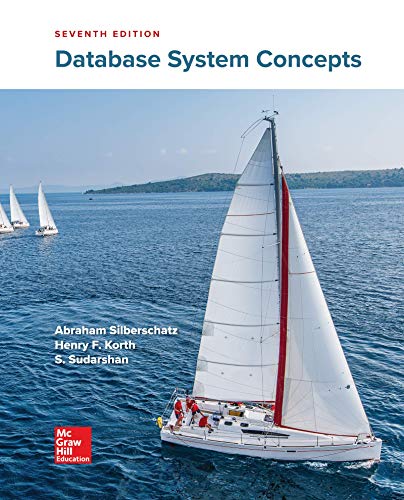
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
![Here is a transcription of the Python code for the `Card` class, typically implemented in a card game system to manage individual playing cards. This code snippet utilizes an order dictionary to assign ranks to different card values and defines a few methods for card representation and comparison.
```python
class Card:
def __init__(self, value, suit):
self.value = value
self.suit = suit
order = {'Ace':1, 'Two':2, 'Three':3, 'Four':4, 'Five':5,
'Six':6, 'Seven':7, 'Eight':8, 'Nine':9, 'Ten':10,
'Jack':11, 'Queen':12, 'King':13}
self.rank = order[self.value]
def __repr__(self):
return self.value + ' of ' + self.suit
def __gt__(self, other):
return self.rank > other.rank and self.suit == 'Hearts'
```
### Explanation:
1. **Initialization (`__init__` method):**
- The class initializes with `value` and `suit` parameters.
- It uses a dictionary called `order` to map each card's face value to a numerical rank.
- The `self.rank` attribute is assigned based on the card's value using this dictionary.
2. **String Representation (`__repr__` method):**
- Defines how the card is represented as a string, displaying the card's value and suit (e.g., "Ace of Spades").
3. **Comparison (`__gt__` method):**
- Provides a way to compare two cards.
- A card is considered greater if it has a higher rank and belongs to the 'Hearts' suit.
Understanding this class allows the implementation of structured and comparable objects representing playing cards in a game.](https://content.bartleby.com/qna-images/question/3fa555a0-fa20-4733-876b-481c70decb77/27a0cedc-f747-448e-90c6-6920534073b4/n589bv_thumbnail.jpeg)
Transcribed Image Text:Here is a transcription of the Python code for the `Card` class, typically implemented in a card game system to manage individual playing cards. This code snippet utilizes an order dictionary to assign ranks to different card values and defines a few methods for card representation and comparison.
```python
class Card:
def __init__(self, value, suit):
self.value = value
self.suit = suit
order = {'Ace':1, 'Two':2, 'Three':3, 'Four':4, 'Five':5,
'Six':6, 'Seven':7, 'Eight':8, 'Nine':9, 'Ten':10,
'Jack':11, 'Queen':12, 'King':13}
self.rank = order[self.value]
def __repr__(self):
return self.value + ' of ' + self.suit
def __gt__(self, other):
return self.rank > other.rank and self.suit == 'Hearts'
```
### Explanation:
1. **Initialization (`__init__` method):**
- The class initializes with `value` and `suit` parameters.
- It uses a dictionary called `order` to map each card's face value to a numerical rank.
- The `self.rank` attribute is assigned based on the card's value using this dictionary.
2. **String Representation (`__repr__` method):**
- Defines how the card is represented as a string, displaying the card's value and suit (e.g., "Ace of Spades").
3. **Comparison (`__gt__` method):**
- Provides a way to compare two cards.
- A card is considered greater if it has a higher rank and belongs to the 'Hearts' suit.
Understanding this class allows the implementation of structured and comparable objects representing playing cards in a game.

Transcribed Image Text:**Card Class Attributes**
**Prompt**: List all of the instance variables (also known as attributes) of the Card class.
---
This text is a prompt indicating that students should identify and list the attributes associated with a "Card" class. In object-oriented programming, a class can have multiple attributes that define the characteristics or data it maintains. Students might expect typical attributes like:
- **Suit**: Represents the suit of the card (e.g., hearts, diamonds).
- **Rank**: Represents the rank or value of the card (e.g., ace, king).
- **Color**: Optional attribute indicating card color (e.g., red, black).
Students are expected to recognize and list these based on either a provided class definition or their understanding of card-related programming concepts.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Lab 2 – Designing a class This lab requires you to think about the steps that take place in a program by writing pseudocode. Read the following program prior to completing the lab. Design a class named Computer that holds the make, model, and amount of memory of a computer. Include methods to set the values for each data field, and include a method that displays all the values for each field. For the programming problem, create the pseudocode that defines the class and enter it below. Enter pseudocode herearrow_forwardclass Product: def __init__(self, product_id, name, price, quantity): self.product_id = product_id self.name = name self.price = round(price, 2) self.quantity = quantity def get_product_description(self): return (f"Product ID: {self.product_id}, " f"Name: {self.name}, " f"Price: ${self.price:.2f}, " f"Quantity Available: {self.quantity}") class ElectronicProduct(Product): def __init__(self, product_id, name, price, quantity,warranty_period): self.warranty_period = warranty_period super().__init__(product_id,name,price,quantity) def get_product_description(self): base_description = super().get_product_description() return base_description + f"\nWarranty: {self.warranty_period}" class ClothingProduct(Product): def __init__(self, product_id, name, price, quantity,size): self.size = size…arrow_forward2. Car Class Write a class named car that has the following fields: ▪ yearModel. The year Model field is an int that holds the car's year model. ▪ make. The make field is a String object that holds the make of the car, such as "Ford", "Chevrolet", "Honda", etc. ▪ speed. The speed field is an int that holds the car's current speed. In addition, the class should have the following methods. ■ Constructor. The constructor should accept the car's year model and make as arguments. These values should be assigned to the object's year Model and make fields. The constructor should also assign 0 to the speed field.arrow_forward
- class Information: def __init__(self, name, address, age, phone_number): self.__name = name self.__address = address self.__age = age self.__phone_number = phone_number def main(): my_info = Information('John Doe','111 My Street', \ '555-555-1281')arrow_forward#include <iostream>using namespace std;class Player{private:int id;static int next_id;public:int getID() { return id; }Player() { id = next_id++; }};int Player::next_id = 1;int main(){Player p1;Player p2;Player p3;cout << p1.getID() << " ";cout << p2.getID() << " ";cout << p3.getID();return 0;} Run the program and give its output.arrow_forwardIn Python: Write a class named Pet, which should have the following data attributes: _ _name (for the name of a pet) _ _animal_type (for the type of animal that a pet is. Example values are 'Dog','Cat', and 'Bird') _ _age (for the pets age) The Pet class should have an _ _init_ _ method that creates these attributes. It should also have the following methods: set_nameThis method assigns a value to the _ _name field set_animal_typeThis method assigns a value to the _ _animal_type field set_ageThis method assignsa value to the _ _age field get_nameThis method assignsa value to the _ _name field get_animal_typeThis method assignsa value to the _ _animal_type field get_ageThis method assignsa value to the _ _age field Once you have written the class, write a program that creates an object of the class and prompts the user to enter the name, type and age of his or her pet. This data should be stored as the objects attributes. Use the objects accessor methods to retrieve the pets…arrow_forward
- class Duration: def __init__(self, hours, minutes): self.hours = hours self.minutes = minutes def __add__(self, other): total_hours = self.hours + other.hours total_minutes = self.minutes + other.minutes if total_minutes >= 60: total_hours += 1 total_minutes -= 60 return Duration(total_hours, total_minutes) first_trip = Duration(2, 58)second_trip = Duration(0, 49) first_time = first_trip + second_tripsecond_time = second_trip + second_trip print(first_time.hours, first_time.minutes)print(second_time.hours, second_time.minutes) what is the entire outputarrow_forwardAssuming that we have defined the Card class as above, fill in the blank in the code below such that the code segment prints out "Yes" without causing any errors. if > Card( 'Queen', 'Spades'): print("Yes")arrow_forwardUse java languagearrow_forward
- class Duration: def __init__(self, hours, minutes): self.hours = hours self.minutes = minutes def __add__(self, other): total_hours = self.hours + other.hours total_minutes = self.minutes + other.minutes if total_minutes >= 60: total_hours += 1 total_minutes -= 60 return Duration(total_hours, total_minutes) first_trip = Duration(3, 36)second_trip = Duration(0, 47) first_time = first_trip + second_tripsecond_time = second_trip + second_trip print(first_time.hours, first_time.minutes) what is the outputarrow_forward#include <iostream>using namespace std;class Player{private:int id;static int next_id;public:int getID() { return id; }Player() { id = next_id++; }};int Player::next_id = 1;int main(){Player p1;Player p2;Player p3;cout << p1.getID() << " ";cout << p2.getID() << " ";cout << p3.getID();return 0;} Run the program and give its output.arrow_forward#include <iostream>using namespace std;class Player{private:int id;static int next_id;public:int getID() { return id; }Player() { id = next_id++; }};int Player::next_id = 1;int main(){Player p1;Player p2;Player p3;cout << p1.getID() << " ";cout << p2.getID() << " ";cout << p3.getID();return 0;} Run the program and give its output.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
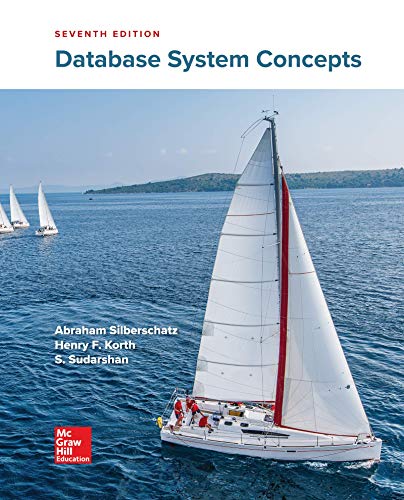
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
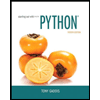
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
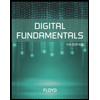
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
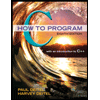
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
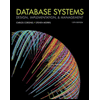
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
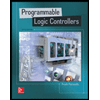
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education