This exercise asks you to define some functions for manipulating linked structures. You should use the Node and TwoWayNode classes, as defined in this chapter. Create a tester module in the testnode.py file that contains your function definitions and your code for testing them. In the testnode.py file, complete the following: Complete the implementation of the length() function (not len). This function expects a singly linked structure as an argument. Returns the number of items in the structure. To test your program run the main() method in the testnode.py file. Your program's output should look like the following:
Types of Linked List
A sequence of data elements connected through links is called a linked list (LL). The elements of a linked list are nodes containing data and a reference to the next node in the list. In a linked list, the elements are stored in a non-contiguous manner and the linear order in maintained by means of a pointer associated with each node in the list which is used to point to the subsequent node in the list.
Linked List
When a set of items is organized sequentially, it is termed as list. Linked list is a list whose order is given by links from one item to the next. It contains a link to the structure containing the next item so we can say that it is a completely different way to represent a list. In linked list, each structure of the list is known as node and it consists of two fields (one for containing the item and other one is for containing the next item address).
This exercise asks you to define some functions for manipulating linked structures. You should use the Node and TwoWayNode classes, as defined in this chapter.
Create a tester module in the testnode.py file that contains your function definitions and your code for testing them.
In the testnode.py file, complete the following:
- Complete the implementation of the length() function (not len).
- This function expects a singly linked structure as an argument.
- Returns the number of items in the structure.
To test your program run the main() method in the testnode.py file.
Your program's output should look like the following:
0: 0 5: 5



Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

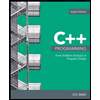
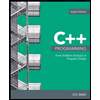