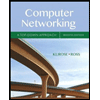
This question was rejected because lack of a text file, which I do not see a way to upload. I saved the first part of the file as an image because that is all I can upload. If that doesn't suffice, please advise how to include a text file.
In C++, I would like some help improving or making a better password lookup function for a Hash Table class/program I made,
The function: it is supposed to lookup a user password using their ID and name which I read in from a text file. The one I have only works for the first 10 records, which I highlighted below in the program.
string lookupPassword (string inUserID, string inUserName){
string thePassword;
...
return thePassword;
}
Here is part of the program thus far:
// Hash.cpp
#include <iostream>
#include<string>
#include<iomanip>
#include<fstream>
using namespace std;
//node class
class nodeBST {
private: //user data
string userID;
string userName;
string userPW;
//BST left and right children
nodeBST* ptrLeftNode;
nodeBST* ptrRightNode;
public:
//constructor
nodeBST() { userID = " "; userName = " "; userPW = " "; ptrLeftNode = NULL; ptrRightNode = NULL; }
//get and set methods for ID, Name, PW
string getUserID() { return userID; }
void setUserID(string inUserID) { userID = inUserID; }
string getUserName() { return userName; }
void setUserName(string inUserName) { userName = inUserName; }
string getUserPW() { return userPW; }
void setUserPW(string inUserPW) { userPW = inUserPW; }
//get and set left and right children pointers
void setPtrLeftNode(nodeBST* inPtrLeftNode) { ptrLeftNode = inPtrLeftNode; }
nodeBST* getPtrLeftNode() { return ptrLeftNode; }
void setPtrRightNode(nodeBST* inPtrRightNode) { ptrRightNode = inPtrRightNode; }
nodeBST* getPtrRightNode() { return ptrRightNode; }
};
class myHash { //hash class
private:
//hash table with chaining - using BST's
nodeBST* myHashTable[10]; //10 BST root node headers
int recordCount = 0; //count initialization
public:
myHash() { //constructor
recordCount = 0;
for (int i = 0; i < 10; i++) { myHashTable[i] = NULL; }
} //loads 100 record text file into hash table
void loadHashTableFromFile(string inFileName) {
string inUserID;
string inUserName;
string inUserPW;
string aWord;
ifstream fin;
cout << "Reading in File for Hash Table" << endl;
fin.open(inFileName); //opens file
for (int i = 0; i < 100; i++) {
if (fin >> aWord) {
if (i % 3 == 0) {
inUserID = aWord;
}
else if (i % 3 == 1) {
inUserName = aWord;
}
else if (i % 3 == 2) {
inUserPW = aWord;
addRecord(inUserID, inUserName, inUserPW);
}
}
}
fin.close(); //closes file
}
string lookupPassword(string inUserId, string inUserName) { //******This is where I need help****
string thePassWord = "Goofy"; //initialize PW temp value
//gather user index
int index = myHashFunction(inUserId);
//root to hold index for user ID
nodeBST* root = myHashTable[index];
//set PW by looking up userPW through root
thePassWord = root->getUserPW();
return thePassWord;
}
int myHashFunction(string inUserID) {
//calculate array index and return index value 0 -9
int index = 0;
char aChar = inUserID[inUserID.length() - 1];
index = aChar - '0';
return index;
}
void addRecord(string inUserID, string inUserName, string inUserPW) {
int index = 0;
//calculate index = ID's last digit
index = myHashFunction(inUserID);
//declare new node and add to BST
nodeBST* newNode = new nodeBST;
//assign values to new node
newNode->setUserID(inUserID);
newNode->setUserName(inUserName);
newNode->setUserPW(inUserPW);
//check if root node occupied
if (myHashTable[index] == NULL) { //add to root
myHashTable[index] = newNode;
}
else {
//add to BST
//find location to add new node
recuriseInsertRecord(myHashTable[index], newNode);
}
}
I have print and traversal functions but I cut them out. Thank You for your time!
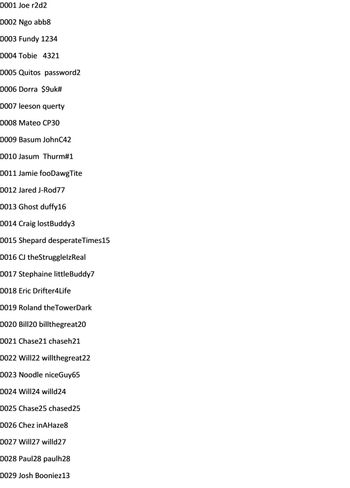

Step by stepSolved in 2 steps

- What are the fundamental operations of a linked list and the main advantage of a linked list over an array? Test program LinkedList.java, and make sure that you understand each operation in the program. (refer to linkedListApplication.java). In this code which part I will keep main.java file and which part I will keep LinkedList.java.java file. Because I will use main.java IDE. Otherwise my program doesn't run. import java.util.*; public class LinkedList{ public Node header; public LinkedList() { header = null; } public final Node Search(int key) { Node current = header; while (current != null && current.item != key) { current = current.link; } return current; } public final void Append(int newItem) { Node newNode = new Node(newItem); newNode.link = header; header = newNode; } public final Node Remove() { Node x = header; if (header != null) {…arrow_forwardPlease answer the question in the screenshot. The language used is Java.arrow_forwardImplement the transaction manager class using java Explain your code in few words. I have included other classes that have relation with the parking transaction calssarrow_forward
- Hi, I am a bit lost on how to do this coding assignment. It would be great if someone could help me fill in the MyStack.java file with the given information. All the information is in the pictures. Thank you!arrow_forwardWrite a program in c++ and make sure it works, that reads a list of students (first names only) from a file. It is possible for the names to be in unsorted order in the file but they have to be placed in sorted order within the linked list.The program should use a doubly linked list.Each node in the doubly linked list should have the student’s name, a pointer to the next student, and a pointer to the previous student. Here is a sample visual. The head points to the beginning of the list. The tail points to the end of the list. When inserting consider all the following conditions:if(!head){ //no other nodes}else if (strcmp(data, head->name)<0){ //smaller than head}else if (strcmp(data, tail->name)>0){ //larger than tail}else{ //somewhere in the middle} When deleting a student consider all the following conditions:student may be at the head, the tail or in the middleBelow, you will find a sample of what the file looks like. Notice the names are in…arrow_forwardI don't get the "//To be implemented" comments, what am I supposed to do?Also, are the header files and header files implementation different? Like is the linkedList.h different than the linkedList.h (implementation)?arrow_forward
- Description: In this project, you are required to make a java code to build a blockchain including multiple blocks. In each block, it contains a list of transactions, previous hash - the digital signature of the previous block, and the hash of the current block which is based on the list of transactions and the previous hash. If anyone would change anything with the previous block, the digital signature of the current block will change. When this changes, the digital signature of the next block will change. Detailed Requirements: 1. Define your block class which contains three private members and four public methods. a. Members: previousHash (int), transactions (String[]), and blockHash (int); b. Methods: i. Block( int previousHash, String[] transactions){} ii. getPreviousHash(){} iii. getTransaction(){} iv. getBlockHash(){} 2. Using ArrayList to design your Blockchain. 3. Your blockchain must contain genesis block and make it to be the first block. 4. You can add a block to the end of…arrow_forwardImplement a city database using a BST to store the database records. Each database record contains the name of the city (a string of arbitrary length) and the coordinates of the city expressed as integer x- and y-coordinates. The BST should be organized by city name. Your database should allow records to be inserted, deleted by name or coordinate, and searched by name or coordinate. Another operation that should be supported is to print all records within a given distance of a specified point.Collect running-time statistics for each operation. Which operations can be implemented reasonably efficiently (i.e., in O(log n) time in the average case) using a BST? Can the database system be made more efficient by using one or more additional BSTs to organize the records by location?arrow_forwardImplement a blog server in C 1. Implement get of all posts (GET /posts), and individual post (GET /post/1) 2. Implement creation of post (POST /posts). Allow the user to enter their name, but check that they are a valid user in db. Do error checking! The user should not specify the post id; that should come from the database. 3. Write three html files to test this; serve them with the blog server: index.html shows the list of posts with a link to each. post.html shows a post. publish.html allows the creation of a new post. Link these together as a user would expect!arrow_forward
- I'm developing a card game that requires one deck of 52 cards using Java. The 52 card has 4 suits from top to bottom: diamonds (d), clubs (c), hearts (h), and spades (s). Each card has a point. What I want is, from the code I've given, I want the program to perform the same but using comparator/comparable instead of hashmaps. The rest of the instructions are in the images just for reference purposes. import java.util.HashMap; import java.io.*;public class CardPointsList {static HashMap<String,Integer> Code = new HashMap<>();static HashMap<Character,Integer> Order = new HashMap<>();static String[][] hand= {{ "c5","s6","sK","dK","dA"},{"s7","s4","dJ","sA","h5"},{"sQ","d3","c9","hK","d5"}};static int[] scores={0,0,0};public static void sortHand() { String temp;for (int h=0;h<3;h++){for (int i=0;i<5;i++){ for (int j=i+1;j<5;j++){ if (hand[h][j].charAt(0) < hand[h][i].charAt(0)){temp = hand[h][j];hand[h][j] = hand[h][i];hand[h][i] = temp;}elseif…arrow_forwardin C++ Write definition of search, isItemAtEqual, retrieve, remove, print, constructor, and destructor for class hashT based upon a method of your choice . Write a test program to test with at least 3 different hashing functions. No PLAGIARISM please . Thank you!arrow_forwardIt says that 'readmatrix' is undefined. Do I need to use dlmread()? My original question was more if I had a file with entries, how do I throw them into a matrix?arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
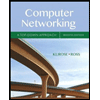
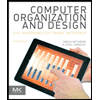
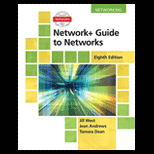
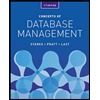
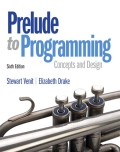
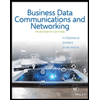