tried this code provided from an expert but for some reason, nothing is being shown on the console after I ran it. And the expert seems to have an output shown to him. is there any problem with this code
#include<stdio.h>
#include<stdlib.h>
#include<string.h>
/* function that converts the change into count of 50,20,10,5 and stores the count in changeArray*/
int coinChange(int changeArray[10][4],int index,int change)
{
int count50=0,count20=0,count10=0,count5=0;
while(change > 0)
{
if(change > 0 && change <=95 && change% 5 == 0)
{
if(change >= 50)
{
change -= 50;
count50++;
}
else if(change >= 20)
{
change -= 20;
count20++;
}
else if(change>=10)
{
change -= 10;
count10++;
}
else if(change>=5)
{
change -= 5;
count5++;
}
}
}
changeArray[index][0]=count50;
changeArray[index][1]=count20;
changeArray[index][2]=count10;
changeArray[index][3]=count5;
return change;
}
/* function that reads 'coins.txt' and stores names in names array, coin values in coins array, compute change and stores change count in change array */
int readFromFile(char names[10][100],int coins[],int change[10][4])
{
int index=-1,i=0;
FILE *fp;
fp=fopen("coins.txt","r");
if(fp==NULL)
{
printf("File doesnot exists\n");
exit(0);
}
char line[128];
while(fgets(line,sizeof(line),fp)!=NULL)
{
index++;
int i=0;
while(line[i]!=' ')
{
names[index][i]=line[i];
i++;
}
names[index][i]='\0';
i++;
int value=0;
while(line[i]!='\n')
{
value=value*10+(int)line[i]-48;
i++;
}
coins[index]=value;
coinChange(change,index,value);
}
fclose(fp);
return index+1;
}
/* function that takes new name as input and search for it names array, if present then display its corresponding details else prints Not found */
void searchAndPrint(char names[10][100],char name[],int coins[10],int change[10][4],int index)
{
int found=-1,i,j;
int values[]={50,20,10,5};
for(i=0;i<10;i++) {
if(strcmp(names[i],name)==0)
{
found=i;
break;
}
}
if(found==-1)
printf("Not Found\n");
else
{
for(j=0;j<4;j++)
{
if(change[found][j]!=0)
printf("%d cents: %d\n",values[j],change[found][j]);
}
}
}
/* function that creates csv files and inserts all data into csv file */
void createAndInsertIntoCSV(char names[10][100],int coins[10],int change[10][4],int index)
{
FILE *fp=fopen("coins.csv","w+");
int i;
fprintf(fp,"%s,%s,%s,%s,%s,%s\n","Name","Change Value","50 count","20 count","10 count","5 count");
for(i=0;i<10;i++) fprintf(fp,"%s,%d,%d,%d,%d,%d\n",names[i],coins[i],change[i][0],change[i][1],change[i][2],change[i][3]);
}
int main()
{
char names[10][100];
int coins[10];
int change[10][4];
int index=readFromFile(names,coins,change); // index represents the no. of records in txt file
while(1)
{
printf("\n1--->Enter name\n2--->Exit\n\nEnter your choice:");
int choice;
scanf("%d",&choice);
if(choice==1)
{
char name[100];
printf("Customer:\n");
scanf("%s",name);
printf("Name: %s\n",name);
searchAndPrint(names,name,coins,change,index);
}
else
{
createAndInsertIntoCSV(names,coins,change,index);
printf("CSV file is generated and saved in present working directory with name \"coins.csv\"\n");
break;
}
}
}
I tried this code provided from an expert but for some reason, nothing is being shown on the console after I ran it. And the expert seems to have an output shown to him. is there any problem with this code?

Step by step
Solved in 2 steps with 1 images

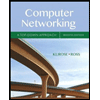
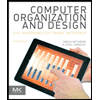
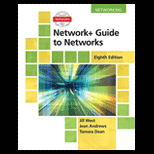
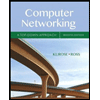
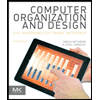
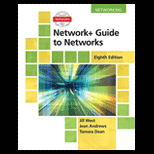
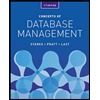
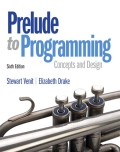
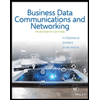