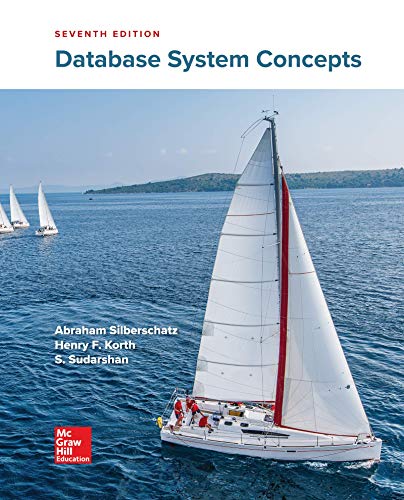
python
Write a program to draw linear lines with equation
y = mx + b
where m is the slope of the line and b is the y-intercept. Prompt user to enter the slope and y-intercept. Draw the line from x=-10 to x=10. Use numpy arange method to generate x values from -10 to 10 with step size of 0.1. For example.,
x = np.arange(-10, 10, 0.1)
Use the plt.axis() function to set the range of x and y values from -10 to 10. Add xlabel with x-axis, ylabel with y-axis, and title Perpendicular Lines.
After you have drawn the line, draw a line that is perpendicular to the first line. Hint: Two lines are perpendicular if the product of their slope is -1. For example, if the first line has slope of 5, then the perpendicular line has slope of -1/5. Use the same y-intercept for the second line.
Note: Please name the Python file perpendicular.py. The system will not accept any other name.

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- Write an improved version of the chaos . py program from Chapter 1 thatallows a user to input two initial values and the number of iterations, and then prints a nicely formatted table showing how the values changeover time. For example, if the starting values were . 25 and . 26 with 10iterations, the table might look like this: index 0.25 0.26----------------------------1 0 . 731250 0 . 7503602 0 . 766441 0 . 7305473 0 . 698135 0 . 7677074 0 . 821896 0 . 6954995 0 . 570894 0 . 8259426 0 . 955399 0 . 5606717 0 . 166187 0 . 9606448 0 . 540418 0 . 1474479 0 . 968629 0 . 49025510 0.118509 0 . 974630arrow_forwardMy instructor saying my code has wrong indentation on several lines… please let me know which lines. I’ve asked this twice before and I haven’t got it yetarrow_forwardI need to write a program for a Lion Hunt. The program should calculate the number of hours it will take a group of hunters to find the lion in the worst case scenario. We asumme that The lion has b b Hiding spots where 2 ≤ b ≤ 10 , 000 2 ≤ b ≤ 10 , 000 . We assume that there are s s Hunters where 1 ≤ s ≤ 1 , 000 1 ≤ s ≤ 1 , 000 . We assume that there are g g number of hunters, who need to be in a group to travel safely where 1 ≤ g ≤ s 1 ≤ g ≤ s . We assume that it takes a group 1 hour to search any of The lions hiding spots The input consists of a single line containing three integers ?, ? and ?, where 2≤?≤1000 is the number of hiding spots in the jungle. 1≤ e. Sample input/output is shown in attached photoarrow_forward
- The programming language used is Javaarrow_forwardWrite a program that takes in four positive integers and outputs the number of odd numbers. (Hint: use the modulo operator to determine if a number is odd) Ex: If the input is: 1 2 3 4 the output is: 2 Below is my code so far and I'm getting back unexpected EOF while parsing. I can't figure out what I'm doing wrong. odd_number=[]number_1=int(input())number_2=int(input())number_3=int(input())number_4=int(input())if number_1%2!=0: odd_number.append(number_1)else: passif number_2%2!=0: odd_number.append(number_2)else: passif number_3%2!=0: odd_number.append(number_3)else: passif number_4%2!=0: odd_number.append(number_4)else: passprint(len(odd_number)arrow_forwardUse Java language and the last digit of ID is a 7arrow_forward
- Write a Python program that uses the Sieve of Eratosthenes method to compute prime numbers up to a specified number.Note: In mathematics, the sieve of Eratosthenes, (Ancient Greek: κόσκινον Ἐρατοσθένους, kóskinon Eratosthénous) one of a number of prime number sieves, is a simple, ancient algorithm for finding all prime numbers up to any given limit..arrow_forwardI need help with creating a Java program described below: Minesweeper.Write a program that reads three numbers, m, n, and p and produces an m-by-n Booleanarray where each element is occupied with probability p. In the minesweeper game, occupiedcells represent bombs and empty cells represent safe cells.Print out the array using an asterisk for bombs and a period for safe cells.Then, create an integer two-dimensional array with the number of neighboring bombs (above,below, left, right, or diagonal). * * . . . * * 1 0 0. . . . . 3 3 2 0 0 . * . . . 1 * 1 0 0Write your code so that you have as few special cases as possible to deal with, by using an(m+2)-by-(n+2) Boolean array.arrow_forwardIt is a python program. Make sure it works and run in IDLE 3.10. Please show the code screenshot and output screenshot.arrow_forward
- please helparrow_forwardWrite a python code using IntegerRoot class that calculates integer nth root of a number m ifits nth root is an integer. For example: (m,n) where m = 1881676372240757194277820616164488626666147700309108518161,n = 3, then the integer nth root of m (i.e. the integer cubic root of m ) is: rgives an out put r = 12345678900987654321.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
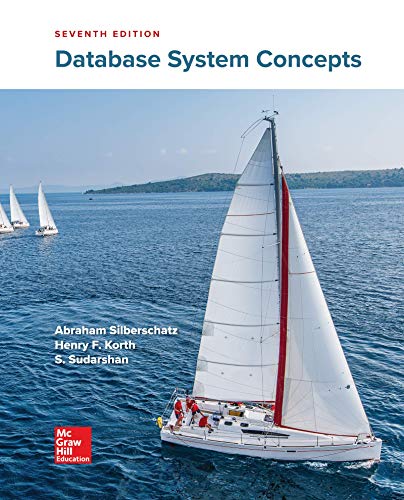
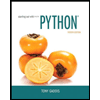
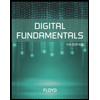
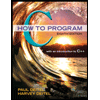
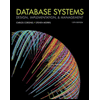
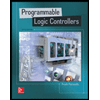