WEIGHT_LIMIT = 100 COUNT_LIMIT = 4 wizard_inventory = [] def display_title(): print("The Wizard Inventory program") print() def display_menu(): print("COMMAND MENU") print("show - Show all items") print("grab - Grab an item") print("edit - Edit an item") print("drop - Drop an item") print("exit - Exit program") print() def show(inventory): print("Show inventory") for i in inventory: print(i[0], i[1], "(", i[2], ")lbs") total = calculate_weight(inventory) print("Total Weights is : ", total, "lbs") print(" Weight limits of 100 lbs") def calculate_weight(inventory): total = 0 for i in inventory: print(i[2]) total = total + float(i[2]) return total def grab_item(inventory): list = [] total = 0 if (len(inventory) < 4): list.extend(get_new_item()) print(list) total = calculate_weight(inventory) total = total + float(list[2]) print(total) if (total < 100): inventory.append(list) else: print("weight exceeds limit of 100 lbs") show(inventory) # create a function which adds new items def get_new_item(): New_Items = [] # store item in list for i in range(3): New_Items.append(input(f'Enter value at index {i}: ')) return New_Items def edit_item(inventory): index = int(input("Enter the index of the item to de edited: ")) if (index > len(inventory)): print("Wrong index,Try Again!") else: Newname = input("Enter the new name for the item: ") print("Before edit : ", inventory[index]) inventory[index][0] = Newname print("After edit : ", inventory[index]) def drop_item(inventory): index = int(input("Enter the index of the item to de deleted: ")) if (index > len(inventory)): print("Wrong index,Try Again!") else: show(inventory) inventory.pop(index) show(inventory) def main(): display_title() display_menu() # start with these 3 items wizard_inventory = [["wooden staff", "Brown", 30.0], ["wizard hat", "Black", 1.5], ["cloth shoes", "Blue", 5.3]] while True: command = input("Command: ") print(command) if command == "show": show(wizard_inventory) elif command == "grab": grab_item(wizard_inventory) elif command == "edit": edit_item(wizard_inventory) elif command == "drop": drop_item(wizard_inventory) elif command == "exit": break else: print("Not a valid command. Please try again.\n") print("Bye!") if __name__ == "__main__": main()
I don't need the get_new_item and the color of the items but it breaks the code, how do I fix this?
WEIGHT_LIMIT = 100
COUNT_LIMIT = 4
wizard_inventory = []
def display_title():
print("The Wizard Inventory
print()
def display_menu():
print("COMMAND MENU")
print("show - Show all items")
print("grab - Grab an item")
print("edit - Edit an item")
print("drop - Drop an item")
print("exit - Exit program")
print()
def show(inventory):
print("Show inventory")
for i in inventory:
print(i[0], i[1], "(", i[2], ")lbs")
total = calculate_weight(inventory)
print("Total Weights is : ", total, "lbs")
print(" Weight limits of 100 lbs")
def calculate_weight(inventory):
total = 0
for i in inventory:
print(i[2])
total = total + float(i[2])
return total
def grab_item(inventory):
list = []
total = 0
if (len(inventory) < 4):
list.extend(get_new_item())
print(list)
total = calculate_weight(inventory)
total = total + float(list[2])
print(total)
if (total < 100):
inventory.append(list)
else:
print("weight exceeds limit of 100 lbs")
show(inventory)
# create a function which adds new items
def get_new_item():
New_Items = []
# store item in list
for i in range(3):
New_Items.append(input(f'Enter value at index {i}: '))
return New_Items
def edit_item(inventory):
index = int(input("Enter the index of the item to de edited: "))
if (index > len(inventory)):
print("Wrong index,Try Again!")
else:
Newname = input("Enter the new name for the item: ")
print("Before edit : ", inventory[index])
inventory[index][0] = Newname
print("After edit : ", inventory[index])
def drop_item(inventory):
index = int(input("Enter the index of the item to de deleted: "))
if (index > len(inventory)):
print("Wrong index,Try Again!")
else:
show(inventory)
inventory.pop(index)
show(inventory)
def main():
display_title()
display_menu()
# start with these 3 items
wizard_inventory = [["wooden staff", "Brown", 30.0], ["wizard hat", "Black", 1.5], ["cloth shoes", "Blue", 5.3]]
while True:
command = input("Command: ")
print(command)
if command == "show":
show(wizard_inventory)
elif command == "grab":
grab_item(wizard_inventory)
elif command == "edit":
edit_item(wizard_inventory)
elif command == "drop":
drop_item(wizard_inventory)
elif command == "exit":
break
else:
print("Not a valid command. Please try again.\n")
print("Bye!")
if __name__ == "__main__":
main()

Step by step
Solved in 3 steps with 2 images

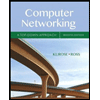
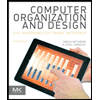
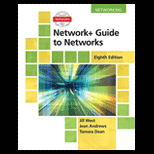
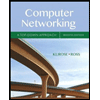
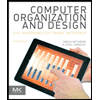
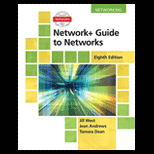
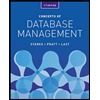
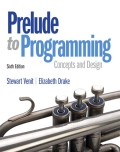
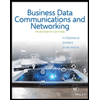