Use the style guide provided in Chapter 2.16 as a starting point and also incorporate these guidelines: • • You can use either brace format (open on same or next line) о Whatever you use – be consistent Use descriptive names (exception - loop variables) 。 Bonus - good naming and style reduces the need for comments Save comments for non-obvious code. Comments go on the line above that code, horizontally aligned o E.g. count = count + 1; doesn't need a comment • • • • Combine declarations and initialization whenever possible Always use the best loop for that type of iteration Use whitespace to separate each section (e.g., classes, methods, imports, variable declarations) – space consistently and eliminate random whitespace. - 。 Space out subtasks logically inside methods – don't clump it all together. Make sure you take the extra time to ensure everything is ready even in the case of a mishap. Failure to follow these procedures will result in a penalty. Sample output Here is an example of what your report should look like. You can vary it as long the temperatures line up vertically and everything is clearly labeled: CIS 231 - Assignment 4 - Your Name Fahr Cels Kelv 30.0 -1.1 272.0 40.0 4.4 277.6 50.0 10.0 283.2 ====== ======= ======= Average: High: 40.0 4.4 277.6 50.0 10.0 283.2 Low: 30.0 -1.1 272.0 Above Average: 1 Equal to Average: 1 Below Average: 1 Standard Deviation: 10.0 This program involves inputting multiple data items (temperatures) that are each within a certain range and then outputting a report about them utilizing arrays and methods. 1. The program will input from 1 to 35 (inclusive) Fahrenheit temperatures from the user. You must first ask them to enter the number of temperatures that they will be typing in. If the value entered is not between 1 and 35, you need to display a message informing the user that the value they entered is out of range and make them re-input until they provide an acceptable value. Prompt every input. 2. The Fahrenheit values are doubles and must range between -150.0 and 350.0 (inclusive.) If an out-of-range temperature is entered, you must display a message informing the user as such and have them re-input until an in-range value is entered. 3. Generate the output specified below. Notes/Specifications: 1. You must create an array to hold your (double) temperature values. 2. All output needs to be right-justified and aligned by the decimal point (see back) 3. Blank lines are only used to separate sections of the report (#5 below, and back.) 4. Display all floating-point (double) numbers to one decimal place. 5. The output must be done in this order: a. The assignment and your name b. The values in ascending order (in Fahrenheit, Celsius, and Kelvin) c. The average temperatures (for Fahrenheit, Celsius, and Kelvin) d. The highest and lowest temperatures (for Fahrenheit, Celsius, and Kelvin) e. The amount of temperatures above/equal to/and below the average (once) f. The standard deviation for Fahrenheit. Use "corrected sample standard deviation" (n-1 divisor): (be sure to address and avoid a potential divide by zero.) 6. Part of your grade is based on how well you break down the problem into smaller components. Each static method should only handle one primary task (computing and outputting data need to be separate methods.) • main() will only be used for crucial variables and calling other (static) methods. There are no instance variables/methods in this program. • NOTE: Taking all code and putting it into a method that's called from main () or other attempt to circumvent this requirement will result in an extremely large deduction. Humongous, in fact. 7. The sort algorithm must be coded using the most optimal implementation provided for that sort (Insertion or Selection) in the Chapter 8 material. 8. All requirements from Assignment 3 (including formatting) are in force for this (and any subsequent) assignment unless specifically amended. 9. Your code must be free of compile and runtime errors. 10. Any variables being accessed in a method must be passed in as arguments and used as parameters. No external access is permitted.
Use the style guide provided in Chapter 2.16 as a starting point and also incorporate these guidelines: • • You can use either brace format (open on same or next line) о Whatever you use – be consistent Use descriptive names (exception - loop variables) 。 Bonus - good naming and style reduces the need for comments Save comments for non-obvious code. Comments go on the line above that code, horizontally aligned o E.g. count = count + 1; doesn't need a comment • • • • Combine declarations and initialization whenever possible Always use the best loop for that type of iteration Use whitespace to separate each section (e.g., classes, methods, imports, variable declarations) – space consistently and eliminate random whitespace. - 。 Space out subtasks logically inside methods – don't clump it all together. Make sure you take the extra time to ensure everything is ready even in the case of a mishap. Failure to follow these procedures will result in a penalty. Sample output Here is an example of what your report should look like. You can vary it as long the temperatures line up vertically and everything is clearly labeled: CIS 231 - Assignment 4 - Your Name Fahr Cels Kelv 30.0 -1.1 272.0 40.0 4.4 277.6 50.0 10.0 283.2 ====== ======= ======= Average: High: 40.0 4.4 277.6 50.0 10.0 283.2 Low: 30.0 -1.1 272.0 Above Average: 1 Equal to Average: 1 Below Average: 1 Standard Deviation: 10.0 This program involves inputting multiple data items (temperatures) that are each within a certain range and then outputting a report about them utilizing arrays and methods. 1. The program will input from 1 to 35 (inclusive) Fahrenheit temperatures from the user. You must first ask them to enter the number of temperatures that they will be typing in. If the value entered is not between 1 and 35, you need to display a message informing the user that the value they entered is out of range and make them re-input until they provide an acceptable value. Prompt every input. 2. The Fahrenheit values are doubles and must range between -150.0 and 350.0 (inclusive.) If an out-of-range temperature is entered, you must display a message informing the user as such and have them re-input until an in-range value is entered. 3. Generate the output specified below. Notes/Specifications: 1. You must create an array to hold your (double) temperature values. 2. All output needs to be right-justified and aligned by the decimal point (see back) 3. Blank lines are only used to separate sections of the report (#5 below, and back.) 4. Display all floating-point (double) numbers to one decimal place. 5. The output must be done in this order: a. The assignment and your name b. The values in ascending order (in Fahrenheit, Celsius, and Kelvin) c. The average temperatures (for Fahrenheit, Celsius, and Kelvin) d. The highest and lowest temperatures (for Fahrenheit, Celsius, and Kelvin) e. The amount of temperatures above/equal to/and below the average (once) f. The standard deviation for Fahrenheit. Use "corrected sample standard deviation" (n-1 divisor): (be sure to address and avoid a potential divide by zero.) 6. Part of your grade is based on how well you break down the problem into smaller components. Each static method should only handle one primary task (computing and outputting data need to be separate methods.) • main() will only be used for crucial variables and calling other (static) methods. There are no instance variables/methods in this program. • NOTE: Taking all code and putting it into a method that's called from main () or other attempt to circumvent this requirement will result in an extremely large deduction. Humongous, in fact. 7. The sort algorithm must be coded using the most optimal implementation provided for that sort (Insertion or Selection) in the Chapter 8 material. 8. All requirements from Assignment 3 (including formatting) are in force for this (and any subsequent) assignment unless specifically amended. 9. Your code must be free of compile and runtime errors. 10. Any variables being accessed in a method must be passed in as arguments and used as parameters. No external access is permitted.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
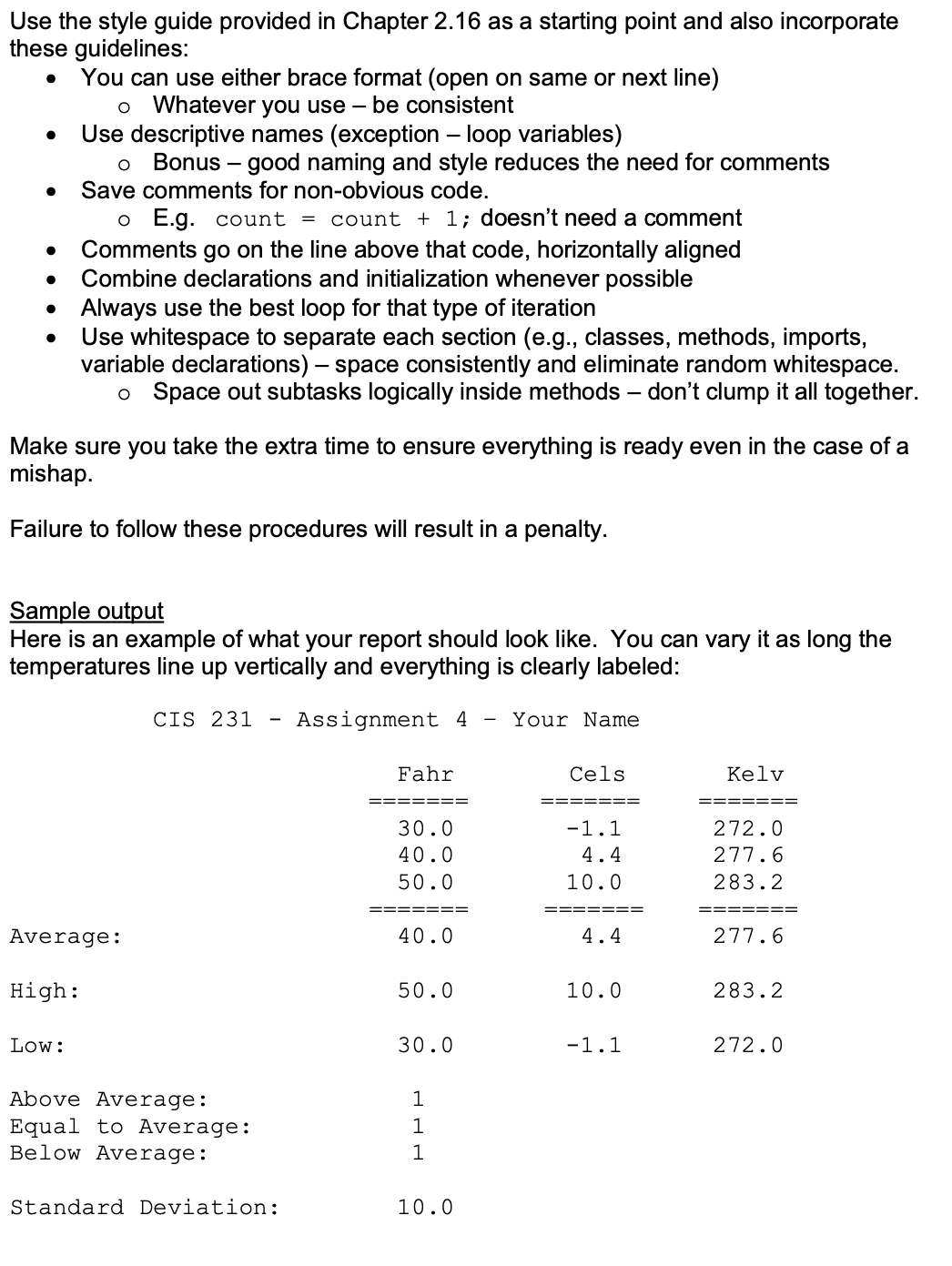
Transcribed Image Text:Use the style guide provided in Chapter 2.16 as a starting point and also incorporate
these guidelines:
•
•
You can use either brace format (open on same or next line)
о Whatever you use – be consistent
Use descriptive names (exception - loop variables)
。 Bonus - good naming and style reduces the need for comments
Save comments for non-obvious code.
Comments go on the line above that code, horizontally aligned
o E.g. count
= count + 1; doesn't need a comment
•
•
•
•
Combine declarations and initialization whenever possible
Always use the best loop for that type of iteration
Use whitespace to separate each section (e.g., classes, methods, imports,
variable declarations) – space consistently and eliminate random whitespace.
-
。 Space out subtasks logically inside methods – don't clump it all together.
Make sure you take the extra time to ensure everything is ready even in the case of a
mishap.
Failure to follow these procedures will result in a penalty.
Sample output
Here is an example of what your report should look like. You can vary it as long the
temperatures line up vertically and everything is clearly labeled:
CIS 231
-
Assignment 4
-
Your Name
Fahr
Cels
Kelv
30.0
-1.1
272.0
40.0
4.4
277.6
50.0
10.0
283.2
======
=======
=======
Average:
High:
40.0
4.4
277.6
50.0
10.0
283.2
Low:
30.0
-1.1
272.0
Above Average:
1
Equal to Average:
1
Below Average:
1
Standard Deviation:
10.0

Transcribed Image Text:This program involves inputting multiple data items (temperatures) that are each within
a certain range and then outputting a report about them utilizing arrays and methods.
1. The program will input from 1 to 35 (inclusive) Fahrenheit temperatures from the
user. You must first ask them to enter the number of temperatures that they will
be typing in. If the value entered is not between 1 and 35, you need to display a
message informing the user that the value they entered is out of range and make
them re-input until they provide an acceptable value. Prompt every input.
2. The Fahrenheit values are doubles and must range between -150.0 and 350.0
(inclusive.) If an out-of-range temperature is entered, you must display a
message informing the user as such and have them re-input until an in-range
value is entered.
3. Generate the output specified below.
Notes/Specifications:
1. You must create an array to hold your (double) temperature values.
2. All output needs to be right-justified and aligned by the decimal point (see back)
3. Blank lines are only used to separate sections of the report (#5 below, and back.)
4. Display all floating-point (double) numbers to one decimal place.
5. The output must be done in this order:
a. The assignment and your name
b. The values in ascending order (in Fahrenheit, Celsius, and Kelvin)
c. The average temperatures (for Fahrenheit, Celsius, and Kelvin)
d. The highest and lowest temperatures (for Fahrenheit, Celsius, and Kelvin)
e. The amount of temperatures above/equal to/and below the average (once)
f. The standard deviation for Fahrenheit. Use "corrected sample standard
deviation" (n-1 divisor):
(be sure to address and avoid a potential divide by zero.)
6. Part of your grade is based on how well you break down the problem into smaller
components. Each static method should only handle one primary task
(computing and outputting data need to be separate methods.)
•
main() will only be used for crucial variables and calling other (static)
methods. There are no instance variables/methods in this program.
• NOTE: Taking all code and putting it into a method that's called from
main () or other attempt to circumvent this requirement will result in an
extremely large deduction. Humongous, in fact.
7. The sort algorithm must be coded using the most optimal implementation
provided for that sort (Insertion or Selection) in the Chapter 8 material.
8. All requirements from Assignment 3 (including formatting) are in force for this
(and any subsequent) assignment unless specifically amended.
9. Your code must be free of compile and runtime errors.
10. Any variables being accessed in a method must be passed in as arguments and
used as parameters. No external access is permitted.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Recommended textbooks for you
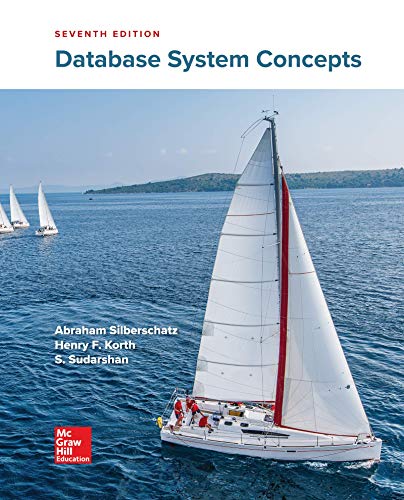
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
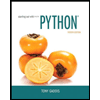
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
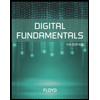
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
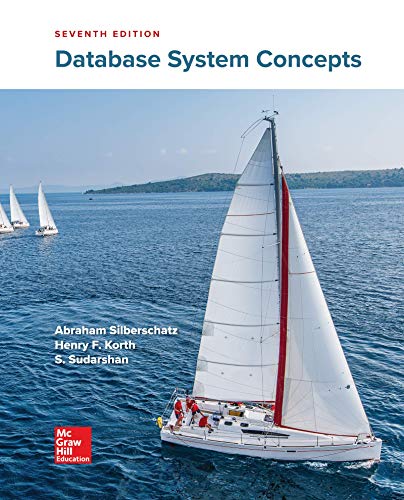
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
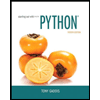
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
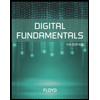
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
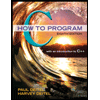
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
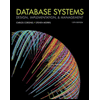
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
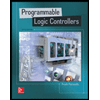
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education