In the Delicious class complete the findClosestAvailable method: This…arrow_forwardSolve using Python3 and inhritance methodarrow_forwardIn python and include doctring: First, write a class named Movie that has four data members: title, genre, director, and year. It should have: an init method that takes as arguments the title, genre, director, and year (in that order) and assigns them to the data members. The year is an integer and the others are strings. get methods for each of the data members (get_title, get_genre, get_director, and get_year). Next write a class named StreamingService that has two data members: name and catalog. the catalog is a dictionary of Movies, with the titles as the keys and the Movie objects as the corresponding values (you can assume there aren't any Movies with the same title). The StreamingService class should have: an init method that takes the name as an argument, and assigns it to the name data member. The catalog data member should be initialized to an empty dictionary. get methods for each of the data members (get_name and get_catalog). a method named add_movie that takes a Movie…arrow_forward
- Complete the following class to implement all the methods in the class: public class Person { String name; int birthYear; public Person() { name = "unknown"; birthYear = 2000; } public Person(String givenName, int yearOfBirth) { //put your implementation for this method } public String getName() { //put your implementation for this method } public String changeName(String newName) { //put your implementation for this method } public int getAgeInYears(int currentYear) { //put your implementation for this method } public static void main(String[] args) { //Create a new Person object called person1; name is Ahmed, and his birth year 1995 // Create a new Person object called person2; name is Rami, and his birth year 2000 //print the age of the first person } }arrow_forwardA programmer is designing a program to catalog all books in a library. He plans to have a Book class that stores features of each book: author, title, isOnShelf, and so on, with operations like getAuthor, getTitle, getShelf Info, and set Shelf Info. Another class, LibraryList, will store an array of Book objects. The LibraryList class will include operations such as listAll Books, addBook, removeBook, and searchForBook. The programmer plans to implement and test the Book class first, before implementing the LibraryList class. The program- mer's plan to write the Book class first is an example of (A) top-down development. (B) bottom-up development. (C) procedural abstraction. (D) information hiding. (E) a driver program.arrow_forwardImplement a “To Do” list. Tasks have a priority between 1 and 9, and a description (which you can come up with on your own, say, “wash dishes”). The program is going to prompt the user to enter the tasks by running the method add_priority_description, the program adds a new task and prints the current list with priority 1 tasks on the top, and priority 9 tasks at the bottom. The program will continue to ask the user if they want to add another tasks, and repeat the add_priority_description method, or enters Q to run the quit method to quit the program. Sample output (not limited to) 1. Study for the final 1. Take the final 2. Watch Justice League with friends 3. Play ball 9. Wash Dishes 9. Clean room. There is a possibility of two tasks having the same priority. If so, the last task that was entered gets to be printed first. Use HEAP in your solution.arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
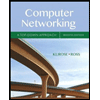
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
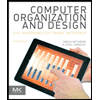
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
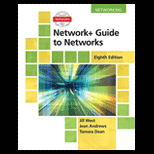
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
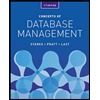
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
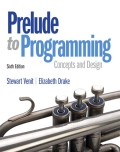
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
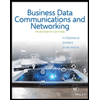
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY