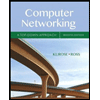
What does a pseudocode for the following
class PersonalInformation:
def __init__(self, name, address, age, phone):
self.name = name
self.address = address
self.age = age
self.phone = phone
def getName(self):
return self.name
def getAddress(self):
return self.address
def getAge(self):
return self.age
def getPhone(self):
return self.phone
def setName(self, name):
self.name = name
def setAddress(self, address):
self.address = address
def setAge(self, age):
self.age = age
def setPhone(self, phone):
self.phone = phone
def __str__(self): return self.name +" " + self.address + " " + str(self.age) +" "+ str(self.phone)
def main():
person1 = PersonalInformation("Ronaldo","Address1",40,1234567890)
person2 = PersonalInformation("Messi", "Address2", 41, 6789012345)
print(person1)
print(person2)
main()

Step by stepSolved in 2 steps

- #this is a python program #topic: OOP Design the Country class so that the code gives the expected output. [You are not allowed to change the code below] # Write your Class Code here country = Country() print('Name:',country.name) print('Continent:',country.continent) print('Capital:',country.capital) print('Fifa Ranking:',country.fifa_ranking) print('===================') country.name = “Belgium” country.continent = “Europe” country.capital = “Brussels” country.fifa_ranking = 1 print('Name:',country.name) print('Continent:',country.continent) print('Capital:',country.capital) print('Fifa Ranking:',country.fifa_ranking) Output: Name: Bangladesh Continent: Asia Capital: Dhaka Fifa Ranking: 187 =================== Name: Belgium Continent: Europe Capital: Brussels Fifa Ranking: 1arrow_forwardclass Student: def __init__(self, id, fn, ln, dob, m='undefined'): self.id = id self.firstName = fn self.lastName = ln self.dateOfBirth = dob self.Major = m def set_id(self, newid): #This is known as setter self.id = newid def get_id(self): #This is known as a getter return self.id def set_fn(self, newfirstName): self.fn = newfirstName def get_fn(self): return self.fn def set_ln(self, newlastName): self.ln = newlastName def get_ln(self): return self.ln def set_dob(self, newdob): self.dob = newdob def get_dob(self): return self.dob def set_m(self, newMajor): self.m = newMajor def get_m(self): return self.m def print_student_info(self): print(f'{self.id} {self.firstName} {self.lastName} {self.dateOfBirth} {self.Major}')all_students = []id=100user_input = int(input("How many students: "))for x in range(user_input): firstName = input('Enter…arrow_forwardC# Solve this error using System; namespace RahmanA3P1BasePlusCEmployee { public class BasePlusCommissionEmployee { public string FirstName { get; } public string LastName { get; } public string SocialSecurityNumber { get; } private decimal grossSales; // gross weekly sales private decimal commissionRate; // commission percentage private decimal baseSalary; // base salary per week // six-parameter constructor public BasePlusCommissionEmployee(string firstName, string lastName, string socialSecurityNumber, decimal grossSales, decimal commissionRate, decimal baseSalary) { // implicit call to object constructor occurs here FirstName = firstName; LastName = lastName; SocialSecurityNumber = socialSecurityNumber; GrossSales = grossSales; // validates gross sales CommissionRate = commissionRate; // validates commission rate BaseSalary = baseSalary; // validates base…arrow_forward
- python: class Student:def __init__(self, first, last, gpa):self.first = first # first nameself.last = last # last nameself.gpa = gpa # grade point average def get_gpa(self):return self.gpa def get_last(self):return self.last class Course:def __init__(self):self.roster = [] # list of Student objects def add_student(self, student):self.roster.append(student) def course_size(self):return len(self.roster) # Type your code here if __name__ == "__main__":course = Course()course.add_student(Student('Henry', 'Nguyen', 3.5))course.add_student(Student('Brenda', 'Stern', 2.0))course.add_student(Student('Lynda', 'Robison', 3.2))course.add_student(Student('Sonya', 'King', 3.9)) student = course.find_student_highest_gpa()print('Top student:', student.first, student.last, '( GPA:', student.gpa,')')arrow_forwardProgram - Python This is my program for a horse race class (Problem below code) class Race: def __init__(self,name,time): self.name = name self.time = time self.entries = [] class Entrant: def __init__(self,horsename,jockeyname): self.horsename = horsename self.jockeyname = jockeyname race1 = Race('RACE 1','10:00 AM May 12, 2021')race2 = Race('RACE 2','11:00 AM May 12, 2021')race3 = Race('RACE 3','12:00 PM May 12, 2021') race1.entries.append(Entrant('Misty Spirit','John Valazquez'))race1.entries.append(Entrant('Frankly I’m Kidding','Mike E Smith')) race2.entries.append(Entrant('Rage against the Machine','Russell Baze')) race3.entries.append(Entrant('Secretariat','Bill Shoemaker'))race3.entries.append(Entrant('Man o War','David A Gall'))race3.entries.append(Entrant('Seabiscuit','Angel Cordero Jr')) print(race1.name, race1.time)for entry in race1.entries: print('Horse:',entry.horsename) print('Jockey:',entry.jockeyname) print()…arrow_forwardPython Code please Point of Sale Write a program that will manage the point of sale in a store. Build the ItemToPurchase class with the following: Attributes item_name (string) item_price (int) item_quantity (int) Default constructor Initializes item's name = "none", item's price = 0, item's quantity = 0 Method print_item_cost() Example of print_item_cost() output:Bottled Water 10 @ $1 = $10 Extend the ItemToPurchase class to contain a new attribute. item_description (string) - Set to "none" in default constructor Implement the following method for the ItemToPurchase class. print_item_description() - Prints item_description attribute for an ItemToPurchase object. Has an ItemToPurchase parameter. Example of print_item_description() output:Bottled Water: Deer Park, 12 oz. Build the ShoppingCart class with the following data attributes and related methods. Note: Some can be method stubs (empty methods) initially, to be completed in later steps. Parameterized constructor…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
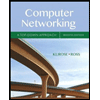
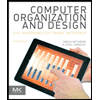
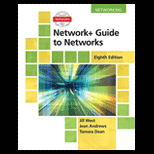
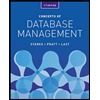
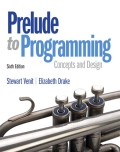
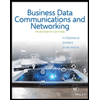