a. Define a Deque interface.
b. Define a LinkedDeque class (Node class)..
c. Define a Test class to test all methods
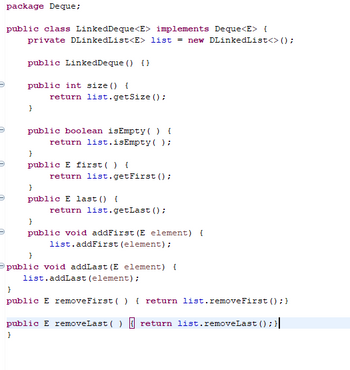
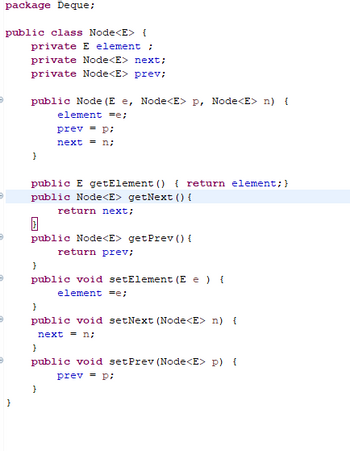

The code you provided looks like a complete and correct implementation of a Deque using a doubly linked list in Java. Here's the breakdown of the provided code:
The
Deque
interface defines the methods for a double-ended queue.The
Node
class represents nodes in the doubly linked list. Each node has an element, a reference to the previous node (prev
), and a reference to the next node (next
).The
LinkedDeque
class implements theDeque
interface using a doubly linked list. It has ahead
, atail
, and asize
to maintain the state of the deque. The methods are implemented as follows:addFirst
andaddLast
add elements to the front and back of the deque, respectively.removeFirst
andremoveLast
remove elements from the front and back of the deque, respectively.isEmpty
checks if the deque is empty.first
andlast
return the elements at the front and back of the deque, respectively.
The
Main
class is used to test the functionality of theLinkedDeque
class. It creates aLinkedDeque
of integers, adds elements, and removes elements to demonstrate how the deque works.
Step by stepSolved in 5 steps with 2 images

- Implement the Linked List using head and tail pointer. Interface (.h file) of LinkedList class is given below. Your task is to provide implementation (.cpp file) for LinkedList class. Interface: template<class Type> class LinkedList { private: Node<Type>* head; Node<Type>* tail; <Type> data; public: LinkedList<Type>(); LinkedList<Type>(const LinkedList<Type> &obj); void sortedInsert(Type); Type deleteFromPosition(int position); //deletes the node at the particular position Type delete(int start,int end); //deletes the nodes from starting to ending position bool isEmpty()const; void print()const; ~LinkedList(); }; program in c++.arrow_forwardUse a SinglyLinked List to implement a Queuea. Define a Queue interface.b. Define a LinkedQueue class (Node class)..c. Define a Test class to test all methods help me make a test class first code package LinkedQueue; import linkedstack.Node; public class SLinkedList<E> { private Node <E> head = null; private Node <E> tail = null; private int size = 0; public SLinkedList() { head = null; tail = null; size = 0; } public int getSize() { return size; } public boolean isEmpty() { return size == 0; } public E getFirst() { if(isEmpty()) { return null; } return head.getElement(); } public E getLast() { if(isEmpty()) { return null; } return tail.getElement(); } public void addFirst(E e) { Node<E> newest = new Node<>(e, null); newest.setNext(head); head = newest; if(getSize()==0) { tail = head; } size++; } public void addLast(E e) { Node<E> newest = new Node<>(e, null); if(isEmpty()) { head = newest; } else {…arrow_forwardJAVA please Given main() in the ShoppingList class, define an insertAtEnd() method in the ItemNode class that adds an element to the end of a linked list. DO NOT print the dummy head node. Ex. if the input is: 4 Kale Lettuce Carrots Peanuts where 4 is the number of items to be inserted; Kale, Lettuce, Carrots, Peanuts are the names of the items to be added at the end of the list. The output is: Kale Lettuce Carrots Peanuts Code provided in the assignment ItemNode.java:arrow_forward
- Computer Science PLEASE HELP TO CONVERT THIS FOR LOOP TO FOREACH LOOP using System;using System.Collections.Generic; namespace SwinAdventure{public class IdentifierableObject{//field ,datapublic List<string> Identifier = new List<string>(); //constructorpublic IdentifierableObject(string[] ident){for (int i = 0; i < ident.Length; i++){Identifier.Add(ident[i]);}} // Methodpublic void AddIdentifier(string id){Identifier.Add(id.ToLower());} // propertiespublic string FirstID{get{return Identifier[0];}} public bool AreYou(string id){for (int i = 0; i < Identifier.Count; i++){if (Identifier[i] == id.ToLower()){return true;} }return false;}}}arrow_forwardImplement a Single linked list to store a set of Integer numbers (no duplicate) • Instance variable• Constructor• Accessor and Update methods 3. Define TestSLinkedList Classa. Declare an instance of the Single List class.b. Test all the methods of the Single List class.arrow_forwardConsider the instance variables and constructors. Given Instance Variables and Constructors: public class SimpleLinkedList<E> implements SimpleList<E>, Iterable<E>, Serializable { // First Node of the List private Node<E> first; // Last Node of the List private Node<E> last; // Number of List Elements private int count; // Total Number of Modifications (Add and Remove Calls) private int modCount; /** * Creates an empty SimpleLinkedList. */ publicSimpleLinkedList(){ first = null; last = null; count = 0; modCount = 0; } ... Assume the class contains the following methods that work correctly: public boolean isEmpty() public int size() public boolean add(E e) public E get(int index) private void validateIndex(int index, int end) Complete the following methods based on the given information from above. /** * Adds an element to the list at the…arrow_forward
- Given main() and an IntNode class, complete the IntList class (a linked list of IntNodes) by writing the insertInDescendingOrder() method to insert new IntNodes into the IntList in descending order. Ex. If the input is: 3 4 2 5 1 6 7 9 8 -1 the output is: 9 8 7 6 5 4 3 2 1 import java.util.Scanner; public class SortedList { public static void main (String[] args) {Scanner scnr = new Scanner(System.in);IntList intList = new IntList();IntNode curNode;int num; num = scnr.nextInt(); while (num != -1) {// Insert into linked list in descending order curNode = new IntNode(num);intList.insertInDescendingOrder(curNode);num = scnr.nextInt();}intList.printIntList();}}arrow_forwardComplete the code for the removeFirst method, which should remove and return the first element in the linked list. Throw aNoSuchElementException if the method is invoked on an empty list. import java.util.NoSuchElementException; public class LinkedList { private Node first; public LinkedList() { first = null; } public Object getFirst() { if (first == null) { throw new NoSuchElementException(); } return first.data; } public Object removeFirst() { // put your code here } class Node { public Object data; public Node next; } }arrow_forwardGiven main() and an IntNode class, complete the IntList class (a linked list of IntNodes) by writing the insertInAscendingOrder() method that inserts a new IntNode into the IntList in ascending order. Ex. If the input is: 8 3 6 2 5 9 4 1 7 -1 the output is: 1 2 3 4 5 6 7 8 9 import java.util.Scanner; public class SortedList {public static void main (String[] args) {Scanner scnr = new Scanner(System.in);IntList intList = new IntList();IntNode curNode;int num;num = scnr.nextInt();// Read in until -1while (num != -1) {// Insert into linked listcurNode = new IntNode(num);intList.insertInAscendingOrder(curNode);num = scnr.nextInt();}intList.printIntList();}}arrow_forward