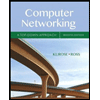
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Title: Using Comparable in Singly Linked List
I'm writing a singly linked list that can insert, remove, search, and count. When I try to implement generic so that the list can accept different types, the instruction requires the use of Comparable. How is Comparable used in this case (since I'm not sorting anything), and what exactly am I comparing? Do I need to "implements Comparable" or "extends Comparable"?
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 2 steps

Knowledge Booster
Similar questions
- help me understand this please (this is not exam question, its from online textrbook)arrow_forwardThis is in c++ Given the MileageTrackerNode class, complete main() to insert nodes into a linked list (using the InsertAfter() function). The first user-input value is the number of nodes in the linked list. Use the PrintNodeData() function to print the entire linked list. Don't print the dummy head node. Example input : 3 2.2 7/2/18 3.2 7/7/18 4.5 7/16/18 output: 2.2, 7/2/18 3.2, 7/7/18 4.5, 7/16/18 main.cpp: #include "MileageTrackerNode.h"#include <string>#include <iostream>using namespace std; int main (int argc, char* argv[]) {// References for MileageTrackerNode objectsMileageTrackerNode* headNode;MileageTrackerNode* currNode;MileageTrackerNode* lastNode; double miles;string date;int i; // Front of nodes listheadNode = new MileageTrackerNode();lastNode = headNode; // TODO: Read in the number of nodes // TODO: For the read in number of nodes, read// in data and insert into the linked list // TODO: Call the PrintNodeData() method// to print the entire linked list //…arrow_forwardImplement a program that creates an unsorted list by using a linked list implemented by yourself. NOT allowed to use LinkedList class or any other classes that offers list functions. It is REQUIRED to use an ItemType class and a NodeType struct to solve this. Use C++! The “data.txt” file has three lines of data 100, 110, 120, 130, 140, 150, 160 100, 130, 160 1@0, 2@3, 3@END You need to create an empty unsorted list add the numbers from the first line to list using putItem() function. Then print all the current keys to command line in one line using printAll(). delete the numbers given by the second line in the list by using deleteItem() function. Then print all the current keys to command line in one line using printAll().. putItem () the numbers in the third line of the data file to the corresponding location in The list. For example, 1@0 means adding number 1 at position 0 of the list. Then print all the current keys to command line in one line…arrow_forward
- Implementation of largest-cover difference #23@title Implementation of largest-cover difference def rectangle_difference(r, t): "Computes the rectangle difference rt, and outputs the result as a list of rectangles." assert len(r) == len(t), "Rectangles have different dimensions" ### YOUR SOLUTION HERE In the above code, we notice how it paid off to encapsulate intervals in their own abstraction. Now that we need to reason about rectangles, we do not need to get bogged down into complicated considerations of how to perform difference; the comparisons between endpoints are all done in the context of intervals, where it is easier to reason about them. It is often the case that problems become easy, once you think at them in the appropriate context. It is much easier to develop the code for the difference of intervals, as we have done, and then move on to difference of rectangles, rather than trying to write code for rectangle difference directly. The result is also far more elegant. # Let…arrow_forwardPlease do this in C++. The only parts needed are commented as //TODO in MAIN. Please do not use cout, the PrintNodeData in MileageTrackerNode.cpp needs to be called. I am struggling with adding data into the linked list. Given the MileageTrackerNode class, complete main() to insert nodes into a linked list (using the InsertAfter() function). The first user-input value is the number of nodes in the linked list. Use the PrintNodeData() function to print the entire linked list. DO NOT print the dummy head node. Ex. If the input is: 3 2.2 7/2/18 3.2 7/7/18 4.5 7/16/18 the output is: 2.2, 7/2/18 3.2, 7/7/18 4.5, 7/16/18 Main.cpp #include "MileageTrackerNode.h"#include <string>#include <iostream>using namespace std; int main (int argc, char* argv[]) {// References for MileageTrackerNode objectsMileageTrackerNode* headNode;MileageTrackerNode* currNode;MileageTrackerNode* lastNode; double miles;string date;int i; // Front of nodes listheadNode = new…arrow_forwardImplement a simple linked list in Python (Write source code and show output) with basic linked list operations like: (a) create a sequence of nodes and construct a linear linked list. (b) insert a new node in the linked list. (b) delete a particular node in the linked list. (c) modify the linear linked list into a circular linked list. Use this template: class Node:def __init__(self, val=None):self.val = valself.next = Noneclass LinkedList:"""TODO: Remove the "pass" statements and implement each methodAdd any methods if necessaryDON'T use a builtin list to keep all your nodes."""def __init__(self):self.head = None # The head of your list, don't change its name. It shouldbe "None" when the list is empty.def append(self, num): # append num to the tail of the listpassdef insert(self, index, num): # insert num into the given indexpassdef delete(self, index): # remove the node at the given index and return the deleted value as an integerpassdef circularize(self): # Make your list circular.…arrow_forward
- In this task you will work with the linked list of digits we have created in the lessons up to this point. As before you are provided with some code that you should not modify: A structure definition for the storage of each digit's information. A main() function to test your code. The functions createDigit(), append(), printNumber(), freeNumber(), readNumber() and divisibleByThree() (although you may not need to use all of these). Your task is to write a new function changeThrees() which takes as input a pointer that holds the address of the start of a linked list of digits. Your function should change all of those digits in this linked list that equal 3 to the digit 9, and count how many replacements were made. The function should return this number of replacements. Provided codearrow_forwardPlease just use main.cpp,sequence.cpp and sequence.h. It has provided the sample insert()function. No documentation (commenting) is required for this assignment. This assignment is based on an assignment from "Data Structures and Other Objects Using C++" by Michael Main and Walter Savitch. Use linked lists to implement a Sequence class that stores int values. Specification The specification of the class is below. There are 9 member functions (not counting the big 3) and 2 types to define. The idea behind this class is that there will be an internal iterator, i.e., an iterator that the client cannot access, but that the class itself manages. For example, if we have a Sequence object named s, we would use s.start() to set the iterator to the beginning of the list, and s.advance() to move the iterator to the next node in the list. (I'm making the analogy to iterators as a way to help you understand the point of what we are doing. If trying to think in terms of iterators is confusing,…arrow_forwardI'm trying to delete a node after inputting data for 2-3 checks but the node I try to delete remains in the list. How do I fix my deleteCheck() function to run correctly with the rest of my code? Thank you. #include <stdio.h>#include <stdlib.h>// Austin Chong// The core concept of this assignment is to use a linked list in a C program.// 07 March 2020 struct checkNode{int checkNumber;char date[8];char paidTo[100];char description[100];double amount;struct checkNode *head;struct checkNode *next;}; struct checkNode *checkBook = NULL; /* Functions */ void addCheck(){int checkNumber;double amount;char temp;struct checkNode *newCheck = (struct checkNode*) malloc(sizeof(struct checkNode));printf("Please enter the check number: ");scanf("%d", &checkNumber);newCheck -> checkNumber = checkNumber;printf("Please enter the amount: ");scanf("%lf", &amount);newCheck -> amount = amount;printf("Please enter the date: ");scanf("%c", &temp);scanf("%[^\n]", newCheck ->…arrow_forward
- You will create two programs. The first one will use the data structure Stack and the other program will use the data structure Queue. Keep in mind that you should already know from your video and free textbook that Java uses a LinkedList integration for Queue. Stack Program Create a deck of cards using an array (Array size 15). Each card is an object. So you will have to create a Card class that has a value (1 - 10, Jack, Queen, King, Ace) and suit (clubs, diamonds, heart, spade). You will create a stack and randomly pick a card from the deck to put be pushed onto the stack. You will repeat this 5 times. Then you will take cards off the top of the stack (pop) and reveal the values of the cards in the output. As a challenge, you may have the user guess the value and suit of the card at the bottom of the stack. Queue Program There is a new concert coming to town. This concert is popular and has a long line. The line uses the data structure Queue. The people in the line are objects…arrow_forwardin this assignment i have to remove vowels from a string using a linked list. the linked list and link code is from a textbook and cannot be changed if the code alters the data structure. for some reason when i implement this code it gives me a logical error where the program only removes all instances of the first vowel in a string instead of moving through all vowels of the string and removing each one with all instances. i would appreciate if you could tell me the problem and solution in words and not in code. The code is in java. output please enter a string.researchhcraeserfalseList (first -->last): researchList (first -->last): researchList (first -->last): rsarch CODE MAIN FUNCTION import java.util.Scanner;/*** Write a description of class test here.** @author (your name)* @version (a version number or a date)*/public class test{// instance variables - replace the example below with your ownpublic static void main(String[] args){Scanner input = new…arrow_forwardin C++ Write a method ‘void addBack(double x)’ that adds value x to the back of a linked list. Assume you have access to a ‘head’ pointer pointing to the first node in the list. Please note that you do NOT have a tail pointer. Be sure to check for any special cases.arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
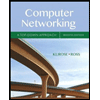
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
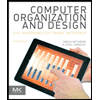
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
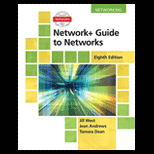
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
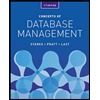
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
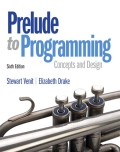
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
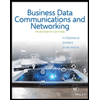
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY