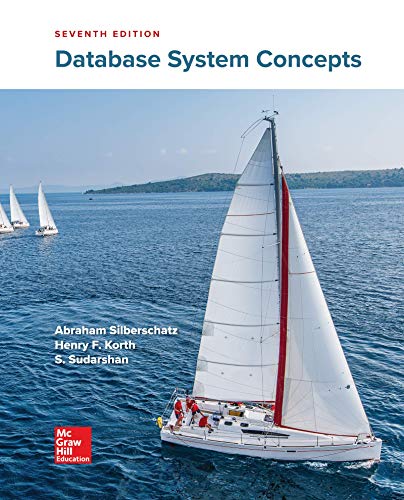
Concept explainers
consider a
1. Ask the user to choose a Klingon consonant they want to practice with. Ask again if the user’s answer is not a valid Klingon consonant, until the user enters a valid consonant.
2. Find a Klingon word that starts with the chosen consonant (the text file contains only one word that starts with any given consonant, so you don’t need to use the random library)
3. Ask the user to translate the chosen word into Klingon
4. Print "Correct" if the user’s answer is correct
5. Print "Sorry, you’re wrong!" if the user’s answer is wrong
6. Print The correct answer is ... if all three user’s answers are wrong
You will also factor in this version:
7. If the answer is incorrect, show the first hint: the first and last characters of the correct Klingon word. When showing a hint, replace all other characters with a star (*)
8. If the answer is still incorrect, show the second hint: the first and the last characters plus an extra random character of the correct Klingon word.
You must read data from the provided text file, that is absolutely essential in this lab assignment. You can not hardcode the words:
Here is the text file:
batlh|honor
cha'pujqut|dilithium crystal
De'wI'|computer
ghIgh|necklace
HablI'|data transceiving device
jabbI'ID|data transmission
laSvargh|factory
mIgh|be evil
noch|sensor
pong|name
qawHaq|memory banks
Qagh|error, mistake
rIgh|be lame
Sagh|be serious
tayqeq|civilization
voDleH|emperor
wa'leS|tomorrow
yuQHom|planetoid
'avwI'|guard
The attached pictures are example Demonstrations of how a code like this might work.
How would I start to code a program like this and which functions should I use?
Thank you very much!
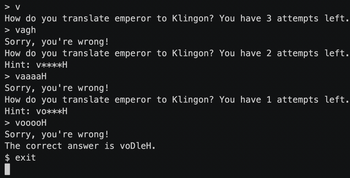
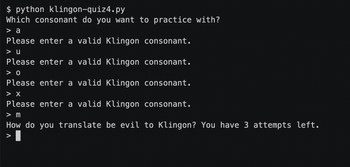

Step by stepSolved in 4 steps with 3 images

- Write a program that performs a survey on beverages. The program should prompt for the next person until a lookout value of -1 is entered to terminate the program. Each person participating in the survey should choose their favorite beverage from the following list? 1. Coffee 2. Tea 3. Coke 4. Orange Juice.arrow_forwardJava Programming: Write a program that reads five integer values from the user, then analyzes them as if they were a hand of cards. When your program runs it might look like this (user input is in orange for clarity): Enter five numeric cards, no face cards. Use 2 - 9. Card 1: 8 Card 2: 7 Card 3: 8 Card 4: 2 Card 5: 9 Pair! (This is a pair, since there are two eights). You are only required to find the hands Pair and Straight.arrow_forwardUsing python, please explain 1: A positive integer greater than 1 is said to be prime if it has no divisors other than 1 and itself. A positive integer greater than 1 is composite if it is not prime. Write a program that asks the user to enter an integer greater than 1, then displays all of the prime numbers that are less than or equal to the number entered The program should work as follows: Once the user has entered a number, the program should populate a list with all of the integers from 2 up through the value entered. The program should then use a loop to step through the list. The loop should pass each element to a function that displays the element whether it is a prime number.arrow_forward
- 1. Write a pyrhon program that prints out a classic hangman stick figure. The program should ask the user to enter a number from 1-6 and the corresponding hangman should be printed. The value the user inputs corresponds to the number of incorrect guesses in a real hangman game and so the completeness of the hangman will correspond to the number of ‘incorrect guesses’ inputted by the user (e.g., if the user enters 1, then only the head of the hangman will be printed; full example below). Example:Enter a number from 1-6: 1O Enter a number from 1-6: 2O|Enter a number from 1-6: 3O\||Enter a number from 1-6: 4O\|/|Enter a number from 1-6: 5O\|/|/Enter a number from 1-6: 6O\|/|/ \ 2. Modify your program from problem 1 so that the user input is checked to be a validsingle digit (1-6) before printing the corresponding hangman. If the input is not valid, theninstead of a hangman the following message should be printed “Invalid input: you must enter asingle number from 1-6.” Example:Enter a…arrow_forward3) Suppose an airline company has hired you to write a program for choosing the passengers who should leave an overbooked flight. That means the airline has booked too many passengers hoping some of them would not show up on time, but passengers are on board and the aircraft doesn't have enough seats available for all these passengers. Your program chooses the passenger who must leave the flight. Passengers will be ranked based on the fare they have paid for booking (a double number called fare), and their number of loyalty points (an integer number called points). Fare and points are equally important, that means, in order to decide the position of each passenger in the list, fare and points each have 50% importance. A Person with minimum priority (combination of fare and points) will leave the flight. You need to define a class called Passenger with these member variables: passenger's name • fare points. Passenger class must have 3 accessors, 3 mutators, a constructor with three…arrow_forwardIn python, write a code that allows the user to input two non-negative number sequences in increasing order (the numbers entered are always getting bigger, and no number repeats), both terminated by a -1. The size of each sequence can vary (maybe sequence - 1 has four numbers, and sequence - 2 has seven). The output of this code should be a third sequence that is a combination of both sequences 1 and 2 and is sorted in non-decreasing order. Note: Non-decreasing order means there can be a repeated number in the third sequence (see example 1). A strictly increasing order sequence cannot have repeat numbers at all (see example 3). Remember, all input sequences must be in strictly increasing order! please do this using python, only using while loops. NO language of "break" or "len" please. Hint: we can use three separate lists to solve this code.arrow_forward
- When we say arithmetic expression, we mean a kind of expression you can type in a very basic desk calculator. That is, neither variables nor brackets are allowed. It contains only numbers and four arithmetic operators, +, -, *, and /. The following are some examples of arithmetic expression: Any number string with or without signs - e.g. 3, -1, +10, 3.14, -0.70 and 099. Number strings mixed with arithmetic operators - e.g. 3+5, -1+2*3, 7/10-0.7, and -1.4-+8.2. An example of FA diagram for recognising a non-negative integer is given below. You may use it as a start point. You need to add a few more states and transitions to handle numbers with decimal point and signs (e.g. -5, +2, 0.21, -32.6, +99.05, but not the form 1.0E-3). Most importantly, you also need to add a few more things to deal with the arithmetic operators +, -, *, /.arrow_forwardWrite a program that records and displays league standings for a baseball league. The program will ask the user to enter five team names, and five win amounts. It will store the data in memory, and print it back out sorted by wins from highest to lowest. The sample output from your program should look something like this (user input in bold orange): Enter team #1: PadresEnter the wins for team #1: 75Enter team #2: DodgersEnter the wins for team #2: 91Enter team #3: GiantsEnter the wins for team #3: 92Enter team #4: Rockies Enter the wins for team #4: 65Enter team #5: DiamondbacksEnter the wins for team #5: 70League Standings:Giants: 92Dodgers: 91Padres: 75Diamondbacks: 70Rockies: 65 Requirements The data must be stored in two parallel arrays: an array of strings named teams, and an array of ints named wins. These arrays must be declared in the main() function. You can assume that the league has five teams, so each of the arrays should have five elements. As usual, you may not use any…arrow_forwardWrite a program that asks the user to enter 10 numbers, that range from 10-100. Make sure that you do not accept any numbers less than 10, if a user enters a number that is less than 10 then ask him again. Once the user enters all 10 numbers, sort the numbers entered in ascending order and print them out. CSharparrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
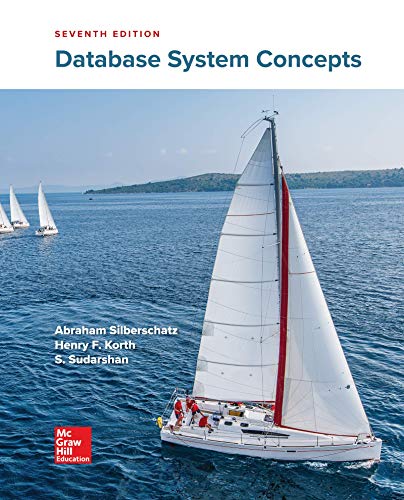
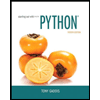
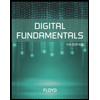
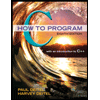
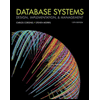
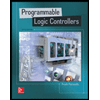