Write a java function to find minimum moves required for making 2 List equal. public int minimumOps(List S, List T){} The two possible moves are: shift(list) returns a new list after moving the front number to the back—i.e., [1, 2, 3 ,4 ] -----> [2, 3, 4, 1] reverse(list) returns the reverse of the list—i.e., [1, 2, 3, 4] -------> [4, 3, 2, 1] for example: consider S = {1, 2, 3, 4} and T = {2, 1, 4, 3}, we can see that T = shift ( shift( reverse( S ))) S = [1, 2 , 3 ,4] -----> [4, 3, 2, 1] ------>[3, 2, 1, 4] -------->[2, 1, 4, 3] = T = 3 moves total. But this is not the only way to transform S to T. To illustrate, here are some other sequence of operations : T = reverse(shift(shift(S))) T = shift(reverse(shift(S))) T = reverse(shift(shift(reverse(reverse(S)))))) Goal is to find the minimum moves on S list to form T list. Some more input/ output examples of length 5 minimumOps(List.of(1,2,3,4,5), List.of(2,1,5,4,3)) == 3 minimumOps(List.of(5,4,3,2,1), List.of(1,5,4,3,2)) == 3 minimumOps(List.of(1,2,3,4,5), List.of(5,4,3,2,1)) == 1
Write a java function to find minimum moves required for making 2 List<Integer> equal.
public int minimumOps(List<Integer> S, List<Integer> T){}
The two possible moves are:
-
shift(list) returns a new list after moving the front number to the back—i.e., [1, 2, 3 ,4 ] -----> [2, 3, 4, 1]
-
reverse(list) returns the reverse of the list—i.e., [1, 2, 3, 4] -------> [4, 3, 2, 1]
for example: consider S = {1, 2, 3, 4} and T = {2, 1, 4, 3},
we can see that T = shift ( shift( reverse( S )))
S = [1, 2 , 3 ,4] -----> [4, 3, 2, 1] ------>[3, 2, 1, 4] -------->[2, 1, 4, 3] = T
= 3 moves total.
-
But this is not the only way to transform S to T.
To illustrate, here are some other sequence of operations :
T = reverse(shift(shift(S)))
-
T = shift(reverse(shift(S)))
-
T = reverse(shift(shift(reverse(reverse(S))))))
Goal is to find the minimum moves on S list to form T list.
-
Some more input/ output examples of length 5
- minimumOps(List.of(1,2,3,4,5), List.of(2,1,5,4,3)) == 3
- minimumOps(List.of(5,4,3,2,1), List.of(1,5,4,3,2)) == 3
- minimumOps(List.of(1,2,3,4,5), List.of(5,4,3,2,1)) == 1

Step by step
Solved in 3 steps with 5 images

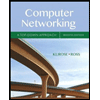
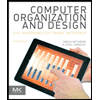
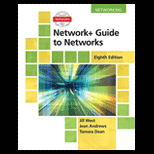
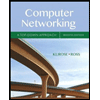
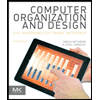
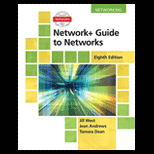
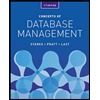
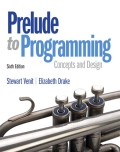
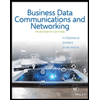