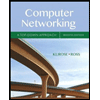
The purpose of this project is to gain a greater understanding of the Intel 32-bit instruction set and understand how a compiler translates C code into assembly language. By compiling the
To compile an unoptimized version use:
gcc -Wa,-adhln -g -masm=intel -m32 "Project 2.c" > "Project 2.asm"
To compile an optimized version use:
gcc -Wa,-adhln -O -masm=intel -m32 "Project 2.c" > "Project 2-o.asm"
The -Wa,-adhln option causes gcc to generate intermixed source and assembly code.
The -masm=intel option causes gcc to generate assembly code in intel format.
The -m32 generates 32-bit code.
The -g option generates unoptimized code while -O generates optimized code.
The generated code includes quite a few directives that can be ignored. Most of the directives begin with a period (.). There are also a few call instructions to procedures that you may not know what they mean that can be ignored. For example:
call __x86.get_pc_thunk.bx
add ebx, OFFSET FLAT:_GLOBAL_OFFSET_TABLE_
The following questions should be answered using the unoptimized code.
In detail explain the code generated for line 82 (k = function1(i, j);). For this and all further questions in which you are asked to explain the code generated in detail, I expect you to copy the generated code and add a comment for each line. For example:
006e C745FC00 mov DWORD PTR -4[ebp], 0 ; initialize i
- In detail explain the code generated for function2 (line 28 through 39).
The following questions should be answered using the optimized code.
- In detail explain the code generated for function1.
- How are function calls optimized?
The following questions should be answered using by comparing the unoptimized code and the optimized code.
- Compare the code generated by function4 in the unoptimized and optimized versions. Explain the optimizations.
C code. Need to optimized and unpotimized versions that show the assembly language.
/* Project 2 */
/* GCC */
/* gcc -Wa,-adhln -g -masm=intel -m32 "Project 2.c" > "Project 2-g.asm" */
/* gcc -Wa,-adhln -O -masm=intel -m32 "Project 2.c" > "Project 2-o.asm" */
#include <stdio.h>
#define NOINLINE __attribute__ ((noinline))
static NOINLINE int function1(int x, int y)
{
int i;
int sum;
int values[10];
sum = 0;
for (i = 0; i < 10; i++) {
values[i] = 10 * i + x * y;
sum += values[i];
}
return (sum);
}
28. static int NOINLINE function2(int *values, int valuesLen)
{
int i;
int sum;
sum = 0;
for (i = 0; i < valuesLen; i++) {
sum += values[i];
}
39. return (sum);
}
static NOINLINE int function3(int x)
{
int y;
y = x / 10;
return (y);
}
static NOINLINE int function4(int a, int b, int c, int d)
{
int r;
if (a > b)
r = a;
else if (a > c)
r = 2 * a;
else if (a > d)
r = 3 * a;
else
r = -1;
return (r);
}
int main(int argc, char **argv)
{
int i;
int j;
int k;
int values[10];
i = 1;
j = 2;
82. k = function1(i, j);
printf("function1: i = %d, j = %d, k = %d\n", i, j, k);
for (i = 0; i < 10; i++) {
values[i] = i;
}
k = function2(values, 10);
printf("function2: k = %d\n", k);
k = function3(100);
printf("function3: k = %d\n", k);
k = function4(1, 2, 3, 4);
printf("function4: k = %d\n", k);
return (0);
}
I have labeled some lines of code

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

In detail explain the code generated for function2 (line 28 through 39).
In detail explain the code generated for function2 (line 28 through 39).
- I like to know how to create an assembly language 80x86 architecture in Lab16A-Adjusted Balances. I am having a hard time figuring out what to start with the program and I need help with how to solve it. Here are the instruction for the assignment and I already upload the pictures of the outrun. Please explains to me how to solve these problems in the assembly language 80x86. Here is an example of how the class write it 80x86 archeictues for assembly language: INCLUDE asmlib.inc .data .code main PROC main ENDP End mainarrow_forwardPython code, the code that I have is wrong. I am getting a error "'relative_error' is inaccurate"arrow_forwardFull explanation of what you did please step by steparrow_forward
- Orthogonality means that an instruction set has a "backup" instruction that can be used instead of any other instruction that does the same thing. It's up to you to prove or disprove what I think.arrow_forwardPLEASE HELParrow_forwardThe portion of the generated assembly code implementing the C function int (int x, int y, int z) is as follows: Based on this assembly code, fill in the missing portions of the C code. Verify your answer using gcc compiler. Note: x in %edi, y in %esi, z in %edx.arrow_forward
- Orthogonality refers to the presence of a "backup" instruction in an instruction set design that may be used in place of any other instruction that achieves the same aim. The onus is on you to confirm or refute my assumption.arrow_forwardE In the following code block(Reference:Q11), you will a set of assembly instructions with corresponding line numbers (line numbers are for informational purpose only and they are not part of the source code). 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 mov edx, 5 dec ecx jmp LABEL1 mov eax, 1 LABEL1: mul edx jmp ecx mov edx, 0678h sub edx, eax jmp DWORD PTR [edx] neg ebx add ecx, ebx mov eax, 0 For each of the conditions/scenario listed below, indicate the corresponding line number (that cause or is associated with the condition/scenario). Enter 0 (Zero) if the condition is not caused by the block of code. 1) Memory indirect jump: type your answer... type your answer... type your answer... type your answer... 2) Register indirect jump: 3) Relative short jump: type your answer... 5) Two's complement type your answer... 4) Relative near jump: 6) Unreachable codearrow_forwardThe inclusion of a "backup" instruction in a design for an instruction set is what is meant by the term "orthogonality." This "backup" instruction may be substituted for any other instruction that accomplishes the same goal. Could you kindly tell me whether or not it is accurate?arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
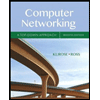
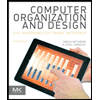
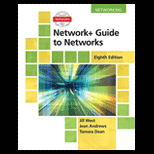
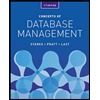
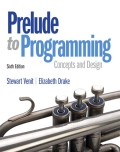
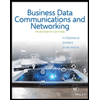