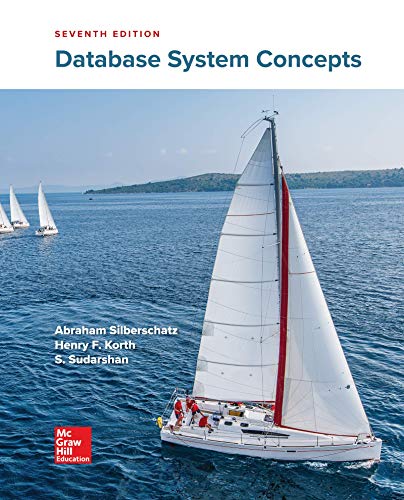
Use python when completing the question:
Write a function interleaved that accepts two sorted sequences of numbers and returns a sorted sequence of all numbers obtained by interleaving the two sequences.
Guidelines:
- each argument is either a list or a range
- assume that each argument is sorted in non-decreasing order (goes up or stays the same, never goes down)
- you are not allowed to use sort or sorted (or any other form of sorting) in your solution
- you must interleave by iterating over the two sequences simultaneously, choosing the smallest current number from each sequence.
Output:
>>> interleaved( [-7, -2, -1], [-4, 0, 4, 8])
[-7, -4, -2, -1, 0, 4, 8]
>>> interleaved( [-4, 0, 4, 8], [-7, -2, -1])
[-7, -4, -2, -1, 0, 4, 8]
>>> interleaved( [-8, 4, 4, 5, 6, 6, 6, 9, 9], [-6, -2, 3, 4, 4, 5, 6, 7, 8])
[-8, -6, -2, 3, 4, 4, 4, 4, 5, 5, 6, 6, 6, 6, 7, 8, 9, 9]
>>> interleaved( [-3, -2, 0, 2, 2, 2, 3, 3, 3], [-3, -2, 2, 3])
[-3, -3, -2, -2, 0, 2, 2, 2, 2, 3, 3, 3, 3]
>>> interleaved( [-3, -2, 2, 3], [-3, -2, 0, 2, 2, 2, 3, 3, 3])
[-3, -3, -2, -2, 0, 2, 2, 2, 2, 3, 3, 3, 3]
>>> interleaved([1,2,2],[])
[1, 2, 2]
>>> interleaved([],[1,2,2])
[1, 2, 2]
>>> interleaved([],[])
[]
>>> interleaved( range(-2,12,3), range(20,50,5) )
[-2, 1, 4, 7, 10, 20, 25, 30, 35, 40, 45]
>>> interleaved( range(20,50,5), range(-2,12,3) )==[-2, 1, 4, 7, 10, 20, 25,
30, 35, 40, 45]
True

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- C Programming Language Note: Input and Output Must be the same Write in C Languagearrow_forwardPYTHON Problem Statement Given a list of numbers (nums), for each element in nums, calculate how many numbers in the list are smaller than it. Write a function that does the calculation and returns the result (as a list). For example, if you are given [6,5,4,8], your function should return [2, 1, 0, 3] because there are two numbers less than 6, one number less than 5, zero numbers less than 4, and three numbers less than 8. Sample Input smaller_than_current([6,5,4,8]) Sample Output [2, 1, 0, 3]arrow_forwardAlert: Don't submit AI generated answer and please submit a step by step solution and detail explanation for each steps. Write a python program using functionsarrow_forward
- Python question Application: Big-O Notation (Q8-11) For each of the time complexities in this segment give the tightest bound in terms of a simple polylogarithmic function using big-O notation. Note: use the ‘^’ symbol to indicate exponents, i.e., write O(n^2) for O(n2). Question 8 (Big-O Notation 1) T(n) = n2+ log n + n Question 9 (Big-O Notation 2) T(n) = n/3 + 4 log n + 2n log(n) Question 10 (Big-O Notation 3) T(n) = 7n5 + 2n Question 11 (Big-O Notation 4) T(n) = (n%5) + 12,000arrow_forwardPrompt: In Python language, write a function that applies the logistic sigmoid function to all elements of a NumPy array. Code: import numpy as np import math import matplotlib.pyplot as plt import pandas as pd def sigmoid(inputArray): modifiedArray = np.zeros(len(inputArray)) #YOUR CODE HERE: return(modifiedArray) def test(): inputs = np.arange(-100, 100, 0.5) outputs = sigmoid(inputs) plt.figure(1) plt.plot(inputs) plt.title('Input') plt.xlabel('Index') plt.ylabel('Value') plt.show() plt.figure(2) plt.plot(outputs,'Black') plt.title('Output') plt.xlabel('Index') plt.ylabel('Value') plt.show() test()arrow_forwardComplete this code using Python m1 = [] #Matrix 1m2 = [] #Matrix 2#Write a function that will return the addition of Matrix A and B.#Create a new matrix C that will hold the addtion result of Matrix A and B (A+B).#Return the resultant matrix Cdef addMatrix(A,B):#Write your code here#Write a function that will return the subtraction of Matrix B from A.#Create a new matrix C that will hold the substraction result of Matrix B from A (A-B).#Return the resultant matrix Cdef subsMatrix(A,B):#Write your code here#Write a function that will return the multiplication of Matrix A and B.#Create a new matrix C that will hold the multiplication result of Matrix A and B (A*B).#Keep in mind,in order to perform matrix multiplication, the number of columns in Matrix A must be equal to the number of columns in Matrix B. #Return the resultant matrix Cdef multipyMatrix(A,B):#Write your code here#Write a function that will transform matrix A to the transpose of matrix A.#The transpose of a matrix means…arrow_forward
- One dimension array in C:Create an array of 100 integer elements and initialize the array elements to zero.Populate the array (using a for loop) with the values 10,20,30,..., 990,1000.Write code (using for loops) to sum all the elements and output the sum to the screen. without using #definearrow_forwardIn C++ Please summarize the differences between the following C++ sorting algorithms: Bubble Sort Merge Sort Selection Sort Insertion Sort Quick Sort Provide 2 - 3 sentences about each and your thoughts about working with the code for each. Which are easier to work with, which seem to move faster. Include the following functions when compiling the code: // Prints out an array of length 'n' // Takes an array and its length as arguments void print_array(int *array, int n){ for(int i = 0; i < n; i++){ cout << array[i] << '\t'; } cout << endl; cout << endl; } // Prints a dividing line void print_dividing_line(){ for(int i = 0; i < 72; i++){ cout << '-'; } cout << endl; } // Prints two elements being swapped and the remaining vector // on separate lines // Takes an array, index of swapping elements, and array size // as arguments void print_swap(int…arrow_forwardQ_8. Decription:- Given an array of integers, write a PHP function to find the maximum element in the array. PHP Function Signature: phpCopy code function findMaxElement($arr) { // Your code here } Example: Input: [10, 4, 56, 32, 7] Output: 56 You can now implement the findMaxElement function to solve this problems. .arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
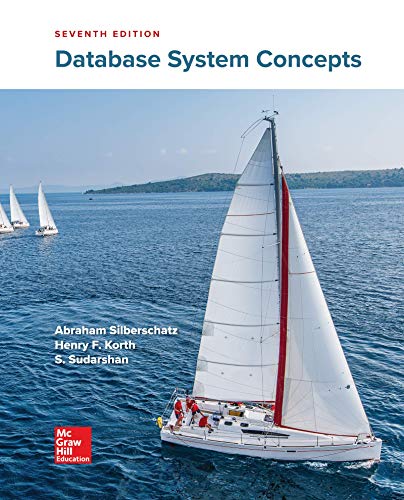
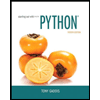
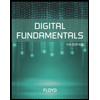
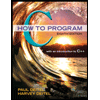
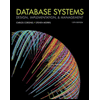
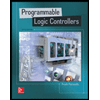