Write a function named "changeCase" that takes an array of characters terminating by NULL character (C-string) and a boolean flag of toUpper. If the toUpper flag is true, it will go through the array and convert all lowercase characters to uppercase. Otherwise, it will convert all uppercase to lowercase. For example, if the array is {'H', 'e', 'l', 'l', 'o', '\0'} and the flag is true, then the array will become {'H', 'E', 'L', 'L', 'O', '\0'}. And if the flag is false, the array will become {'h', 'e', 'l', 'l', 'o', '\0'}
Write a function named "changeCase" that takes an array of characters terminating by NULL character (C-string) and a boolean flag of toUpper. If the toUpper flag is true, it will go through the array and convert all lowercase characters to uppercase. Otherwise, it will convert all uppercase to lowercase. For example, if the array is {'H', 'e', 'l', 'l', 'o', '\0'} and the flag is true, then the array will become {'H', 'E', 'L', 'L', 'O', '\0'}. And if the flag is false, the array will become {'h', 'e', 'l', 'l', 'o', '\0'}
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
- Write a function named "changeCase" that takes an array of characters terminating by NULL character (C-string) and a boolean flag of toUpper. If the toUpper flag is true, it will go through the array and convert all lowercase characters to uppercase. Otherwise, it will convert all uppercase to lowercase.
For example, if the array is {'H', 'e', 'l', 'l', 'o', '\0'} and the flag is true, then the array will become
{'H', 'E', 'L', 'L', 'O', '\0'}. And if the flag is false, the array will become
{'h', 'e', 'l', 'l', 'o', '\0'}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

Recommended textbooks for you
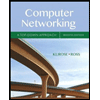
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
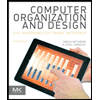
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
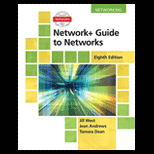
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
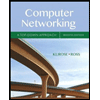
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
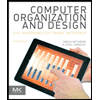
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
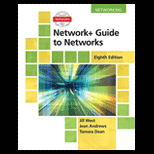
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
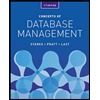
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
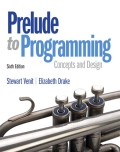
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
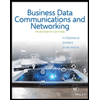
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY