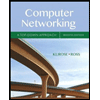
What is the probability that in a classroom of x people, at least 2 will be born on the same day of the year (ignore leap year)? Use a Monte Carlo Simulation and a frequency table to write a program that calculates this probability, where the number of people (x) in the simulated class is given by the user.
PLEASE USE the outline of the code GIVEN to answer this question:
import math
import random
# create and initialize frequency table:
ft = []
k = 0
while(k < 365) :
ft.append(0)
k = k+1
# Allow the user to determine class size:
print("Please type in how many people are in the class: ")
x= int(input())
success = 0
# Simulate:
c = 0
while(c < 10000) :
# Step 1: re-initialize birthday frequency table (it must be re-initialized for each play-through (why?):
k = 0
while(k < 365) :
ft[k] = 0
k = k+1
# Step 2: randomly get x birthdays and update frequency table:
k = 0
while(k < x):
# your code goes here ##########################
k = k+1
# Step 3: Check to see if this class has at least two people with same b-day and update success appropriately
k = 0
while(k < 365):
# your code goes here #########################
k = k+1
c = c+1
print("The probability that in a class of ", end="")
print(x , end="")
print(" people, at least two have the same birthday is: " , end="")
print(success/10000)
THANK YOU!

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 2 images

- A parking lot charges $3 per hour for the first 10 hours of a 24 hour period. There is no further charge starting at the 11th hour and ending at the 24th hour. The charge for a full 24 hour period is therefore $30. The same pattern applies for periods longer than 24 hours. Write a program to calculate the cost in dollars, given the number of hours that the parking lot was used. public class Parking { } public static void main(String[] args) { } // Change hours to get the answer for the those number of hours. int hours = 25; } int result = cost(hours); System.out.println(result); public static int cost(int hours) { int result = 0; // Write your code within the body of this method. return result;arrow_forwardA robot sits in a 30 foot square room. It be directed to travel a randomly generated distance between 8 and 20 feet. The user will input a value that will serve as the seed for the random number generator. Every foot it travels it indicates that it has traveled 'x feet'. Upon arrival at the user specified distance, it announces." made it!"(This will happen even if the robot moves 0 feet). The program should also indicate how many more feet the robot could travel before running into a wall assuming it always starts at one wall of the room. Note that grammar used to indicate singular versus plural distances. i.e. for an random generation of 5, the resulting output should be: How far should the robot travel? 5 feet 1 foot I made it! I can only travel 25 mor 2 feet 3 feet 4 feet 5 feet e feet. tabs are used to space the output of traveled feet 330492.1753326.qx3zay7 LAB АCTIVITY main.cpp 1 #include 2 using namespace std; 3 4 int main() int roomsize - 30; 6 int distance = 0; 7 8 cout <«…arrow_forwardANSWER MUST BE IN PYTHON3. This is the method header: #!/bin/python3 import math import os import random import re import sys # # Complete the 'linear_interpolate' function below. # # The function is expected to return a float. # The function accepts following parameters: # 1. int n # 2. List[float] x_knots # 3. List[float] y_knots # 4. float x_input # def linear_interpolate(n, x_knots, y_knots, x_input):arrow_forward
- Loop through the animalList created in a previous question and print the total time taken and total time given for each animal. Example output: Zirly the Zebra - Time taken for all behaviors: 890 minutes of 1000 Henry the Hawk - Time taken for all behaviors: 350 minues of 1000 Choose one of the following from the above choices (A, B, C or D within the code):// Assume the following variables exist: Behavior[] someBehaviors = // Omitted for brevity Animal zirly = Animal("Zirly the Zebra", 812.3, someBehaviors); Animal henry = Animal("Henry the Hawk", 35.5, someBehaviors); Animal[] animalList = new Animal[]{ zirly, henry }; // --------------------------- // A for(int i = animalList.length; i < animalList.length; i++) { Animal tempAnimal = animalList[i]; int timeTakenSum = 0; int timeGivenSum = 0; for(j = 0; j < tempAnimal.getBehaviorList().length; j++) { Behavior tempBehavior = tempAnimal.getBehaviorList()[j]; timeTakenSum +=…arrow_forward1. fInd the average win and lose rate of the crabs game 2. Find the Mean, Median, and mode of how long a player takes until he wins and loses a game.Basically find out how long each game lasts. With mean, median, and mode. This code is for python and its for the craps game. Below is the code I have so far. import randomimport sysimport pandas as pddef roll_dice():die1 = random.randrange(1, 7)die2 = random.randrange(1, 7)return (die1, die2)def display_dice(dice):die1, die2 = diceprint(f'Player rolled {die1} + {die2} = {sum(dice)}')# List that stores number of wins on every rollwinList = []# List that stores number of losses on every rolllossList = []# List that stores label indexes of horizontal bar plotylabel = []# 1# number of games of crapsn = int(input("Enter number of games: "))# Iterating 13 times# Because it is mentioned in the question that plot should have 13# horizontal bars for wins, and 13 horizontal bars for losses.for roll in range(13):ylabel.append('Roll ' + str(roll +…arrow_forwardMailings Review View Help AaBbC AaBbCcD AaBbCcD AaBbCc Aa Heading 1 T Normal 1No Spac. Heading 2 Hea Paragraph Styles 8. Consider the following equation for the sum of a finite arithmetic series: S, = n(a, ta where n is the number of elements in the series, a is the first element of the series, and a, is the last element of the series. Write a method that returns the sum of the elements stored in the array in 8 above. The array must be passed to the method as an argument. Your solution should implement the equation for the sum S.arrow_forward
- Given code: int s=0; for ( int i=1; i<5; i++ ){ s = s + i ; } Which one is correct, after running the code. Group of answer choices s=1 s=15 s=10 s=5arrow_forwardHow do you make a username password generator in pythonarrow_forwardJava Programming: Write a program that reads five integer values from the user, then analyzes them as if they were a hand of cards. When your program runs it might look like this (user input is in orange for clarity): Enter five numeric cards, no face cards. Use 2 - 9. Card 1: 8 Card 2: 7 Card 3: 8 Card 4: 2 Card 5: 9 Pair! (This is a pair, since there are two eights). You are only required to find the hands Pair and Straight.arrow_forward
- In PYTHON use a Monte Carlo Simulation to write a code that gives the probability that in a classroom of x people, at least 2 will be born on the same day of the year, ignoring leap year? The number of people in the class is given by the user as variable x. Here is the code outline that is needed to answer this question. Please use it in your answer: import mathimport random # create and initialize frequency table:ft = []k = 0while(k < 365) : ft.append(0) k = k+1 # Allow the user to determine class size:print("Please type in how many people are in the class: ")x= int(input()) success = 0 # Simulate:c = 0while(c < 10000) : # Step 1: re-initialize birthday frequency table (it must be re-initialized for each play-through (why?): k = 0 while(k < 365) : ft[k] = 0 k = k+1 # Step 2: randomly get x birthdays and update frequency table: k = 0 while(k < x): # your code goes here ########################## k = k+1 # Step 3: Check to see if this…arrow_forwardstrobogrammatic number is a number that looks the same when rotated 180 degrees (looked at upside down). Find all strobogrammatic numbers that are of length = n. For example, Given n = 2, return ["11","69","88","96"]. def gen_strobogrammatic(n): Given n, generate all strobogrammatic numbers of length n. :type n: int :rtype: List[str] return helper(n, n) def helper(n, length): if n == 0: return [™"] if n == 1: return ["1", "0", "8"] middles = helper(n-2, length) result = [] for middle in middles: if n != length: result.append("0" + middle + "0") result.append("8" + middle + "8") result.append("1" + middle + "1") result.append("9" + middle + "6") result.append("6" + middle + "9") return result def strobogrammatic_in_range(low, high): :type low: str :type high: str :rtype: int res = count = 0 low_len = len(low) high_len = len(high) for i in range(low_len, high_len + 1): res.extend(helper2(i, i)) for perm in res: if len(perm) == low_len and int(perm) int(high): continue count += 1 return…arrow_forwardthis is a java exercisearrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
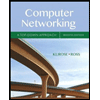
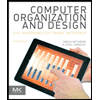
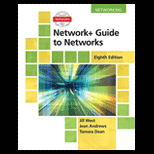
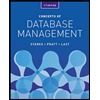
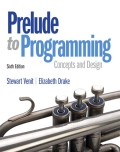
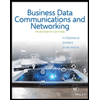