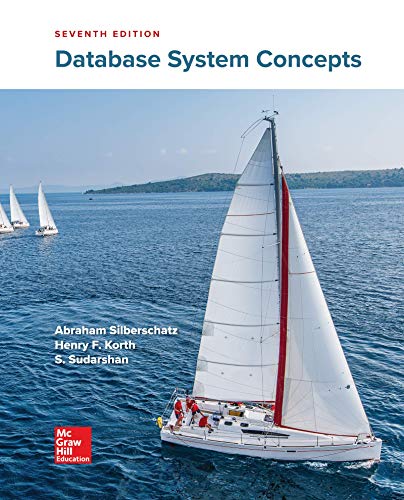
please go in to the detail and explain the purpose and function to each line & function of code listed below.
#include <stdio.h>
//Represent a node of the singly linked list
struct node{
int data;
struct node *next;
};
//Represent the head and tail of the singly linked list
struct node *head, *tail = NULL;
//addNode() will add a new node to the list
void addNode(int data) {
//Create a new node
struct node *newNode = (struct node*)malloc(sizeof(struct node));
newNode->data = data;
newNode->next = NULL;
//Checks if the list is empty
if(head == NULL) {
//If list is empty, both head and tail will point to new node
head = newNode;
tail = newNode;
}
else {
//newNode will be added after tail such that tail's next will point to newNode
tail->next = newNode;
//newNode will become new tail of the list
tail = newNode;
}
}
//removeDuplicate() will remove duplicate nodes from the list
void removeDuplicate() {
//Node current will point to head
struct node *current = head, *index = NULL, *temp = NULL;
if(head == NULL) {
return;
}
else {
while(current != NULL){
//Node temp will point to previous node to index.
temp = current;
//Index will point to node next to current
index = current->next;
while(index != NULL) {
//If current node's data is equal to index node's data
if(current->data == index->data) {
//Here, index node is pointing to the node which is duplicate of current node
//Skips the duplicate node by pointing to next node
temp->next = index->next;
}
else {
//Temp will point to previous node of index.
temp = index;
}
index = index->next;
}
current = current->next;
}
}
}
//display() will display all the nodes present in the list
void display() {
//Node current will point to head
struct node *current = head;
if(head == NULL) {
printf("List is empty \n");
return;
}
while(current != NULL) {
//Prints each node by incrementing pointer
printf("%d ", current->data);
current = current->next;
}
printf("\n");
}
int main()
{
//Adds data to the list
addNode(1);
addNode(2);
addNode(3);
addNode(2);
addNode(2);
addNode(4);
addNode(1);
printf("Originals list: \n");
display();
//Removes duplicate nodes
removeDuplicate();
printf("List after removing duplicates: \n");
display();
return 0;
}

Step by stepSolved in 2 steps with 1 images

- in c++arrow_forwardC++The List class represents a linked list of dynamically allocated elements. The list has only one member variable head which is a pointer that leads to the first element. See the following code for the copy constructor to List. List (const List & list) { for (int i = 0; i <list.size (); i ++) { push_back (list [i]); } } What problems does the copy constructor have? Select one or more options: 1. List (const List & list) {} does not define a copy constructor. 2. The list parameter should not be constant. 3. The new elements will be added to the wrong list. 4. Copy becomes shallow rather than deep. 5. The copy will create dangling pointers. 6. Copying will create memory leaks. 7. The condition must be: i <size () 8. head is never initialized.arrow_forwardstruct insert_at_back_of_sll { // Function takes a constant Book as a parameter, inserts that book at the // back of a singly linked list, and returns nothing. void operator()(const Book& book) { /// TO-DO (3) /// // Write the lines of code to insert "book" at the back of "my_sll". Since // the SLL has no size() function and no tail pointer, you must walk the // list looking for the last node. // // HINT: Do not attempt to insert after "my_sll.end()". // ///// END-T0-DO (3) ||||// } std::forward_list& my_sll; };arrow_forward
- Create a user-defined function called duplicates. This function will check to see if a singly linked list contains nodes with the same data stored. If any duplicates are found, return the value 1. Otherwise return 0. You may use the following typedef structure. typedef struct node_s{ int data; struct node_s * nextptr; }node_t;arrow_forwardSuppose a node of a doubly linked list is defined as follows: struct Node{ int data; struct Node* next; struct Node* prev; }; Write the function definition of the function deleteElement as presented below. This function deletes a node at position n from a doubly linked list. struct Node* deleteElement(struct Node* head, int n){ //write the function definition }arrow_forwardPlease fill in the blanks from 26 to 78 in C. /*This program will print students’ information and remove students using linked list*/ #include<stdio.h> #include<stdlib.h> //Declare a struct student (node) with 5 members //each student node contains an ID, an age, graduation year, // 1 pointer points to the next student, and 1 pointer points to the previous student. struct student { int ID; int age; int classOf; struct student* next; struct student* prev; }; /* - This function takes the head pointer and iterates through the list to get all 3 integers needed from the user, and save it to our list - Update the pointer to point to the next node.*/ void scanLL(struct student* head) { while(head != NULL) //this condition makes sure the list is not at the end { printf("Lets fill in the information. Enter 3 integers for the student's ID, age, and graduation year: "); scanf("%i %i %i",&&head->ID, &head->age, &head->classOf); //in order: ID, age, graduation…arrow_forward
- • find_last(my_list, x): Takes two inputs: the first being a list and the second being any type. Returns the index of the last element of the list which is equal to the second input; if it cannot be found, returns None instead. >> find_last(['a', 'b', 'b', 'a'], 'b') 2 >>> ind = find_last(['a', 'b', 'b', 'a'], 'c') >>> print(ind) Nonearrow_forwardplease complate code in fill in the blanksarrow_forwardProject 2: Singly-Linked List The purpose of this assignment is to assess your ability to: ▪Implement sequential search algorithms for linked list structures ▪Implement sequential abstract data types using linked data ▪Analyze and compare algorithms for efficiency using Big-O notation For this assignment, you will implement a singly-linked node class. Use your singly-linked node to implement a singly-linked list class that maintains its elements in ascending order. The SinglyLinkedList class is defined by the following data: ▪A node pointer to the front and the tail of the list Implement the following methods in your class: ▪A default constructor list<T> myList ▪A copy constructor list<T> myList(aList) ▪Access to first elementmyList.front() ▪Access to last elementmyList.back() ▪Insert value myList.insert(val) ▪Remove value at frontmyList.pop_front() ▪Remove value at tailmyList.pop_back() ▪Determine if emptymyList.empty() ▪Return # of elementsmyList.size() ▪Reverse order of…arrow_forward
- LAB: Playlist (output linked list) Given main(), complete the SongNode class to include the function PrintSongInfo(). Then write the PrintPlaylist() function in main.cpp to print all songs in the playlist. DO NOT print the head node, which does not contain user-input values. Ex: If the input is: Stomp! 380 The Brothers Johnson The Dude 337 Quincy Jones You Don't Own Me 151 Lesley Gore -1 the output is: LIST OF SONGS ------------- Title: Stomp! Length: 380 Artist: The Brothers Johnson Title: The Dude Length: 337 Artist: Quincy Jones Title: You Don't Own Me Length: 151 Artist: Lesley Gorearrow_forwardstruct node{int num;node *next, *before;};start 18 27 36 45 54 63 The above-linked list is made of nodes of the type struct ex. Your task is now to Write a complete function code to a. Find the sum of all the values of the node in the linked list. b. Print the values in the linked list in reverse order. Use a temporary pointer temp for a and b. i dont need a full code just the list partarrow_forwardO b. Remove the O. Remove the second element in the list O d. Remove the element before the last element in the list QUESTION 6 The following code inserts an element at the beginning of the list public void insertBegin(T e) { Node temp = new Node (e); head = temp; temp.Next = head; size++; } O True O False QUESTION 7 what does the following code do?arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
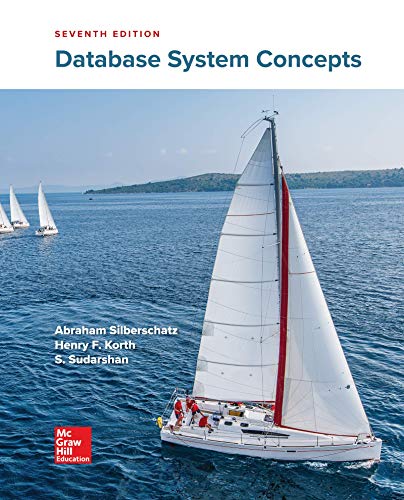
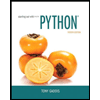
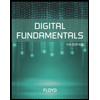
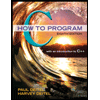
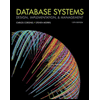
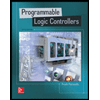