write a c++ program Use a pretest loop to allow the user to enter data until they input 0 for the purchase amount. Do all of your output in main(). Make it look like this. Don’t forget your underlines won’t be immediately underneath. Use methods for the following: getPurchaseAmt() Get the monthly purchase amount from the user. Include an error-trap around this input value so the user will have to enter a positive number (0r 0 to end the loop). Return the purchase amount. displayMenu() Display a menu of the membership types for the user to choose from, as follows: Membership Types: Premium Plus Standard Enter choice (1, 2, or 3): This method only displays. There is nothing to return, so it will be a void method. You will call this method from getType(). getType() Call the displayMenu() method and get the membership type from the user. Include an error-trap around the menu and choice so the program will not continue until the user enters a valid choice. Return the choice. calcPoints() Premium members receive 3 reward points for every 100 dollars they spend, Plus members receive 2 reward points for every hundred dollars they spend, and Standard members receive 1 reward point for every 100 dollars they spend. (Hint: Integer division and type casting as int will come in handy for this). Return the number of reward points.
Types of Loop
Loops are the elements of programming in which a part of code is repeated a particular number of times. Loop executes the series of statements many times till the conditional statement becomes false.
Loops
Any task which is repeated more than one time is called a loop. Basically, loops can be divided into three types as while, do-while and for loop. There are so many programming languages like C, C++, JAVA, PYTHON, and many more where looping statements can be used for repetitive execution.
While Loop
Loop is a feature in the programming language. It helps us to execute a set of instructions regularly. The block of code executes until some conditions provided within that Loop are true.
write a c++
Do all of your output in main(). Make it look like this. Don’t forget your underlines won’t be immediately underneath.
Use methods for the following:
getPurchaseAmt()
Get the monthly purchase amount from the user. Include an error-trap around this input value so the user will have to enter a positive number (0r 0 to end the loop). Return the purchase amount.
displayMenu()
Display a menu of the membership types for the user to choose from, as follows:
Membership Types:
- Premium
- Plus
- Standard
Enter choice (1, 2, or 3):
This method only displays. There is nothing to return, so it will be a void method. You will call this method from getType().
getType()
Call the displayMenu() method and get the membership type from the user. Include an error-trap around the menu and choice so the program will not continue until the user enters a valid choice. Return the choice.
calcPoints()
Premium members receive 3 reward points for every 100 dollars they spend, Plus members receive 2 reward points for every hundred dollars they spend, and Standard members receive 1 reward point for every 100 dollars they spend. (Hint: Integer division and type casting as int will come in handy for this). Return the number of reward points.

Step by step
Solved in 3 steps with 1 images

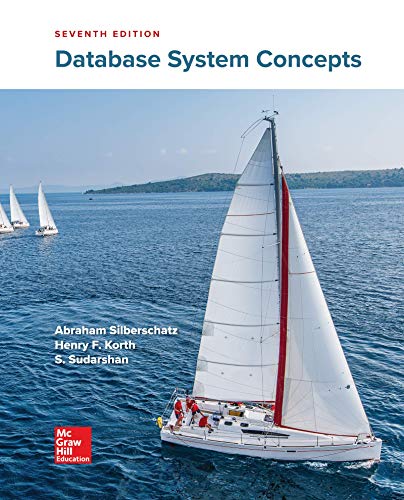
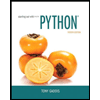
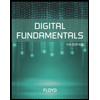
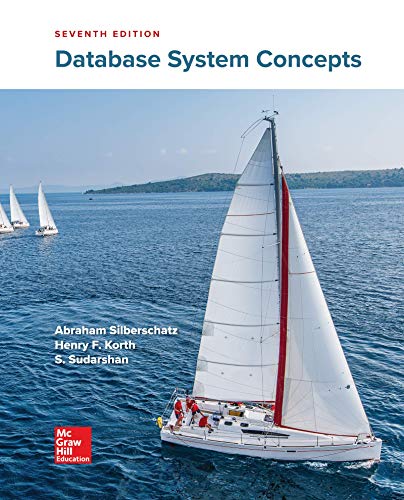
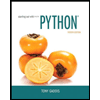
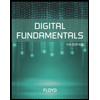
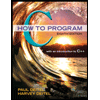
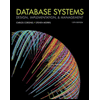
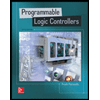