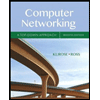
Concept explainers
class dvd:
def __init__(self, title, director, producer, release_date, duration, dvd_id):
self.title = title
self.director = director
self.producer = producer
self.release_date = release_date
self.duration = duration
self.dvd_id = dvd_id
def get_title(self):
return self.title
def get_director(self):
return self.director
def get_producer(self):
return self.producer
def get_release_date(self):
return self.release_date
def get_duration(self):
return self.duration
def get_dvd_id(self):
return self.dvd_id
def __repr__(self):
return "Movie Name: "+self.title+", \nDirector : "+self.director+", \nProducer : "+self.producer+", \nRelease Date : "+str(self.release_date)+", \nDuration : "+str(self.duration)+", \nDVD ID : "+str(self.dvd_id)
class customer:
def __init__(self, name, customer_id):
self.name = name
self.dvds=[]
self.customer_id = customer_id
def get_name(self):
return self.name
def get_customer_id(self):
return self.customer_id
def getDvds(self):
s=""
for d in self.dvds:
s=s+d.__repr__()+"\n"
return s
def __repr__(self):
return "Customer Name : "+self.name+"\nCustomer ID : "+str(self.customer_id)+"\n Rented DVD Details : "+self.getDvds()
class store:
def __init__(self):
self.dvds = []
self.customers = []
def add_dvd(self, dvd):
self.dvds.append(dvd)
def add_customer(self, customer):
self.customers.append(customer)
def rent_dvd(self, dvd_id, customer_id):
for dvd in self.dvds:
if dvd.get_dvd_id() == dvd_id:
for customer in self.customers:
if customer.get_customer_id() == customer_id:
customer.dvds.append(dvd)
self.dvds.remove(dvd)
def return_dvd(self, dvd_id, customer_id):
for customer in self.customers:
if customer.get_customer_id() == customer_id:
for dvd in customer.dvds:
if dvd.get_dvd_id() == dvd_id:
self.dvds.append(dvd)
customer.dvds.remove(dvd)
def get_dvd(self, dvd_id):
for dvd in self.dvds:
if dvd.get_dvd_id() == dvd_id:
return dvd
def get_customer(self, customer_id):
for customer in self.customers:
if customer.get_customer_id() == customer_id:
return customer
def get_dvds(self):
return self.dvds
def get_customers(self):
return self.customers
def get_dvds_for_customer(self, customer_id):
for customer in self.customers:
if customer.get_customer_id() == customer_id:
return customer.dvds
# main
store = store()
dvd1 = dvd("Iron Man", "Jon Favreau", "Avi Arad/Kevin Feige", 2008, 156, 1)
dvd2 = dvd("The Incredible Hulk", "Louis Leterrier", "Avi Arad/Gale Anne Hurd/Kevin Feige", 2008, 91, 2)
dvd3 = dvd("Iron Man 2", "Jon Favreau", "Kevin Feige", 2010, 150, 3)
dvd4 = dvd("Thor", "Kenneth Branagh", "Kevin Feige", 2011, 144, 4)
dvd5 = dvd("Captain America: The First Avenger", "Joe Johnston", "Kevin Feige", 2011, 144, 5)
dvd6 = dvd("The Avengers", "Joss Whedon", "Kevin Feige", 2012, 133, 6)
dvd7 = dvd("Iron Man 3", "Shane Black", "Kevin Feige", 2013, 126, 7)
dvd8 = dvd("Thor: The Dark World ", "Kenneth Branagh", "Kevin Feige", 2013, 114, 8)
dvd9 = dvd("Guardians of the Galaxy", "James Gunn", "Kevin Feige", 2014, 120, 9)
dvd10 = dvd("Avengers: Age of Ultron", "Joss Whedon", "Kevin Feige", 2015, 132, 10)
store.add_dvd(dvd1)
store.add_dvd(dvd2)
store.add_dvd(dvd3)
store.add_dvd(dvd4)
store.add_dvd(dvd5)
store.add_dvd(dvd6)
store.add_dvd(dvd7)
store.add_dvd(dvd8)
store.add_dvd(dvd9)
store.add_dvd(dvd10)
customer1 = customer("Willim Harvay", 1)
customer2 = customer("Robort Root", 2)
customer3 = customer("Romina Daniel", 3)
customer4 = customer("Ravi Chaudri", 4)
customer5 = customer("Elsa Gorgeo", 5)
customer6 = customer("John Trimander", 6)
customer7 = customer("Srabell Carnico", 7)
customer8 = customer("Mike samson", 8)
customer9 = customer("Shorlok Williamson", 9)
customer10 = customer("Rocky simon", 10)
store.add_customer(customer1)
store.add_customer(customer2)
store.add_customer(customer3)
store.add_customer(customer4)
store.add_customer(customer5)
store.add_customer(customer6)
store.add_customer(customer7)
store.add_customer(customer8)
store.add_customer(customer9)
store.add_customer(customer10)
store.rent_dvd(1, 1)
store.rent_dvd(2, 1)
store.rent_dvd(3, 2)
store.rent_dvd(3, 3)
store.return_dvd(2, 1)
store.rent_dvd(1, 1)
store.rent_dvd(2, 1)
store.rent_dvd(3, 2)
store.rent_dvd(3, 3)
store.return_dvd(2, 1)
Create the management method for the following classes,
a)Admin mode:
- See the Available List of DVD
- See the rent-out DVD list
- Add DVD
- Add Customer
- Remove DVD
- Remove Customer
b)Customer mode:
- See the Available List of DVD
- Rent a DVD
- Return a DVD

Step by stepSolved in 2 steps

- In C#, a program that implements and uses a class called MyRectangle. Data items should include: length, width, color, and label. Define properties with appropriate accessor functions for each of the data items. Methods should include a default constructor that sets values to the empty string or zero, a constructor that allows the user to specify all values for the data items, a member method that computes and returns the area of the shape, and a member method called DisplayShape that outputs all the information (including the area via the ComputeArea method for the given rectangle in a reasonable format. Sample output belowarrow_forwardclass dvd: def __init__(self, title, director, producer, release_date, duration, dvd_id): self.title = title self.director = director self.producer = producer self.release_date = release_date self.duration = duration self.dvd_id = dvd_id def get_title(self): return self.title def get_director(self): return self.director def get_producer(self): return self.producer def get_release_date(self): return self.release_date def get_duration(self): return self.duration def get_dvd_id(self): return self.dvd_id class customer: def __init__(self, name, customer_id): self.name = name self.customer_id = customer_id def get_name(self): return self.name def get_customer_id(self): return self.customer_id class store: def __init__(self): self.dvds = [] self.customers = [] def add_dvd(self,…arrow_forwardActivity name: activity_main.xmlJava File: MainActivity.javaWrite the code needed to initialize binding variable in OnCreate method in MainActivity.java file.arrow_forward
- This should be done with Visual Studio C# console app net core Thanks!arrow_forwardCOURSE CRS_CODE DEPT_CODE CRS_DESCRIPTION CRS_CREDIT CLASS CLASS_CODE CRS_CODE CLASS_SECTION CLASS_TIME CLASS ROOM PROF_NUM 00 ENROLL CLASS_CODE STU_NUM ENROLL_GRADE STUDENT STU_NUM STU_LNAME STU_FNAME STU_INIT STU_DOB STU_HRS STU_CLASS STU_GPA STU_TRANSFER DEPT_CODE STU_PHONE PROF_NUM Review the relational diagram above and briefly describe the following in one sentence. 1. How would you extend this database to identify the Professor who taught a class? 2. How would you extend this database to identify the Department that had a course?arrow_forwardPython Required information id fname lname company address city ST zip 103 Art Venere 8 W Cerritos Ave #54 Bridgeport NJ 8014 104 Lenna Paprocki Feltz Printing 639 Main St Anchorage AK 99501arrow_forward
- 1.Problem Description Student information management system is used to input, display student information records. 1. The GUI interface for inputing ia designed as follows: The top part are the student imformation used to input student information, and the bottom part are four buttonns, 2. each button meaning: 3. (2) Total: add java score and C++ score, and display the result; 4. (3) Save: save student record into file named student.dat; 5. (4) Clear: set all fields on GUI to empty string: 6. (5) Close: close the window of GUI Student ID: Java Score: Note: Total Student ID: BC501 Java Score: 80 Note: He study java hard. Total Student Management System Student name: C++ Score: Save C++ Score: 90.5 Student Management System Student name: ALVIN CARM Save Total scores: Clear Sex Clear D E Male Female Close Sex Male Female Total scores: 170.5 Closearrow_forwardclass Widget: """A class representing a simple Widget === Instance Attributes (the attributes of this class and their types) === name: the name of this Widget (str) cost: the cost of this Widget (int); cost >= 0 === Sample Usage (to help you understand how this class would be used) === >>> my_widget = Widget('Puzzle', 15) >>> my_widget.name 'Puzzle' >>> my_widget.cost 15 >>> my_widget.is_cheap() False >>> your_widget = Widget("Rubik's Cube", 6) >>> your_widget.name "Rubik's Cube" >>> your_widget.cost 6 >>> your_widget.is_cheap() True """ # Add your methods here if __name__ == '__main__': import doctest # Uncomment the line below if you prefer to test your examples with doctest # doctest.testmod()arrow_forwardcan someone help me or teach whats wrong with my code please badly needed this nightarrow_forward
- // Declare data fields: a String named customerName, // an int named numItems, and // a double named totalCost.// Your code here... // Implement the default contructor.// Set the value of customerName to "no name"// and use zero for the other data fields.// Your code here... // Implement the overloaded constructor that// passes new values to all data fields.// Your code here... // Implement method getTotalCost to return the totalCost.// Your code here... // Implement method buyItem.//// Adds itemCost to the total cost and increments// (adds 1 to) the number of items in the cart.//// Parameter: a double itemCost indicating the cost of the item.public void buyItem(double itemCost){// Your code here... }// Implement method applyCoupon.//// Apply a coupon to the total cost of the cart.// - Normal coupon: the unit discount is subtracted ONCE// from the total cost.// - Bonus coupon: the unit discount is subtracted TWICE// from the total cost.// - HOWEVER, a bonus coupon only applies if the…arrow_forwardfile operator code 1 // The FileOperator class that includes methods for file input and output 2 // Maham Hameed 3 import java.io.*; 4 public class FileOperator 5 { 6 // instance variables 7 private File m_file; 8 9 // constructor 10 // Do not make any changes to this method! 11 public FileOperator() 12 { 13 String fileName = "employeeData.txt"; 14 m_file = new File(fileName); 15 } 16 17 // This method writes an array of Employees into a physical file. 18 // Each line is in this format: "S-Optimus Prime-Computer Science-$1200" 19 // "S" represents StudentWorker ("F" represents Faculty) 20 public void writeFile(Employee[] employees) 21 { 22 // TODO: implement this method 23 } 24 }arrow_forwardRequired information id fname lname company address city ST zip 103 Art Venere 8 W Cerritos Ave #54 Bridgeport NJ 8014 104 Lenna Paprocki Feltz Printing 639 Main St Anchorage AK 99501arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
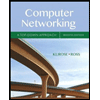
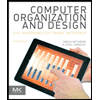
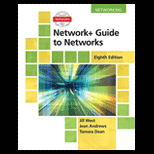
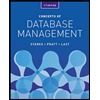
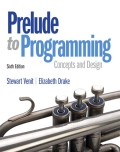
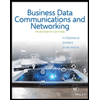