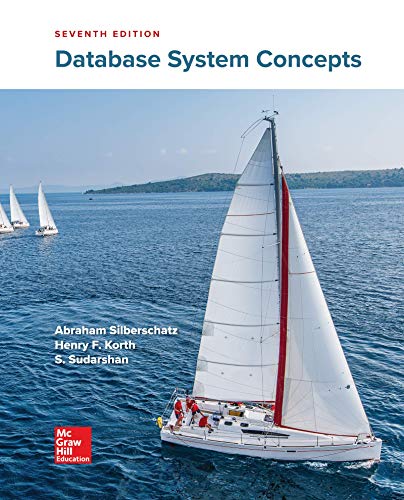
USING PYTHON
1. Write the PYTHON Programs for the following exercises
2. For each program, you need to write:
-
- Comments for all the values, constants, and functions
- IPO
- Variables
- Pseudocode
Patient Charges
Write a class named Patient that has attributes for the following data:
- First name, middle name, and last name
- Address, city, state, and ZIP code
- Phone number
- Name and phone number of emergency contact
The Patient class’s _ _init_ _ method should accept an argument for each attribute. The Patient class should also have accessor and mutator methods for each attribute.
Next, write a class named Procedure that represents a medical procedure that has been performed on a patient. The Procedure class should have attributes for the following data:
- Name of the procedure
- Date of the procedure
- Name of the practitioner who performed the procedure
- Charges for the procedure
The Procedure class’s _ _init_ _ method should accept an argument for each attribute. The Procedure class should also have accessor and mutator methods for each attribute.
Next, write a program that creates an instance of the Patient class, initialized with sample data.
Then, create three instances of the Procedure class, initialized with the following data:
Procedure #1: Procedure #2: Procedure #3:
Procedure name: Physical ExamProcedure name: X-ray Procedure name: Blood test
Date: Today’s date Date: Today’s date Date: Today’s date
Practitioner: Dr. Irvine Practitioner: Dr. Jamison Practitioner: Dr. Smith
Charge: 250.00 Charge: 500.00 Charge: 200.00
The program should display
the patient’s information,
information about all three of the procedures,
and the total charges of the three procedures.

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 4 images

- Pythonarrow_forward#this is a python program #topic: OOP Design the Country class so that the code gives the expected output. [You are not allowed to change the code below] # Write your Class Code here country = Country() print('Name:',country.name) print('Continent:',country.continent) print('Capital:',country.capital) print('Fifa Ranking:',country.fifa_ranking) print('===================') country.name = “Belgium” country.continent = “Europe” country.capital = “Brussels” country.fifa_ranking = 1 print('Name:',country.name) print('Continent:',country.continent) print('Capital:',country.capital) print('Fifa Ranking:',country.fifa_ranking) Output: Name: Bangladesh Continent: Asia Capital: Dhaka Fifa Ranking: 187 =================== Name: Belgium Continent: Europe Capital: Brussels Fifa Ranking: 1arrow_forwardQuestion p .Full explain this question and text typing work only We should answer our question within 2 hours takes more time then we will reduce Rating Dont ignore this linearrow_forward
- true of false: a) The first step in any programming task is to start coding. b) The only places we would ever want to put a comment in our code are above a method and above a class.arrow_forwardTime This class is going to represent a 24-hour cycle clock time. That means 0:0:0 (Hour:Minute:Second) is the beginning of a day and 23:59:59 is the end of the day. This class has these data members. int sec int min int hourarrow_forwardCharge Account ValidationCreate a class with a method that accepts a charge account number as its argument. The method should determine whether the number is valid by comparing it to the following list of valid charge account numbers:5658845 4520125 7895122 8777541 8451277 13028508080152 4562555 5552012 5050552 7825877 12502551005231 6545231 3852085 7576651 7881200 4581002These numbers should be stored in an array. Use a sequential search to locate the number passed as an argument. If the number is in the array, the method should return true, indicating the number is valid. If the number is not in the array, the method should return false, indicating the number is invalid.Write a program that tests the class by asking the user to enter a charge account number. The program should display a message indicating whether the number is valid or invalid.arrow_forward
- 6. Patient Charges Write a class named Patient that has attributes for the following data: • First name, middle name, and last name • Address, city, state, and ZIP code • Phone number ● The Patient class's init__ method should accept an argument for each attribute. The Name and phone number of emergency contact Patient class should also have accessor and mutator methods for each attribute. Next, write a class named Procedure that represents a medical procedure that has been performed on a patient. The Procedure class should have attributes for the following data: • Name of the procedure • Date of the procedure • Name of the practitioner who performed the procedure • Charges for the procedure 107 The Procedure class's __init__ method should accept an argument for each attribute. The Procedure class should also have accessor and mutator methods for each attribute Next, write a program that creates an instance of the Patient class, initialized with sample data. Then, create three…arrow_forwardFocus on classes, objects, methods and good programming styleYour task is to create a BankAccount class. Class name BankAccount Attributes __balance float float __pin integer integer Methods __init_()get_pin()check_pin()deposit()withdraw()get_balance() The bank account will be protected by a 4-digit pin number (i.e. between 1000 and 9999). The pin should be generated randomly when the account object is created. The initial balance should be 0.get_pin()should return the pin.check_pin(pin) should check the argument against the saved pin and return True if it matches, False if it does not.deposit(amount) should receive the amount as the argument, add the amount to the account and return the new balance.withraw(amount) should check if the amount can be withdrawn (not more than is in the account), If so, remove the argument amount from the account and return the new balance if the transaction was successful. Return False if it was not.get_balance()…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
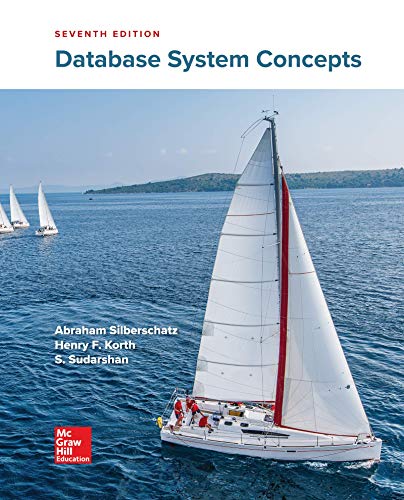
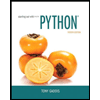
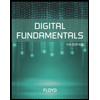
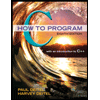
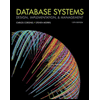
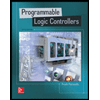