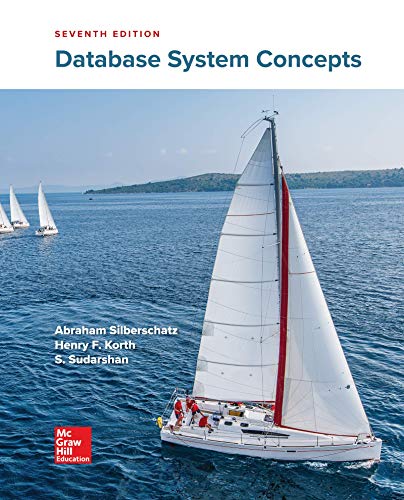
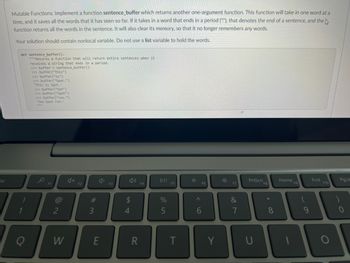

Step-1: Start
Step-2: Define function sentence_buffer()
Step-2.1: Declare variable buffer and assign ""
Step-2.2: Define function inner(word)
Step-2.2.1: Declare nonlocal buffer
Step-2.2.2: If buffer equal to "" then In buffer assign word
Step-2.2.3: else
Step-2.2.3.1: In buffer assign buffer + " " + word
Step-2.2.4: If word.endswith(".")
Step-2.2.4.1: Declare variable result and assign buffer
Step-2.2.4.2: In buffer assign ""
Step-2.2.4.3: Return result
Step-2.2.4: Return None
Step-2.3: Return inner
Step-3: In main
Step-3.1: Declare variable buffer and call function sentence_buffer()
Step-3.2: Print buffer("This")
Step-3.3: Print buffer("is")
Step-3.4: Print buffer("Spot.")
Step-3.5: Print buffer("See")
Step-3.6: Print buffer("Spot")
Step-3.7: Print buffer("run.")
Step-4: Stop
Step by stepSolved in 4 steps with 2 images

- How can I get my Python files from Blackboard and save them to send to my professor?arrow_forwardIn Python Chack the picture and fix code please. This is the Required Output example: Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and email address 5. Quit the program Enter your choice: 2 Enter name: John Enter email address: John@yahoo.com That name already exists Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and email address 5. Quit the program Enter your choice: 2 Enter name: Jack Enter email address: Jack@yahoo.com Name and address have been added Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and email address 5. Quit the program Enter your choice: 1 Enter a name: Sam The specified name was not found Menu…arrow_forwardStrict Warning ⚠️ don't you dare useany AI tool otherwise I'll report your accountarrow_forward
- (1) What's the Time Build a Python script that tells you the current time. Create a new script called current_time.py in Jupyter or a text editor. Add a docstring to the script to explain what it does. Import the datetime module. Get the current time using datetime.now(). Print the result, but only if the script is to be executed. *** hint, __name__==__main__ Execute the script in the terminal to check if it prints the time. Import the time into a Python console and check if the console output does not print the time. The output of the terminal should be in this format: HH:MM:SS.SSSSSS In [ ]: (2) Formatting Customer Names Build a function that displays a customer's name and location if applicableSuppose that you are building a Customer Relationship Management (CRM) system, and you want to display a user record in the following format: John Smith (California). However, if you don't have a location in your system, you just want to see "John Smith." Create a…arrow_forwardHello sir. Please help me How can I get the copyright information in pythonarrow_forwardPython Help Write (1) the pseudo code, (2) the code, of the following problems: An online bank wants you to create a program that shows prospective customers how their deposits will grow. Your program should read the initial balance and the annual interest rate. Print out the balance after the first three years.arrow_forward
- Code in C language. Follow instructions in photo. Use text provided as input.txt file. A1, A2 20294 Lorenzana Dr Woodland Hills, CA 91364 B1, B2 19831 Henshaw St Culver City, CA 94023 C1, C2 5142 Dumont Pl Azusa, CA 91112 D1, D2 20636 De Forest St Woodland Hills, CA 91364 A1, A2 20294 Lorenzana Dr Woodland Hills, CA 91364 E1, E2 4851 Poe Ave Woodland Hills, CA 91364 F1, F2 20225 Lorenzana Dr Los Angeles, CA 91111 G1, G2 20253 Lorenzana Dr Los Angeles, CA 90005 H1, H2 5241 Del Moreno Dr Los Angeles, CA 91110 I1, I2 5332 Felice Pl Stevenson Ranch, CA 94135 J1, J2 5135 Quakertown Ave Thousand Oaks, CA 91362 K1, K2 720 Eucalyptus Ave 105 Inglewood, CA 89030 L1, L2 5021 Dumont Pl Woodland Hills, CA 91364 M1, M2 4819 Quedo Pl Westlake Village, CA 91362 I1, I2 5332 Felice Pl Stevenson Ranch, CA 94135 I1, I2 5332 Felice Pl Stevenson Ranch, CA 94135 N1, N2 20044 Wells Dr Beverly Hills, CA 90210 O1, O2 7659 Mckinley Ave Los Angeles, CA 90001arrow_forward3 Correct and detailed answer. No copy paste answer please!arrow_forwardPython help! How do I make code that when I input my name and age (as string variables), it converts it and prints it into ascii binary and numeric binary?arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
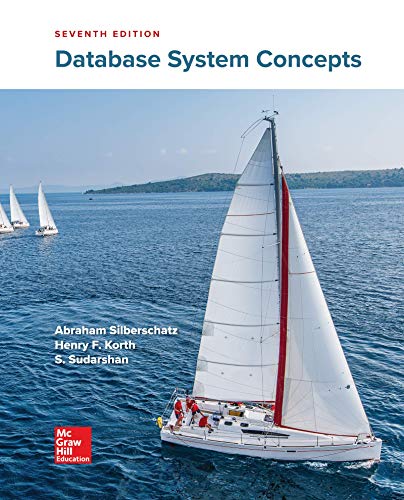
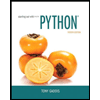
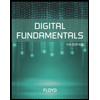
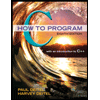
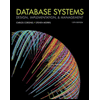
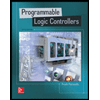