shuffle(cards) : Shuffles the 52 cards in a new list, such that the list of cards is ordered randomly, while leaving the original list of 52 cards unchanged. Shuffle returns the "shuffled deck" to the caller. Input parameters: a list of cards, not shuffled. Return value: a list of cards, shuffled. The original list is not modified (aka "mutated") deal(number_of_hands, number_of_cards, cards): Takes the number of hands (up to 4), number of cards per hand (up to 13), and the deck of cards to deal from. Your function should return a list containing all of the hands that were dealt. Each hand will be a represented as a list of cards. Therefore, this function will return a list of lists. Input parameters: number of hands -> int, number of cards -> int, cards -> list. Return value: a list of hands, where each hand is itself a list of cards dealt.
shuffle(cards) : Shuffles the 52 cards in a new list, such that the list of cards is ordered randomly, while leaving the original list of 52 cards unchanged. Shuffle returns the "shuffled deck" to the caller. Input parameters: a list of cards, not shuffled. Return value: a list of cards, shuffled. The original list is not modified (aka "mutated") deal(number_of_hands, number_of_cards, cards): Takes the number of hands (up to 4), number of cards per hand (up to 13), and the deck of cards to deal from. Your function should return a list containing all of the hands that were dealt. Each hand will be a represented as a list of cards. Therefore, this function will return a list of lists. Input parameters: number of hands -> int, number of cards -> int, cards -> list. Return value: a list of hands, where each hand is itself a list of cards dealt.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
100%
this is a python deck of card question, please help with the questions thank you!!
heres my code:
import random
def card_draw():
suits = ["s", "h", "d", "c" ]
faces = ["2","3","4","5","6","7","8","9","T","J","Q","K","A"]
deck = [ ]
for suit in suits:
for face in faces:
deck.append((face+suit[0]))
return deck
def shuffled_deck( ):
#card_draw()
new = [ ]
# n = random.shuffle(card_draw())
new.append(random.shuffle(card_draw()))
return new
def main():
print("Original deck is:")
print(card_draw())
print("The shuffled deck is:")
print(shuffled_deck)
if __name__ == "__main__":
main()
![shuffle(cards): Shuffles the 52 cards in a new list, such that the list of cards is ordered randomly, while
leaving the original list of 52 cards unchanged. Shuffle returns the "shuffled deck" to the caller.
Input parameters: a list of cards, not shuffled. Return value: a list of cards, shuffled. The original list is
not modified (aka "mutated")
deal(number_of_hands, number_of_cards, cards): Takes the number of hands (up to 4), number of cards per
hand (up to 13), and the deck of cards to deal from. Your function should return a list containing all of the
hands that were dealt. Each hand will be a represented as a list of cards. Therefore, this function will
return a list of lists.
Input parameters: number of hands -> int, number of cards -> int, cards -> list.
Return value: a list of hands, where each hand is itself a list of cards dealt.
Example tests we might run with your functions:
= RESTART: /Users/keithbagley/Dropbox/NORTHEASTERN UNIVERSITY/CSS001/Fal12020/Home
work/HN4/cards.py
Original deck is:
['2s', '3s', '4s', '5s', '6s', '7s', '8s', '9s', 'Ts', 'Js', 'Qs', 'Ks', 'As', '2h
', '3h', '4h', '5h', '6h', '7h', '8h', '9h', 'Th', 'Jh', 'Qh', 'Kh', 'Ah', '2d',
3d', '4d', '5d', '6d', '7d', '8d', '9d', 'Td', 'Jd', 'Qd', 'Kd', 'Ad', '2c', '3c',
"4c', '5c', '6c', '7c', '8c', '9c', 'Tc', 'Jc', 'Qc', 'Kc', 'Ac']
The shuffled deck is:
['7c', '3s', '4s', '5s', '6s', '7s', '8s', '9s', 'Ts', 'Js', 'Qs', 'Ks', 'As', '2h
', '3h', '4h', '5h', '6h', '7h', '8h', '9h', 'Th', 'Jh', 'Qh', 'Kh', 'Ah', '2d', '
3d', '4d', '5d', '6d', '7d', '8d', '9d', 'Td', 'Jd', 'Qd', "Kd', 'Ad', '2c', '3c',
'4c', '5c', '6c', '2s', '8c', '9c', 'Tc', 'Jc', 'Qc', 'Kc', 'Ac']
Dealt one hand
[['7c', '6s'], ['3s', '7s'], ['4s', '8s'], ['5s', '9s']]
Cards left in deck
['Ts', 'Js', 'Qs', 'Ks', 'As', '2h', '3h', '4h', '5h', '6h', '7h', '8h', '9h', 'Th
', 'Jh', 'Qh', 'Kh', 'Ah', '2d', '3d', '4d', '5d', '6d', '7d', '8d', '9d', 'Td', '
Jd', 'Qd', 'Kd', 'Ad', '2c', '3c', '4c', '5c', '6c', '2s', '8c', '9c', 'Tc', 'Jc',
"Qc', 'Kc', 'Ac']](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F10732faa-f9b7-4c12-81f1-19b5d255f419%2F2897402d-3b98-417a-b49f-0d52750d9af8%2Fk8df1pm_processed.png&w=3840&q=75)
Transcribed Image Text:shuffle(cards): Shuffles the 52 cards in a new list, such that the list of cards is ordered randomly, while
leaving the original list of 52 cards unchanged. Shuffle returns the "shuffled deck" to the caller.
Input parameters: a list of cards, not shuffled. Return value: a list of cards, shuffled. The original list is
not modified (aka "mutated")
deal(number_of_hands, number_of_cards, cards): Takes the number of hands (up to 4), number of cards per
hand (up to 13), and the deck of cards to deal from. Your function should return a list containing all of the
hands that were dealt. Each hand will be a represented as a list of cards. Therefore, this function will
return a list of lists.
Input parameters: number of hands -> int, number of cards -> int, cards -> list.
Return value: a list of hands, where each hand is itself a list of cards dealt.
Example tests we might run with your functions:
= RESTART: /Users/keithbagley/Dropbox/NORTHEASTERN UNIVERSITY/CSS001/Fal12020/Home
work/HN4/cards.py
Original deck is:
['2s', '3s', '4s', '5s', '6s', '7s', '8s', '9s', 'Ts', 'Js', 'Qs', 'Ks', 'As', '2h
', '3h', '4h', '5h', '6h', '7h', '8h', '9h', 'Th', 'Jh', 'Qh', 'Kh', 'Ah', '2d',
3d', '4d', '5d', '6d', '7d', '8d', '9d', 'Td', 'Jd', 'Qd', 'Kd', 'Ad', '2c', '3c',
"4c', '5c', '6c', '7c', '8c', '9c', 'Tc', 'Jc', 'Qc', 'Kc', 'Ac']
The shuffled deck is:
['7c', '3s', '4s', '5s', '6s', '7s', '8s', '9s', 'Ts', 'Js', 'Qs', 'Ks', 'As', '2h
', '3h', '4h', '5h', '6h', '7h', '8h', '9h', 'Th', 'Jh', 'Qh', 'Kh', 'Ah', '2d', '
3d', '4d', '5d', '6d', '7d', '8d', '9d', 'Td', 'Jd', 'Qd', "Kd', 'Ad', '2c', '3c',
'4c', '5c', '6c', '2s', '8c', '9c', 'Tc', 'Jc', 'Qc', 'Kc', 'Ac']
Dealt one hand
[['7c', '6s'], ['3s', '7s'], ['4s', '8s'], ['5s', '9s']]
Cards left in deck
['Ts', 'Js', 'Qs', 'Ks', 'As', '2h', '3h', '4h', '5h', '6h', '7h', '8h', '9h', 'Th
', 'Jh', 'Qh', 'Kh', 'Ah', '2d', '3d', '4d', '5d', '6d', '7d', '8d', '9d', 'Td', '
Jd', 'Qd', 'Kd', 'Ad', '2c', '3c', '4c', '5c', '6c', '2s', '8c', '9c', 'Tc', 'Jc',
"Qc', 'Kc', 'Ac']
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
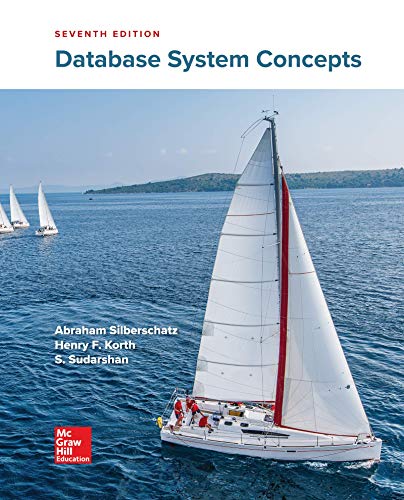
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
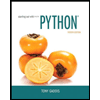
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
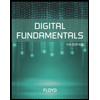
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
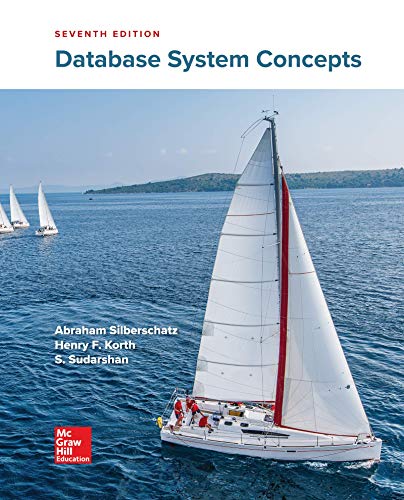
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
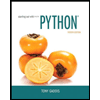
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
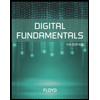
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
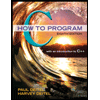
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
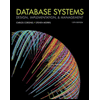
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
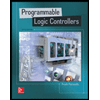
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education