C++ Vectors Help: write a program using parallel vectors and a function which fills each of them with 500 random numbers between 1 and 100. The program should then pass both vectors to a function which will return an integer indicating a count of how many times both vectors had even numbers in the same location. So if vector01[0] contained 4 and vector02[0] contained 12, you would add one to count.If vector01[1] contained 3 and vector02[1] contained 4, you would not add one to count. main would display something like : The Vectors contain 128 cells where both values are even. Above was the question: I already have the code written: #include #include #include #include using namespace std; int main() { int even = 0; srand(time(0)); vector vector01; vector vector02; for (int i = 0; i < 500; i++) { vector01.push_back(rand() % 100 + 1); vector02.push_back(rand() % 100 + 1); if (vector01[i] % 2 == 0 && vector02[i] % 2 == 0) even++; } cout << "The vectors contain " << even << " cells where both values are even." << endl; return 0; } The code completely works, but there is just one problem. Now the question asked us to pass the vector values into a function and calculate the total even numbers, I can't figure out how to do that at all! Would you please help me with this using the basic vector code and the variables I have? Thank you!
C++
write a program using parallel vectors and a function which fills each of them with 500 random numbers between 1 and 100. The program should then pass both vectors to a function which will return an integer indicating a count of how many times both vectors had even numbers in the same location. So if vector01[0] contained 4 and vector02[0] contained 12, you would add one to count.If vector01[1] contained 3 and vector02[1] contained 4, you would not add one to count.
main would display something like :
The Vectors contain 128 cells where both values are even.
Above was the question: I already have the code written:
#include<iostream>
#include<vector>
#include<cstdlib>
#include<ctime>
using namespace std;
int main()
{
int even = 0;
srand(time(0));
vector <int> vector01;
vector <int> vector02;
for (int i = 0; i < 500; i++)
{
vector01.push_back(rand() % 100 + 1);
vector02.push_back(rand() % 100 + 1);
if (vector01[i] % 2 == 0 && vector02[i] % 2 == 0)
even++;
}
cout << "The vectors contain " << even << " cells where both values are even." << endl;
return 0;
}
The code completely works, but there is just one problem.
Now the question asked us to pass the vector values into a function and calculate the total even numbers, I can't figure out how to do that at all! Would you please help me with this using the basic vector code and the variables I have? Thank you!

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

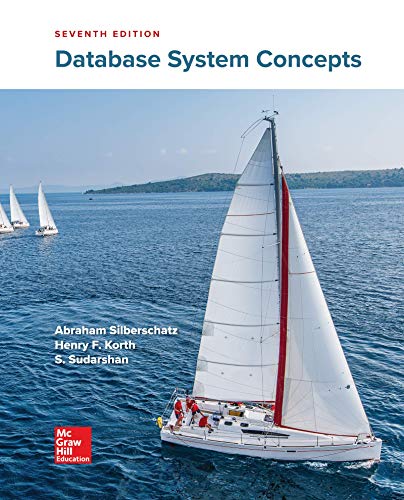
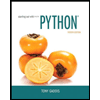
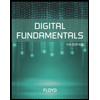
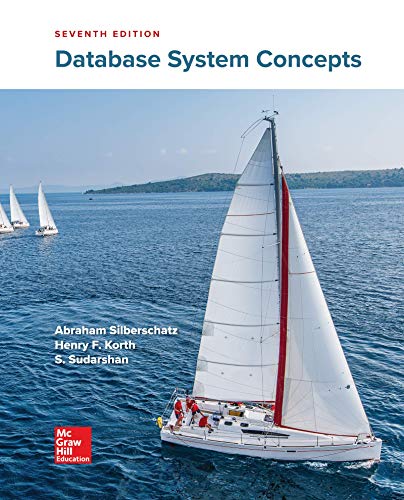
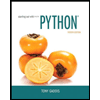
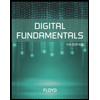
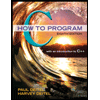
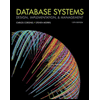
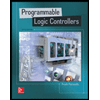