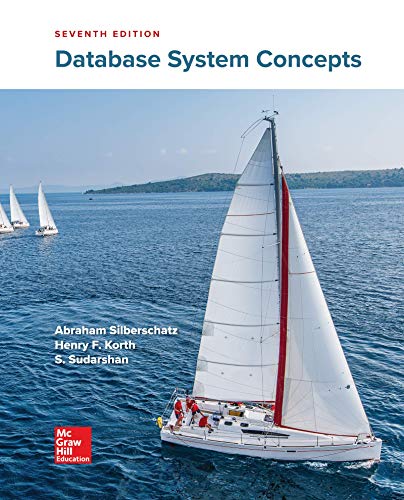
python code
CSIS 153 Fall 2020 Program 9 – Inheritance
- Create a class called Vehicle and store it in a file called modVehicle.py
class Vehicle |
- numVehicles: integer #class-level attribute _nextVinToUse: integer #class-level attribute -VIN : integer #instance-level attribute - isNew boolen #True if new, False if used - color: string #red, silver, blue, green, brown, gray |
<<constructor>> Vehicle(tmpYear:int, tmpColor:string) +getVin( ):integer -setVin( )_void #PRIVATE method will access the nextVinToUse, # AND increment nextVinToUse # assign to VIN as string +getYearManufactured( ): int +calcVehicleAge( ): int #subtract yearManufactured from the current year +str( ): string #return a string where each attribute is labeled AND |
- Create a class called Car which is a child of the Vehicle class. Store it in the modVehicle.py file.
class Car(Vehicle) |
-numHondas: integer #class-level attribute -make: string #Ford, Subaru, Toyota, Honda, etc. |
<<constructor>>Car(tmpYear:integer, tmpColor: string, tmpMake:string) +getType( ): string +getNumHondas():integar +str( ): string #prints ALL of the attributes with labels |
- Create a test class that thoroughly tests EVERY method of each of the classes. Carefully label your output to illustrate what is being tested.
V1 = Vehicle(“C123”, 2010,”Blue”);
Print(“printing v1info: “, v1)
Print(“Testing getters:”)
Print(“retrieving VIN: “, v1.getVin())
Etc
Print(“Testing settings”)

Step by stepSolved in 3 steps with 2 images

- class Product: def __init__(self, product_id, name, price, quantity): self.product_id = product_id self.name = name self.price = round(price, 2) self.quantity = quantity def get_product_description(self): return (f"Product ID: {self.product_id}, " f"Name: {self.name}, " f"Price: ${self.price:.2f}, " f"Quantity Available: {self.quantity}") class ElectronicProduct(Product): def __init__(self, product_id, name, price, quantity,warranty_period): self.warranty_period = warranty_period super().__init__(product_id,name,price,quantity) def get_product_description(self): base_description = super().get_product_description() return base_description + f"\nWarranty: {self.warranty_period}" class ClothingProduct(Product): def __init__(self, product_id, name, price, quantity,size): self.size = size…arrow_forwardAssignment 2 A Student class has the following attributes: String name; String streetAddress; int age; String phonNumber; String mobileNumber; Requirements: . Create the setters and getters . Create one default constructor • create one paramertized constructor • Create an object of the class using the default constructor . ● . Create another object of the class using the paramertized constructor Make sure to suply the values from the keyboard Print the information about each employee . Make sure to add comments to you code and that your code is indented corectly . Use the camelCase naming convension. . Use try and catch block. After you are doneo a samle run and tak a screenshot of it. zip the screenshot and the sourc code file and upload the zipped file to the Blackboard.arrow_forwardWhen a class uses dynamically allocated objects for its members and it does not have a copy assignment operator what of the following could happen (mark all that apply) There could be runtime errors There could be a double frees (double delete errors) There could be memory leaks Nothing happens the compiler provides the correct constructor There could be compile time errorsarrow_forward
- 8. Python concer # TODO: Define BankAccount class # TODO: Define constructor with parameters to initialize instance attributes # TODO: Define deposit_checking() # TODO: Define deposit_savings() # TODO: Define withdraw_checking() # TODO: Define withdraw_savings() # TODO: Define transfer_to_savings() if __name__ == "__main__": account = BankAccount("Mickey", 500.00, 1000.00) account.checking_balance = 500 account.savings_balance = 500 account.withdraw_savings(100) account.withdraw_checking(100) account.transfer_to_savings(300) print(account.name) print('${:.2f}'.format(account.checking_balance)) print('${:.2f}'.format(account.savings_balance.arrow_forwardCreate student object class (Student.java) Student objects should have the following attributes: student name major class name course id grade credits Create Course object class (Course.java) Course object should be the following attributes: course id instructor id room id Create testStudent class Output Create a report that has appropriate headings and 1 line of detail for each student record read in. Detail lines should include the following information: student name, class id, Instructor id, room id, grade, credits, comment Read student information, process the information and print an output line for each student record in the ClassesData.txt file. You will also need the CoursesData.txt file for courses information. This is the information included in the ClassesData.txt file 1001Intro. to CompSci 4ALBERT, PETER A. Comp Info System A1001Intro. to CompSci 4ALLENSON, SHEILA M. Comp Info System B1001Intro. to CompSci 4ANDERSON, ALENE T. Comp Info System…arrow_forwardProgram: File GamePurse.h: class GamePurse { // data int purseAmount; public: // public functions GamePurse(int); void Win(int); void Loose(int); int GetAmount(); }; File GamePurse.cpp: #include "GamePurse.h" // constructor initilaizes the purseAmount variable GamePurse::GamePurse(int amount){ purseAmount = amount; } // function definations // add a winning amount to the purseAmount void GamePurse:: Win(int amount){ purseAmount+= amount; } // deduct an amount from the purseAmount. void GamePurse:: Loose(int amount){ purseAmount-= amount; } // return the value of purseAmount. int GamePurse::GetAmount(){ return purseAmount; } File main.cpp: // include necessary header files #include <stdlib.h> #include "GamePurse.h" #include<iostream> #include<time.h> using namespace std; int main(){ // create the object of GamePurse class GamePurse dice(100); int amt=1; // seed the random generator srand(time(0)); // to play the…arrow_forward
- A Mutator function within a class must have access to the private data item but Accessor functions should not. True False Question 32 4 pts The private and public areas of a class can appear in any order. In other words the public declarations or private declarations can come first...it does not matter. True Falsearrow_forwardclass Widget: """A class representing a simple Widget === Instance Attributes (the attributes of this class and their types) === name: the name of this Widget (str) cost: the cost of this Widget (int); cost >= 0 === Sample Usage (to help you understand how this class would be used) === >>> my_widget = Widget('Puzzle', 15) >>> my_widget.name 'Puzzle' >>> my_widget.cost 15 >>> my_widget.is_cheap() False >>> your_widget = Widget("Rubik's Cube", 6) >>> your_widget.name "Rubik's Cube" >>> your_widget.cost 6 >>> your_widget.is_cheap() True """ # Add your methods here if __name__ == '__main__': import doctest # Uncomment the line below if you prefer to test your examples with doctest # doctest.testmod()arrow_forwardExercise 1-Account class • Design a class named Account that contains : • A private int data field named id for the account • A private double data field named balance for the account • A privet Date data field named dateCreated that stores the date when the account was created • A no-arg constructor that creates a default account • A constructor that creates an account with the specified id and initial balance • The getters (i.e., accessors) and setters (i.e., mutators) methods for id and balance • The getter method for dateCreated • A method named withdraw that withdraws a specified amount from the account • A method named deposit that deposits a specified amount to the accountarrow_forward
- A(n) is a unique form of class member that enables an object to store and retrieve data.arrow_forwardObject adapters and class adapters each provide a unique function. These concepts are also significant due to the significance that you attach to them.arrow_forwardCreate a Class called Transaction with: instance variables: stock - your stock class type - char -> b or s (buy/sell) quantity - can be fractional price - buy/sell price when - LocalDate constructors: constructor with parameters for stock, type and quantity. - sets the price from the stock price instance variable - sets when from LocalDate getters and setters for each instance variablearrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
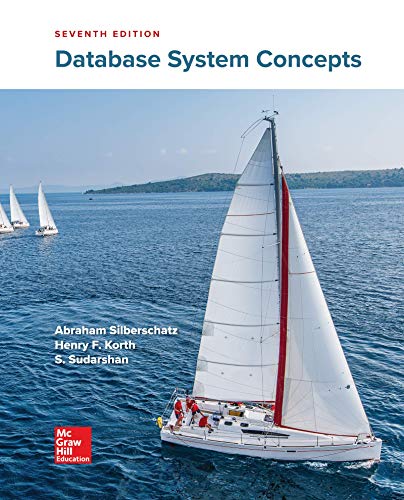
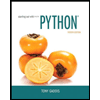
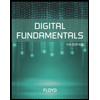
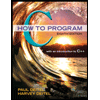
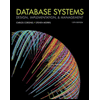
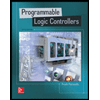