Use a SinglyLinked List to implement a Queue
a. Define a Queue interface.
b. Define a LinkedQueue class (Node class)..
c. Define a Test class to test all methods
help me make a test class
first code
third code
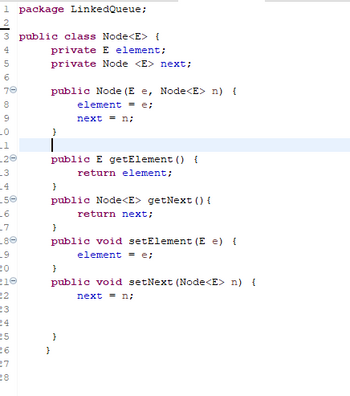

The code you've provided is a Java implementation of a queue using a singly-linked list. Here's a breakdown of the code:
Queue
interface: It defines the methods that a queue should have, includingenqueue
,dequeue
, andisEmpty
.Node
class: This is a simple class representing a node in the singly-linked list. Each node holds an integer value (data
) and a reference to the next node in the list (next
).LinkedQueue
class: It implements theQueue
interface. This class maintains a reference to thehead
andtail
nodes of the linked list.enqueue(int data)
: Adds a new node with the specified data to the end of the queue. If the queue is empty, bothhead
andtail
are set to the new node.dequeue()
: Removes and returns the data from the front of the queue. If the queue is empty, it returns -1. If the queue becomes empty after dequeue, bothhead
andtail
are set to null.isEmpty()
: Checks if the queue is empty by inspecting thehead
reference.
Main
class: The test class demonstrates how to use theLinkedQueue
. It creates an instance ofQueue
using theLinkedQueue
class, enqueues three elements, and then dequeues and prints them in the order they were added.
Step by stepSolved in 5 steps with 2 images

- Implement the Linked List using head and tail pointer. Interface (.h file) of LinkedList class is given below. Your task is to provide implementation (.cpp file) for LinkedList class. Interface: template<class Type> class LinkedList { private: Node<Type>* head; Node<Type>* tail; <Type> data; public: LinkedList<Type>(); LinkedList<Type>(const LinkedList<Type> &obj); void sortedInsert(Type); Type deleteFromPosition(int position); //deletes the node at the particular position Type delete(int start,int end); //deletes the nodes from starting to ending position bool isEmpty()const; void print()const; ~LinkedList(); }; program in c++.arrow_forwardAdd the three classic operations of Queues in the MagazineList.java to make it become a queue. Add testing code in MagazineRack.java to test your code. (You do not need to modify Magazine.java) Magazine.java : public class Magazine {private String title;//-----------------------------------------------------------------// Sets up the new magazine with its title.//-----------------------------------------------------------------public Magazine(String newTitle){ title = newTitle;}//-----------------------------------------------------------------// Returns this magazine as a string.//-----------------------------------------------------------------public String toString(){return title;}} MagazineList.java : attached the image below MagazineRack.java: public class MagazineRack{//----------------------------------------------------------------// Creates a MagazineList object, adds several magazines to the// list, then prints…arrow_forwardJAVA please Given main() in the ShoppingList class, define an insertAtEnd() method in the ItemNode class that adds an element to the end of a linked list. DO NOT print the dummy head node. Ex. if the input is: 4 Kale Lettuce Carrots Peanuts where 4 is the number of items to be inserted; Kale, Lettuce, Carrots, Peanuts are the names of the items to be added at the end of the list. The output is: Kale Lettuce Carrots Peanuts Code provided in the assignment ItemNode.java:arrow_forward
- I want convert the code from singly-linked list to doubly-linked list package Problem; class Node{ private Object value; // Holds the data of the node private Node next; // Holds the follwoing node's address Node(Object v, Node n){ this.value =v; this.next = n; } public Node getNext(){ return this.next; } public Object getValue(){ return this.value; } public void setNext(Node n){ this.next=n; } public void setValue(Object v) { this.value=v; } } class SLL{ public Node head; SLL(){ this.head=null; } public void display(){ Node curr = this.head; while(curr != null) { System.out.print(curr.getValue()); if(curr.getNext()!=null)…arrow_forwardConsider the instance variables and constructors. Given Instance Variables and Constructors: public class SimpleLinkedList<E> implements SimpleList<E>, Iterable<E>, Serializable { // First Node of the List private Node<E> first; // Last Node of the List private Node<E> last; // Number of List Elements private int count; // Total Number of Modifications (Add and Remove Calls) private int modCount; /** * Creates an empty SimpleLinkedList. */ publicSimpleLinkedList(){ first = null; last = null; count = 0; modCount = 0; } ... Assume the class contains the following methods that work correctly: public boolean isEmpty() public int size() public boolean add(E e) public E get(int index) private void validateIndex(int index, int end) Complete the following methods based on the given information from above. /** * Adds an element to the list at the…arrow_forwardA stack-ended queue, sometimes known as a steque, is a data type that allows push, pop, and enqueue operations. Make an API for this ADT. Create a linked-list implementation.arrow_forward
- I want convert the code from singly-linked list to doubly-linked list package Problem; class Node{ private Object value; // Holds the data of the node private Node next; // Holds the follwoing node's address Node(Object v, Node n){ this.value =v; this.next = n; } public Node getNext(){ return this.next; } public Object getValue(){ return this.value; } public void setNext(Node n){ this.next=n; } public void setValue(Object v) { this.value=v; } } class SLL{ public Node head; SLL(){ this.head=null; } public void display(){ Node curr = this.head; while(curr != null) { System.out.print(curr.getValue()); if(curr.getNext()!=null)…arrow_forwardUse a Doubly Linked List to implement a Dequea. Define a Deque interface.b. Define a LinkedDeque class (Node class)..c. Define a Test class to test all methods help me make a test class first code package Deque; public class DLinkedList<E> { private Node<E> header = null; private Node<E> trailer = null; private int size = 0; public DLinkedList() { header = new Node<>(null, null,null); trailer = new Node<>(null,header,null); header.setNext(trailer); size = 0; } public int getSize() { return size; } public boolean isEmpty() { return size ==0; } public E getFirst(){ if(isEmpty()) { return null; } return header.getNext().getElement(); } public E getLast(){ if(isEmpty()) { return null; } return trailer.getPrev().getElement(); } public void addFirst(E e) { addBetween(e,header,header.getNext()); } public void addLast(E e) { addBetween(e,trailer.getPrev(), trailer); } public E removeFirst() { if (isEmpty()) { return null; } return…arrow_forwardGiven main() and an IntNode class, complete the IntList class (a linked list of IntNodes) by writing the insertInDescendingOrder() method to insert new IntNodes into the IntList in descending order. Ex. If the input is: 3 4 2 5 1 6 7 9 8 -1 the output is: 9 8 7 6 5 4 3 2 1 import java.util.Scanner; public class SortedList { public static void main (String[] args) {Scanner scnr = new Scanner(System.in);IntList intList = new IntList();IntNode curNode;int num; num = scnr.nextInt(); while (num != -1) {// Insert into linked list in descending order curNode = new IntNode(num);intList.insertInDescendingOrder(curNode);num = scnr.nextInt();}intList.printIntList();}}arrow_forward