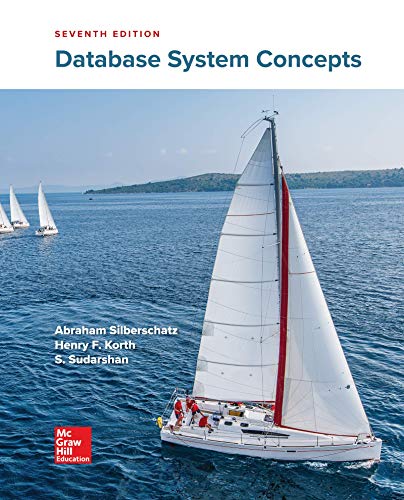
Write a program that takes in four positive integers and outputs the number of odd numbers. (Hint: use the modulo operator to determine if a number is odd)
Ex: If the input is:
1 2 3 4
the output is:
2
Below is my code so far and I'm getting back unexpected EOF while parsing. I can't figure out what I'm doing wrong.
odd_number=[]
number_1=int(input())
number_2=int(input())
number_3=int(input())
number_4=int(input())
if number_1%2!=0:
odd_number.append(number_1)
else:
pass
if number_2%2!=0:
odd_number.append(number_2)
else:
pass
if number_3%2!=0:
odd_number.append(number_3)
else:
pass
if number_4%2!=0:
odd_number.append(number_4)
else:
pass
print(len(odd_number)

Trending nowThis is a popular solution!
Step by stepSolved in 5 steps with 2 images

- in java Strings itemToFind and inputItem are read from input. Integer wordCount is initialized with 1. Write a while loop that iterates until inputItem is equal to "Done". In each iteration of the loop: Increment wordCount if inputItem is equal to itemToFind. Read string inputItem from input. Click here for example Note: Assume that input has at least two strings. 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 import java.util.Scanner; public class SimpleWhileLoop { publicstaticvoidmain(String[] args) { Scannerscnr=newScanner(System.in); StringitemToFind; StringinputItem; intwordCount; itemToFind=scnr.next(); inputItem=scnr.next(); wordCount=1; /* Your code goes here */ System.out.println(itemToFind+" occurs "+wordCount+" time(s)."); } }arrow_forwardIn the classic problem FizzBuzz, you are told to print the numbers from 1 to n. However,when the number is divisible by 3, print "Fizz''. When it is divisible by 5, print "Buzz''. When it isdivisible by 3 and 5, print"FizzBuzz''. In this problem, you are asked to do this in a multithreaded way.Implement a multithreaded version of FizzBuzz with four threads. One thread checks for divisibilityof 3 and prints"Fizz''. Another thread is responsible for divisibility of 5 and prints"Buzz''. A third threadis responsible for divisibility of 3 and 5 and prints "FizzBuzz''. A fourth thread does the numbers.arrow_forward1.Generate a list of 10 random numbers with values between 1 and 20, and submit the first 10 numbers as an answer to this question. (Use any random number generator). For the purpose of using them in the next questions, call these numbers A, B, C, D, . . . J 2.Convert A*pi /B into degrees. 3.Find the result of a. Degrees = (A \times B \times C) + 180 mod 180; this is the remainder after dividing (A \times B \times C) + 180 by 180 b. Convert Degrees into radians, and put the answer in \pi notation. 4.Calculate the measurements of the acute angles of a right triangle whose sides measure A, B, C units.arrow_forward
- Using python, please explain 1: A positive integer greater than 1 is said to be prime if it has no divisors other than 1 and itself. A positive integer greater than 1 is composite if it is not prime. Write a program that asks the user to enter an integer greater than 1, then displays all of the prime numbers that are less than or equal to the number entered The program should work as follows: Once the user has entered a number, the program should populate a list with all of the integers from 2 up through the value entered. The program should then use a loop to step through the list. The loop should pass each element to a function that displays the element whether it is a prime number.arrow_forwardCorrect code to output the following: 0 2 4 6 8 10 12 14 16 18 CODE: int[] numbers = new int[10]; for(int i=0; i < numbers.length; ++i) numbers[i] = i * 2; for(int i=0; i < numbers.length; i++) System.out.print(numbers[++i] + " "); System.out.println();arrow_forwardYour task is to take an integer number n & prints the difference between the product of its digits and the sum of its digits.arrow_forward
- n this lab, you complete a prewritten Python program for a carpenter who creates personalized house signs. The program is supposed to compute the price of any sign a customer orders, based on the following facts: The charge for all signs is a minimum of $35.00. The first five letters or numbers are included in the minimum charge; there is a $4 charge for each additional character. If the sign is make of oak, add $20.00. No charge is added for pine. Black or white characters are included in the minimum charge; there is an additional $15 charge for gold-leaf lettering.arrow_forwardIn Java: Forms often allow a user to enter an integer. Write a program that takes in a string representing an integer as input, and outputs yes if every character is a digit 0-9. Ex: If the input is: 1995 the output is: yes Ex: If the input is: 42,000 or 1995! the output is: no Hint: Use a loop and the Character.isDigit() function.arrow_forwardDescription A mathmatician Goldbach's conjecture: any even number(larger than 2) can divide into two prime number’s sum.But some even numbers can divide into many pairs of two prime numbers’ sum. Example:10 =3+7, 10=5+5, 10 can divide into two pairs of two prime number. Input Input consist a positive even number n(4<=n<=32766). Output Print the value of how many pairs are this even number can be divided into. Sample Input 1 1234 Sample Output 1 25arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
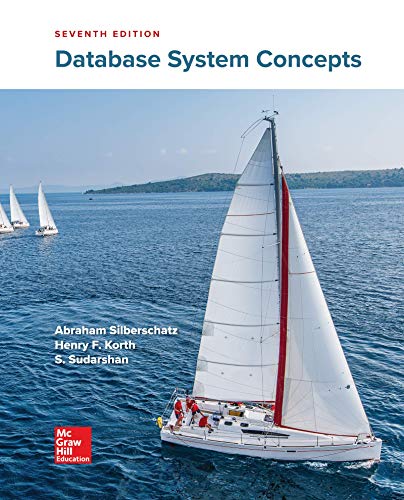
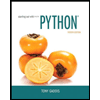
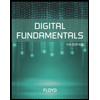
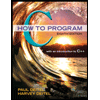
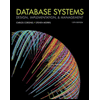
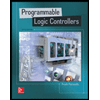