work and write the cosine function's taylor expansion (N=10) in C++ and compare the with your version (see nartual exponent EXP() vs exp()). EQUATION ATTACHED. EXAMPLE: #include #include using namespace std; // lazy technique // Factorial Recursion. // https://en.wikipedia.org/wiki/Factorial // write the factorial function as a loop (iteration) // This might seem trivial, but people have researched this // this topic. Sterling series approximation // https://en.wikipedia.org/wiki/Stirling_approximation int fact(int N) { if (N == 0) return 1; else return N * fact(N - 1); } // taylor series approximation for the // natural exponent 2.718... which is abbreviated as exp. double EXP(double x) { double sum = 0.0; for (int i = 0; i < 10; i++) sum += pow(x, i) / fact(i); return sum; } // dummy--aka place holder--functions. double SIN(double x) { return 1; } double COS(double x) { return 0; } int main() { std::cout << "e-World!\n"; for (double x = 0.0; x <= 1.0; x += .1) { cout << x << '\t' << EXP(x)<<'\t'<
work and write the cosine function's taylor expansion (N=10) in C++ and compare the <cmath> with your version (see nartual exponent EXP() vs exp()). EQUATION ATTACHED.
EXAMPLE:
#include <iostream>
#include <cmath>
using namespace std; // lazy technique
// Factorial Recursion.
// https://en.wikipedia.org/wiki/Factorial
// write the factorial function as a loop (iteration)
// This might seem trivial, but people have researched this
// this topic. Sterling series approximation
// https://en.wikipedia.org/wiki/Stirling_approximation int fact(int N) { if (N == 0) return 1; else return N * fact(N - 1); }
// taylor series approximation for the
// natural exponent 2.718... which is abbreviated as exp.
double EXP(double x) { double sum = 0.0;
for (int i = 0; i < 10; i++)
sum += pow(x, i) / fact(i);
return sum; } // dummy--aka place holder--functions.
double SIN(double x) { return 1; }
double COS(double x) { return 0; }
int main() {
std::cout << "e-World!\n";
for (double x = 0.0; x <= 1.0; x += .1) {
cout << x << '\t' << EXP(x)<<'\t'<<exp(x) << endl;
}
}


Algorithm :-
1. Start
2. Create factorial function fact(int n)
3. return (n==0) || (n==1) ? 1 : n* fact(n-1)
5. Run for loop 1 to 10 with increment of 1
6. Return sum+=( pow(x, 2*i) / fact(2*i) )*pow(-1,i) until the loop run
7. Call main function
8. Run for loop for x =0.0 to 1 with the increment of 0.1
9. Print COS(x) function and cos(x)
10. End
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

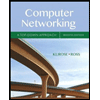
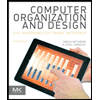
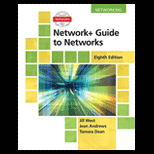
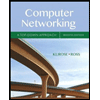
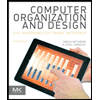
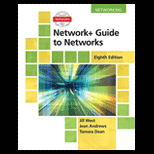
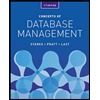
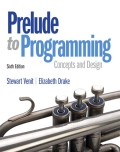
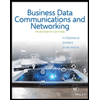