truct in #1, write a function that takes an array of soccer players and its size as arguments and returns the index of the player who scored the most points. 4. Write a Circle class that has the following member variables: • radius : a double • pi : a double initialized with the value 3.14159 The class should have the following member functions: • Default Constructor. A default constructor that sets radius to 0.0. • Constructor. Accepts the radius of the circle as an argument. • setRadius. A mutator function for the radius variable.
1. Define a struct for a soccer player that stores their name, jersey number, and total
points scored.
2. Using the struct in #1, write a function that takes an array of soccer players and its
size as arguments and returns the average number of points scored by the players.
3. Using the struct in #1, write a function that takes an array of soccer players and its
size as arguments and returns the index of the player who scored the most points.
4. Write a Circle class that has the following member variables:
• radius : a double
• pi : a double initialized with the value 3.14159
The class should have the following member functions:
• Default Constructor. A default constructor that sets radius to 0.0.
• Constructor. Accepts the radius of the circle as an argument.
• setRadius. A mutator function for the radius variable.
• getRadius. An accessor function for the radius variable.
• getArea. Returns the area of the circle, which is calculated as area = pi * radius *
radius
• getCircumference. Returns the circumference of the circle, which is calculated as
circumference = 2 * pi * radius
5. Define a function to sum the elements in an array, but use pointer notation rather
than array notation whenever possible.
6. Write a swap function, that swaps the values of two variables in main, but use
pointers instead of reference parameters.
7. Write a function that takes an array of ints and its size as arguments. It should
create a new array that is the same size as the argument. It should set the values in
the new array by adding 10 to each element in the original array. The function
should return a pointer to the new array. (Make sure you use dynamic memory
allocation)
Please can i get solution for sub parts, 3, 4, 5, 6, 7.
thank you

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

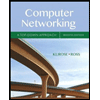
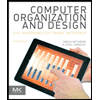
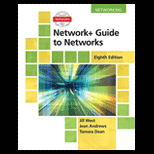
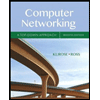
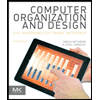
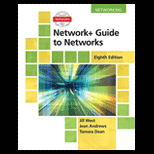
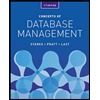
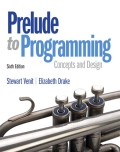
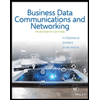