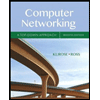
Q#:In a population, the birth rate and death rate are calculated as follows:
Birth Rate = Number of Births ÷ Population
Death Rate = Number of Deaths ÷ Population
For example, in a population of 100,000 that has 8,000 births and 6,000 deaths per year,
Birth Rate = 8,000 ÷ 100,000 = 0.08
Death Rate = 6,000 ÷ 100,000 = 0.06
Design a Population class that stores a current population, annual number of births, and annual number of deaths for some geographic area. The class should allow these three values to be set in two ways: either by passing arguments to a three-parameter constructor when a new Population object is created or by calling the setPopulation, setBirths, and setDeaths class member functions. Alternatively, you can use public properties of C#. The class should also have getBirthRate and getDeathRate functions that compute and return the birth and death rates. Write a short program that uses the Population class and illustrates its capabilities.

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- Write UML Class Diagram that illustrates the following program: 1) Person class The person class should include the following data fields; • Name • ID number • birth date • home address • phone number Be sure to include get and set methods for each data field. 2) Course class The course class should contain the following fields: • ID Number • Name College (Business, Engineering, Nursing, etc) • An Array of person objects . 3) University class The University class should contain the data fields for the university, such as: • University name • Federal ID number • Address • Array / ArrayList of course class objects • Array / ArrayList of person class objects Extended Classes: 4 & 5) Extend the Person class into Student class and Employee class 6) Extend the Employee class into Instructor class and Staff class 7 & 8) Extend the Student class into graduate Student and undergrad Student class 9) Registration Class ---> The Registration class creates transactions to link each student with…arrow_forwardclass Book: book_belongs_to = 'Schulich School of Engineering' def _init_(self, pages = 0, title = 'Unknown', author = 'Unknown', isbn = self.pages = pages self.title = title e): self.author = author self.isbn = isbn book1 = Book(255, 'Black Beauty', 'Anna Sewell', 9780001840423) book2 = Book(208, 'The Chrysalids', 'John Wyndham', 9780140013085) Book.book_belongs_to = 'Emily Marasco' book3 = Book()arrow_forwardQuestion 31 class Person: def __init__(mysillyobject, name, age): = name mysillyobject.name mysillyobject.age = age def myfunc(abc): print("Hello my age is", abc.age) p1 = Person("John", 36) p1.age = 40 p1.myfunc() O 40 Hello my age is 40 36 Hello my age is 36arrow_forward
- 6. Patient Charges Write a class named Patient that has attributes for the following data: • First name, middle name, and last name • Address, city, state, and ZIP code • Phone number ● The Patient class's init__ method should accept an argument for each attribute. The Name and phone number of emergency contact Patient class should also have accessor and mutator methods for each attribute. Next, write a class named Procedure that represents a medical procedure that has been performed on a patient. The Procedure class should have attributes for the following data: • Name of the procedure • Date of the procedure • Name of the practitioner who performed the procedure • Charges for the procedure 107 The Procedure class's __init__ method should accept an argument for each attribute. The Procedure class should also have accessor and mutator methods for each attribute Next, write a program that creates an instance of the Patient class, initialized with sample data. Then, create three…arrow_forwardTwo data members: feet(int) and inches(float) Two overloaded constructors: one that takes no argument and the other that takes two arguments. Zero argument constructor initializes data members from 0 whereas two argument constructor initializes data members from the passed arguments. Two getters and two setters corresponding to two data members. A member function named toDecimal that converts a distance to decimal, e.g., if a distance equals 2 feet and 6 inches then it would become 2.5 in decimal. A member function named toString that returns a string representation of a distance, e.g., if a distance equals 2 feet and 6 inches then the corresponding string representation should be 2’-6”. by using C++arrow_forwardCIST 1305 UNIT 10 DROP BOX ASSIGNMENT Create the class diagram and write the pseudocode for a class named Computer that holds the make, model, and amount of memory of a computer. Include methods to set the values for each data field, and include a method that displays all the values for each field.arrow_forward
- N-SIDED REGULAR POLYGON) In an n-sided regular polygon, all sides have the same length and all angles have the same degree (i.e., the polygon is both equilateral and equiangular). Design a class named RegularPolygon that contains: ▪ A private int data field named n that defines the number of sides in the polygon with default value 3. ▪ A private double data field named side that stores the length of the side with default value 1. A private double data field named x that defines the x-coordinate of the polygon's center with default value 0. ▪ A private double data field named y that defines the y-coordinate of the polygon's center with default value 0. A no-arg constructor that creates a regular polygon with default values. A constructor that creates a regular polygon with the specified number of sides and length of side, centered at (0, 0). A constructor that creates a regular polygon with the specified number of sides, length of side, and x- and y-coordinates. ▪ The accessor and…arrow_forwardDesign a struct with following members: p_num_lots, int* type pa_lots, int [] type (dynamically allocated) Implement following methods: parameterized constructor which takes an int for num of lots copy constructor copy assignment operator destructorarrow_forwardPointer and classImplement a class called Team as specified:data members:name - the name of the Team (defined as a dynamic variable)members - a dynamic array of stringsize - number of members in the team.functions:Course(): default constructor set name to “TBD”, and size to 0, members toempty listCourse(string): one argument constructor set name, and size to 0, members toempty listaccessor - an accessor for the name and size variablemutator - an mutator for the name variableUse following main() to test your class.int main() {Team a,b("Mets");cout<<a.getName()<<endl; // print TBDa.setName("Yankee");cout<<a.getName()<<endl; // print Yankeecout<<b.getName()<<endl; // print Metscout<<a.getSize()<<endl; // print 0return 0;}arrow_forward
- "CommissionEmployee" class: package commissions; import employees.Employee; public class CommissionEmployee extends Employee { private double gross_sales; private double rate; public CommissionEmployee(String fname, String lname, String SSN, double gross_sales, double rate) { super(fname, lname, SSN); this.gross_sales = gross_sales; this.rate = rate; } public double getGross_sales() { return gross_sales; } public void setGross_sales(double gross_sales) { this.gross_sales = gross_sales; } public double getRate() { return rate; } public void setRate(double rate) { this.rate = rate; } public double earnings() { return gross_sales*rate; } @Override public String toString() { return super.toString()+"[gross_sales=" + gross_sales + ", rate=" + rate + "]"; } } Update the code in JAVA to include the following: "BasePlusCommissionEmployee" class that inherits from "CommissionEmployee" class. A BasePlusCommissionEmployee has a…arrow_forwardPersonal Information Class: Design a class called Employee that holds the following data about an employee: name ID number Department Job Title Class. Store your class in a separate file called employee.py. Your class will have an initializer method that will be passed the information entered by the user as arguments. Write appropriate accessor and mutator methods for each data attribute. Write a __str__ method to print the contents of the class (see example of __str__ on p. 523). Main program: Your main program should create three instances of the class. Your program should get the information from the user and pass it as parameters to the initializer method. Using the __str__ method invoked by the print function, the program should display the personal information for the three individuals. Output and Sample Dialog: Enter employee name: Mary Smith Enter employee ID: 123456 Enter department: Accounting Enter position: Accountant Enter employee name: Joe Morales Enter…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
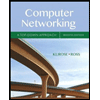
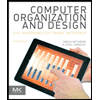
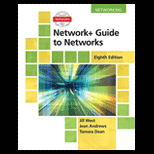
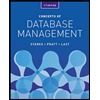
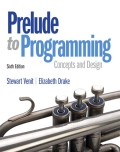
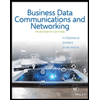