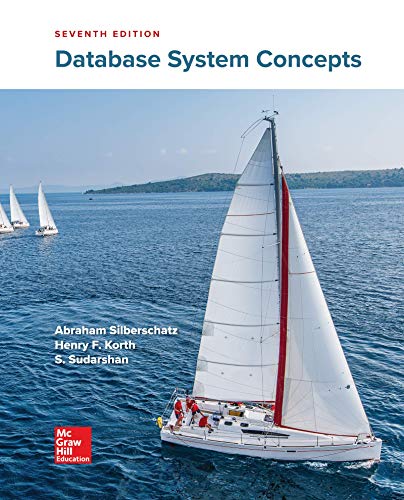
Concept explainers
Write a Java program that reads in a paragraph of text from a file and prompts the user to enter a word to search for. The program should then output the number of times the word appears in the paragraph.
Here are the basic steps to implement this program:
-
Prompt the user to enter the name of the input file.
-
Read in the paragraph of text from the input file.
-
Prompt the user to enter a word to search for.
-
Search the paragraph for the word and count the number of times it appears.
-
Output the number of times the word appears in the paragraph.
To implement step 4, you can use a similar approach to the example I provided in class:
-
Split the paragraph into an array of words using the split() method.
-
Iterate through each word in the array and compare it to the search word (ignoring case).
-
If the word matches the search word, increment a counter variable.

Step by stepSolved in 5 steps with 4 images

- Write a C++ program using classes that readslines from a file until the end of file. The program should prompt the user for the file name to read from. The program should open the file for reading, and if the filecannot be opened, print the message “File couldn’t be opened”, followed by a space and the filename, and exit. The program should keep track of the number of lines, the number of non-blank lines, the number of words, and the number of integers read from the file.arrow_forwardNeed help writing this java code, it has three objectives: to process strings to compare, search, sort, and verify location of specific pattern to output result via interface Description Write a Java program to read a text file (command line input for file name), process the text file and perform the following. Print the total number of words in the file. (When using java_test.txt, I calculate the number of words, including number, as 254.) Print the total number of different words (case sensitive, meaning “We” and “we” are two different words) in the file. (When using java_test.txt, I calculate the number of different words, including number, as 168.) Print all words in ascending order (based on the ASCII code) without duplication. Write a pattern match method to find the location(s) of a specific word. This method should return all line number(s) and location(s) of the word(s) found in the file. Print all line(s) with line number(s) where the word is found by invoking the method…arrow_forwardWrite a python program that prompts the user for their favorite basketball team. It should be able to read the list of teams provided below in a file called favorite_teams.txt and check if their team is in that file. Teams in the file:JazzBullsMavericksSpursIf the team is in the file let the user know that their team is in the list of favorites. If the team is not in the file, add the team to the end of the file. Also, let the user know that their team will be added to the file.Sample Run in File:What is your favorite NBA team? Jazz [Enter]Your team Jazz is in the listFile before and after run: JazzBullsMavericksSpurs Sample Run not in File:What is your favorite NBA team? Pelicans [Enter]Your team Pelicans is not in the list. It will be added.File before run: JazzBullsMavericksSpursFile after run:JazzBullsMavericksSpursPelicansarrow_forward
- Write a Java program that reads from a URL and searches for a given word in the URL and creates a statistic file as an output. The statistic file needs to include some information from the URL. URL address Number of words in the URL page Number of repetitions for a given word displays the number of times the word appears. You need to have two functions, one for reading from the URL and the other function for searching the word.arrow_forwardUse python 3.5 Please define main and invoke it.arrow_forwardJava Your program must read a file called personin.txt. Each line of the file will be a person's name, the time they arrived at the professor's office, and the amount of time they want to meet with the professor. These entries will be sorted by the time the person arrived. Your program must then print out a schedule for the day, printing each person's arrival, and printing when each person goes in to meet with the professor. You need to print the events in order of the time they happen. In other words, your output will be sorted by the arrival times and the times the person goes into the professor's office. In your output you need to print out a schedule. In the schedule, new students go to the end of the line. Whenever the professor is free, the professor will either meet with the first person in line, or meet with the first person in line if nobody is waiting. Assume no two people arrive at the same time. You should solve this problem using a stack and a queue. You can only…arrow_forward
- Write a program that queries information from three files(given to you). The first file contains the names and telephone numbers of a group of people. The second file contains the names and Social Security numbers of a group of people. The third file contains the names and annual income of a group of people. The groups of people should overlap. Your program should ask the user for a telephone number and then print the name, Social Security number, and annual income, if it can determine that information. Sample run1: Enter the phone number (7 digits, with a dash): 555-1234 555-1234 is associated with Bob Bob's SSN is 000300021 Bob's salary is 55000 Sample run2: Enter the phone number (7 digits, with a dash): 675-4566 Couldn't find a name associated with that number. Sample run3: Enter the phone number (7 digits, with a dash): 000-2345 000-2345 is associated with John John's SSN is 000000004 John's salary is 65000 python languagearrow_forwardWrite a program that reads in from a file a starting month name, an ending month name, and then the monthly rainfall for each month during that period. As it does this, it should sum the rainfall amounts and then report the total rainfall and average rainfall for the period. For example, the output might look like this: During the months of March-June, the total rainfall was 7.32 inches and the average monthly rainfall was 1.83 inches. Data for the program can be found in the rainfall.txt file located in the Chapter 5 º programs folder on the book's companion website. Hint: After reading in the month names, you will need to read in rain amounts until the EOF is reached and count how many pieces of rain data you read in.arrow_forwardWrite a Python program for a simple quiz game. The questions and answers are stored in a text file named "questions.txt." Each line in the file represents a question and its corresponding options and correct answer in the format: "Question, Option1, Option2, Option3, Option4, CorrectOption." The program should load the questions from the file, present them to the user one by one, and keep track of their score. Allow the user to input their answer, and after completing the quiz, display their final score. Make sure to handle cases where "questions.txt" does not exist or has incorrect formatting. Use functions to structure your code, making it easier to understand and maintain. You can use a list or dictionary to store the questions and options. Ex: questions.txt: What is the capital of France?, Berlin, London, Paris, Madrid, 3 Who is the author of "To Kill a Mockingbird"?, Mark Twain, Harper Lee, J.K. Rowling, Ernest Hemingway, 2 What is the largest mammal on Earth?, Elephant, Blue…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
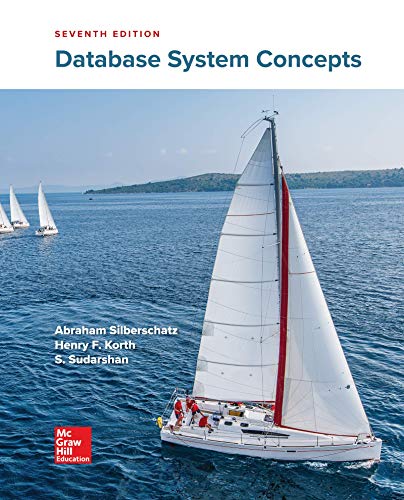
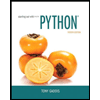
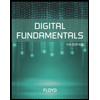
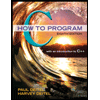
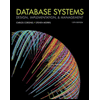
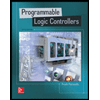