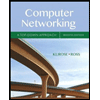
turn this c code into assembly language in optimized and unoptimized mode.
/* Project 2 */
/* GCC */
/* gcc -Wa,-adhln -g -masm=intel -m32 "Project 2.c" > "Project 2-g.asm" */
/* gcc -Wa,-adhln -O -masm=intel -m32 "Project 2.c" > "Project 2-o.asm" */
#include <stdio.h>
#define NOINLINE __attribute__ ((noinline))
static NOINLINE int function1(int x, int y)
{
int i;
int sum;
int values[10];
sum = 0;
for (i = 0; i < 10; i++) {
values[i] = 10 * i + x * y;
sum += values[i];
}
return (sum);
}
static int NOINLINE function2(int *values, int valuesLen)
{
int i;
int sum;
sum = 0;
for (i = 0; i < valuesLen; i++) {
sum += values[i];
}
return (sum);
}
static NOINLINE int function3(int x)
{
int y;
y = x / 10;
return (y);
}
static NOINLINE int function4(int a, int b, int c, int d)
{
int r;
if (a > b)
r = a;
else if (a > c)
r = 2 * a;
else if (a > d)
r = 3 * a;
else
r = -1;
return (r);
}
int main(int argc, char **argv)
{
int i;
int j;
int k;
int values[10];
i = 1;
j = 2;
k = function1(i, j);
printf("function1: i = %d, j = %d, k = %d\n", i, j, k);
for (i = 0; i < 10; i++) {
values[i] = i;
}
k = function2(values, 10);
printf("function2: k = %d\n", k);
k = function3(100);
printf("function3: k = %d\n", k);
k = function4(1, 2, 3, 4);
printf("function4: k = %d\n", k);
return (0);
}

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- 13.write a small assembly languageu program that stores the data 46H and AH into the stack memory addressed by 600H and 602H, then store the first in AX and second in BX 14. Write a simpleo assembly languageu program to divide 90/9 using repeated subtraction?arrow_forwardThe portion of the generated assembly code implementing the C function int (int x, int y, int z) is as follows: Based on this assembly code, fill in the missing portions of the C code. Verify your answer using gcc compiler. Note: x in %edi, y in %esi, z in %edx.arrow_forward1. Writing Assembler Show valid x64 assembly code that implements the following C function. Indicate the calling convention you used: Microsoft or Linux/MacOS int inOrder(int a, int b, int c) { if (a > b) return 0; return b - c; }arrow_forward
- What is the assembly language statement of this machine language code 8B7164H? Select the correct response: MOV SI, [BX + DI + 64H] MOV[BX + DI + 46H], SI MOV[BX + DI + 64H], CX MOV CX, [BX + DI + 46H]arrow_forwardI need to know the meaning of the TEMP EQUs, DVAR EQUs, and FLAG EQU. This is machine language for a microcontroller, it seems a substraction given the HEX OC-4F. I just need a brief descriptionarrow_forwardPlease answer should be in mips assembly languagearrow_forward
- Please help and explain with this problem from microcomputer systems in ARM assembly programmingarrow_forwardE In the following code block(Reference:Q11), you will a set of assembly instructions with corresponding line numbers (line numbers are for informational purpose only and they are not part of the source code). 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 mov edx, 5 dec ecx jmp LABEL1 mov eax, 1 LABEL1: mul edx jmp ecx mov edx, 0678h sub edx, eax jmp DWORD PTR [edx] neg ebx add ecx, ebx mov eax, 0 For each of the conditions/scenario listed below, indicate the corresponding line number (that cause or is associated with the condition/scenario). Enter 0 (Zero) if the condition is not caused by the block of code. 1) Memory indirect jump: type your answer... type your answer... type your answer... type your answer... 2) Register indirect jump: 3) Relative short jump: type your answer... 5) Two's complement type your answer... 4) Relative near jump: 6) Unreachable codearrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
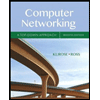
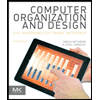
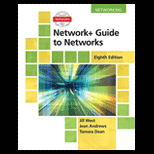
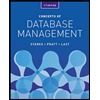
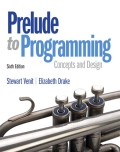
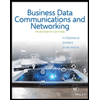