Write a Java program. The program should ask the user for the name of an input file and the name of an output file. It should then open the input file as a text file (if the input file does not exist it should throw an exception) and read the contents line by line. It should also open the output file as a text file and write to it the lines in the input file, prefixed by line numbers starting at 1. So if an input file named “fred.txt” contains: Hello, I am Fred. I enrolled in JT179. Bye and the user enters “fred.txt” and “fredNum.txt”, then after running the program “fredNum.txt” will contain something like: 1 Hello, 2 3 I am Fred. 4 I enrolled in JT179. 5 6 Bye Finally, the program should display on the screen your name, the name of the output file, followed by a count of the total number of lines, the total number of words, and the total number of characters in the input file. For the above input file, the following will be displayed to the screen: My name = Joe Bloggs Name of Output file = fredNum.txt Total number of lines in fred.txt = 6 Total number of words in fred.txt = 10 Total number of characters in fred.txt = 43
Write a Java program. The program should ask the user for the name of an input file and the name of
an output file. It should then open the input file as a text file (if the input file
does not exist it should throw an exception) and read the contents line by line.
It should also open the output file as a text file and write to it the lines in the
input file, prefixed by line numbers starting at 1.
So if an input file named “fred.txt” contains:
Hello,
I am Fred.
I enrolled in JT179.
Bye
and the user enters “fred.txt” and “fredNum.txt”, then after running the
program “fredNum.txt” will contain something like:
1 Hello,
2
3 I am Fred.
4 I enrolled in JT179.
5
6 Bye
Finally, the program should display on the screen your name, the name of the
output file, followed by a count of the total number of lines, the total number
of words, and the total number of characters in the input file.
For the above input file, the following will be displayed to the screen:
My name = Joe Bloggs
Name of Output file = fredNum.txt
Total number of lines in fred.txt = 6
Total number of words in fred.txt = 10
Total number of characters in fred.txt = 43

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

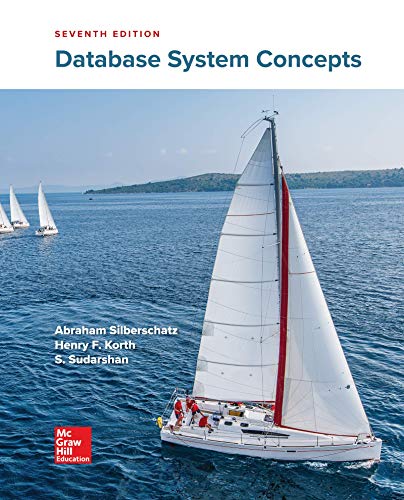
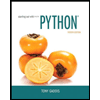
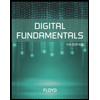
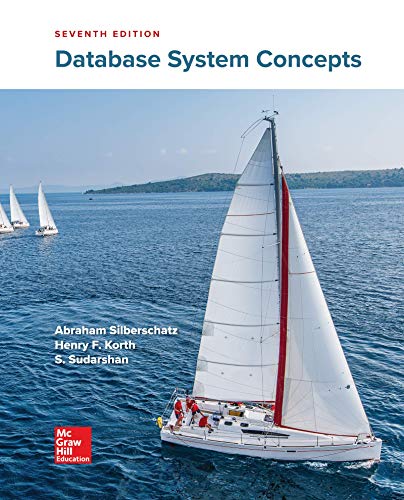
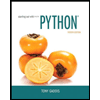
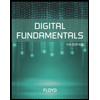
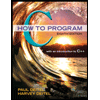
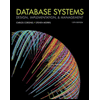
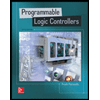