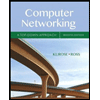
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Write a findSpelling Function -PHP
Write a function findSpellings($word, $allWords) that takes a string and an array of dictionary words as parameters. The function should return an array of possible spellings for a misspelled $word. One way to approach this is to use the soundex() Words that have the same soundex are spelled similarly. Return an array of words from $allWords that match the soundex for $word.
I have most of the code. How do I append the $sound to the $mathcing array?
![### PHP Code Explanation
This section contains a PHP script designed to find words with similar pronunciations using the `soundex` algorithm. Below is a line-by-line breakdown of the code.
```php
<?php
function findSpellings($word, $allWords) {
$matching = [];
$sounds = soundex($word);
foreach($allWords as $sound) {
if ($sounds == soundex($sound)) {
// how to add $sound to $matching
}
}
}
?>
```
**Code Details:**
1. **Opening PHP Tag:** `<?php` - Indicates the start of the PHP code block.
2. **Function Declaration:** `function findSpellings($word, $allWords)` defines a function named `findSpellings` that takes two parameters:
- `$word`: A single word to be compared.
- `$allWords`: An array of words for comparison.
3. **Initialize Array:** `$matching = [];` initializes an empty array to store words that have similar phonetic representations to `$word`.
4. **Soundex Conversion:** `$sounds = soundex($word);` computes the Soundex key of the input `$word`.
5. **Loop Through Words:** `foreach($allWords as $sound)` - Iterates over each word in the `$allWords` array.
6. **Soundex Comparison:**
```php
if ($sounds == soundex($sound)) {
```
Checks if the Soundex key of the current word in `$allWords` matches that of `$word`.
7. **Add to Matching:**
```php
// how to add $sound to $matching
```
The comment indicates where to insert code to add `$sound` to the `$matching` array if it passes the Soundex comparison.
8. **Closing Braces:** Properly closes the `foreach` loop and the function block.
9. **Closing PHP Tag:** `?>` denotes the end of the PHP code block.
This script is incomplete; it lacks logic to add matching words to the array. A possible completion would involve using `$matching[] = $sound;` within the `if` statement block.](https://content.bartleby.com/qna-images/question/2bc2a3ad-b0fb-46d0-8be8-5188cce94b42/ec0fd16f-8e3a-4d8e-8a57-88876844d14f/tdbtqmv_thumbnail.png)
Transcribed Image Text:### PHP Code Explanation
This section contains a PHP script designed to find words with similar pronunciations using the `soundex` algorithm. Below is a line-by-line breakdown of the code.
```php
<?php
function findSpellings($word, $allWords) {
$matching = [];
$sounds = soundex($word);
foreach($allWords as $sound) {
if ($sounds == soundex($sound)) {
// how to add $sound to $matching
}
}
}
?>
```
**Code Details:**
1. **Opening PHP Tag:** `<?php` - Indicates the start of the PHP code block.
2. **Function Declaration:** `function findSpellings($word, $allWords)` defines a function named `findSpellings` that takes two parameters:
- `$word`: A single word to be compared.
- `$allWords`: An array of words for comparison.
3. **Initialize Array:** `$matching = [];` initializes an empty array to store words that have similar phonetic representations to `$word`.
4. **Soundex Conversion:** `$sounds = soundex($word);` computes the Soundex key of the input `$word`.
5. **Loop Through Words:** `foreach($allWords as $sound)` - Iterates over each word in the `$allWords` array.
6. **Soundex Comparison:**
```php
if ($sounds == soundex($sound)) {
```
Checks if the Soundex key of the current word in `$allWords` matches that of `$word`.
7. **Add to Matching:**
```php
// how to add $sound to $matching
```
The comment indicates where to insert code to add `$sound` to the `$matching` array if it passes the Soundex comparison.
8. **Closing Braces:** Properly closes the `foreach` loop and the function block.
9. **Closing PHP Tag:** `?>` denotes the end of the PHP code block.
This script is incomplete; it lacks logic to add matching words to the array. A possible completion would involve using `$matching[] = $sound;` within the `if` statement block.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

Knowledge Booster
Similar questions
- Create a function in Javascript that takes two numbers as arguments (num, length) and returns an array of multiples of num until the array length reaches lengtharrow_forwardWrite a function that takes two string parameters and their common length. It fills out the second array with the capital form of the first parameter. Test your function in the main program.arrow_forwardPart 2: Unroll Write a function called unroll, which takes in a square array of arrays (i.e. a grid with n rows and n columns). An input could look like this: const square = [ [1, 2, 3, 4], [5, 6, 7, 8], ]; [9, 10, 11, 12], [13, 14, 15, 16] unroll should take in such a square array and return a single array containing the values in the square. You should obtain the values by traversing the square in a spiral: from the top- left corner, move all the way to the right, then all the way down, then all the way to the left, then all the way up, and repeat. For the above example, unroll should return [1, 2, 3, 4, 8, 12, 16, 15, 14, 13, 9, 5, 6, 7, 11, 10].arrow_forward
- Create a function using Java: Number of Rows = 3-6 Number of Columns = 3-6 Function Name: winInDiagonalFSParameters: board: 2D integer array, piece: integerReturn: booleanAssume board is valid 2D int array, piece is X==1/O==2.Look at all forward slash / diagonals in the board. If any forward slash / diagonals has at least 3consecutive entries with given type of piece (X/O) (3 in a row XXX, OOO), then return true,otherwise falsearrow_forwardWrite a function called arraySum() that takes two arguments: an integer array and the number of elements in the array. Have the function return as its result the sum of the elements in the arrayarrow_forwardWrite a function named "changeCase" that takes an array of characters terminating by NULL character (C-string) and a boolean flag of toUpper. If the toUpper flag is true, it will go through the array and convert all lowercase characters to uppercase. Otherwise, it will convert all uppercase to lowercase. For example, if the array is {'H', 'e', 'l', 'l', 'o', '\0'} and the flag is true, then the array will become {'H', 'E', 'L', 'L', 'O', '\0'}. And if the flag is false, the array will become{'h', 'e', 'l', 'l', 'o', '\0'}arrow_forward
- JAVASCRIPT Write the function divideArray() in script.js that has a single numbers parameter containing an array of integers. The function should divide numbers into two arrays, evenNums for even numbers and oddNums for odd numbers. Then the function should sort the two arrays and output the array values to the console.arrow_forwardvowelIndex Write a function vowelIndex that accepts a word (a str) as an argument and returns the index of the first vowel in the word. If there is no vowel, -1 is returned. For this problem, both upper and lower case vowels count, and 'y' is not considered to be a vowel. Sample usage: >>> vowelIndex('hello') 1 >>> vowelIndex('string') 3 >>> vowelIndex('string') 3 >>> vowelIndex('BUY') 1 >>> vowelIndex('APPLE') 0 >>> vowelIndex('nymphly') -1 >>> vowelIndex('string')==3 True >>> vowel Index ('nymphly')==-1 Truearrow_forwardt. Given a string as a parameter to the function, return a square grid filled with all the characters of the string from left to right. Fill empty spaces in the grid with '_'. Sample Input: stringtogrid Output: {{s, t, r, i}, {n, g, t, o}, {g, r, i, d}, {_, _, _, _}} Complete the function: vector<vector<int> formGrid(string s){}arrow_forward
- Create a function named "onlyOdd".The function should accept a parameter named "numberArray".Use the higher order function filter() to create a new array that only containsthe odd numbers. Return the new array.// Use this array to test your function:const testingArray = [1, 2, 4, 17, 19, 20, 21];arrow_forwardScrabble Scrabble is a game where players get points by spelling words. Words are scored by adding together the point values of each individual letter. Define a function scrabble_score (word:str) -> int that takes a string word as input and returns the equivalent scrabble score for that word. score = {"a": 1, "c": 3, "f": 4, "i": 1, "1": 1, "o": 1, "r": 1, "u": 1, "t": 1, "x": 8, "z": 10} For example, "b": 3, "h": 4, "n": 1, Your Answer: == 20 assert scrabble_score("quick") assert scrabble_score("code") == 7 1 # Put your answer here 2 Submit "e": 1, "d": 2, "g": 2, "k": 5, "j": 8, "m": 3, "q": 10, "p": 3, "s": 1, "w": 4, "v": 4, "y": 4,arrow_forwardPrompt: In Python language, write a function that applies the logistic sigmoid function to all elements of a NumPy array. Code: import numpy as np import math import matplotlib.pyplot as plt import pandas as pd def sigmoid(inputArray): modifiedArray = np.zeros(len(inputArray)) #YOUR CODE HERE: return(modifiedArray) def test(): inputs = np.arange(-100, 100, 0.5) outputs = sigmoid(inputs) plt.figure(1) plt.plot(inputs) plt.title('Input') plt.xlabel('Index') plt.ylabel('Value') plt.show() plt.figure(2) plt.plot(outputs,'Black') plt.title('Output') plt.xlabel('Index') plt.ylabel('Value') plt.show() test()arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
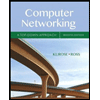
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
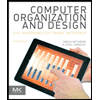
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
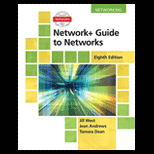
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
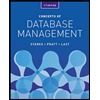
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
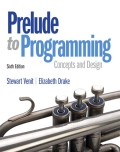
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
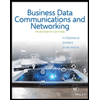
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY