the following code is centered around data collection, filtering, and using the Singleton pattern in Java, which is all part of a mockup "student information management system", with its purpose being making mockup new student accounts. I've been having issues using the software “dbeaver” for making java projects. Please organize the below code into an executable project “simulation.student” and given step by step instruction as to how this was done Address.java public class Address { private String _city; private String _state; private String _streetName; // New private int _streetNumber; // New private int _apartmentNumber; // New public Address(String city, String state, int zip, String streetName, int streetNumber, int apartmentNumber) { setCity(city); setState(state); setZip(zip); setStreetName(streetName); setStreetNumber(streetNumber); setApartmentNumber(apartmentNumber); } public String getCity() { return _city; } public void setCity(String city) { _city = city; } public String getState() { return _state; } public void setState(String state) { _state = state; } public int getZip() { return _zip; } public void setZip(int zip) { _zip = zip; } public String getStreetName() { return _streetName; } public void setStreetName(String streetName) { _streetName = streetName; } public int getStreetNumber() { return _streetNumber; } public void setStreetNumber(int streetNumber) { _streetNumber = streetNumber; } public int getApartmentNumber() { return _apartmentNumber; } public void setApartmentNumber(int apartmentNumber) { _apartmentNumber = apartmentNumber; } } Student.java public abstract class Student { private long Id; private String _lastName, _firstName; protected Address _stuAddress; protected double tuition; protected StudentClassification eStudentClass; public Student(String firstName, String lastName, StudentClassification eStudent, Address stuAddress) { setId(); if ((!firstName.equals("")) && (firstName.matches("^[-a-zA-Z\\s]*$"))) { setFirstName(firstName); } else { setFirstName("INVALID FIRST NAME"); } setLastName(lastName); setClassification(eStudent); _stuAddress = stuAddress; } private void setId() { Id = StudentIdGenerator.getNextId(); } public long getId() { return Id; } public String getLastName() { return _lastName; } public void setLastName(String pName) { _lastName = pName; } public String getFirstName() { return _firstName; } public void setFirstName(String pName) { _firstName = pName; } public double getTuition() { return tuition; } public StudentClassification getClassification() { return eStudentClass; } public abstract void setTuition(); @Override public String toString() { StringBuilder studentInfo = new StringBuilder("Name: " + getFirstName() + " " + getLastName() + "\n"); studentInfo.append("ID: " + getId() + " Tuition: " + getTuition() + "\n"); studentInfo.append("Address: " + _stuAddress.getCity() + ", " + _stuAddress.getState()); studentInfo.append(" " + _stuAddress.getZip()); studentInfo.append(" " + _stuAddress.getStreetName() + ", " + _stuAddress.getStreetNumber()); studentInfo.append(", Apt " + _stuAddress.getApartmentNumber()); return studentInfo.toString(); } } StudentDemo3.java import java.util.ArrayList; public class StudentDemo3 { public static void main(String[] args) { ArrayList students = new ArrayList<>(); StringBuilder studentOutput = new StringBuilder(""); // Use the setStartingID() method in 'StudentIdGenerator' Singleton class to start at 500 StudentIdGenerator.setStartingID(500); students.add(new UndergraduateStudent("Harry Muggle", "Potter", StudentClassification.FRESHMAN, new Address("Atlanta", "Georgia", 30305, "Main St", 123, 101))); students.add(new UndergraduateStudent("Diana-Wonder-Woman", "Prince", StudentClassification.JUNIOR, new Address("Washington D.C.", "District of Columbia", 30318, "Broadway Ave", 456, 202))); // Add two GraduateStudent objects to the 'students' collection (ArrayList) students.add(new GraduateStudent("John", "Doe", StudentClassification.MASTERS, new Address("New York", "New York", 10001, "5th Ave", 789, 303))); students.add(new GraduateStudent("Jane", "Smith", StudentClassification.DOCTORATE, new Address("Los Angeles", "California", 90001, "Sunset Blvd", 987, 505))); // Loop to print student information for (Student s : students) { studentOutput.append("\n-----------------------------\n").append(s.toString()); if (s instanceof UndergraduateStudent) { studentOutput.append("\nUndergraduate student of status: ").append(s.getClassification().toString()); } else if (s instanceof GraduateStudent) { studentOutput.append("\nGraduate student of status: ").append(s.getClassification().toString()); } System.out.println(studentOutput.toString() + "\n"); studentOutput.setLength(0); } } } please run the code such that it has the appropriate expected outcome of ----------------------------- Name: Harry Muggle Potter ID: 500 Tuition: 4000.0 Address: St. Louis, Georgia 30305 Undergraduate student of status: FRESHMAN -----------------------------
the following code is centered around data collection, filtering, and using the Singleton pattern in Java, which is all part of a mockup "student
Address.java
public class Address {
private String _city;
private String _state;
private String _streetName; // New
private int _streetNumber; // New
private int _apartmentNumber; // New
public Address(String city, String state, int zip, String streetName, int streetNumber, int apartmentNumber) {
setCity(city);
setState(state);
setZip(zip);
setStreetName(streetName);
setStreetNumber(streetNumber);
setApartmentNumber(apartmentNumber);
}
public String getCity() {
return _city;
}
public void setCity(String city) {
_city = city;
}
public String getState() {
return _state;
}
public void setState(String state) {
_state = state;
}
public int getZip() {
return _zip;
}
public void setZip(int zip) {
_zip = zip;
}
public String getStreetName() {
return _streetName;
}
public void setStreetName(String streetName) {
_streetName = streetName;
}
public int getStreetNumber() {
return _streetNumber;
}
public void setStreetNumber(int streetNumber) {
_streetNumber = streetNumber;
}
public int getApartmentNumber() {
return _apartmentNumber;
}
public void setApartmentNumber(int apartmentNumber) {
_apartmentNumber = apartmentNumber;
}
}
Student.java
public abstract class Student {
private long Id;
private String _lastName, _firstName;
protected Address _stuAddress;
protected double tuition;
protected StudentClassification eStudentClass;
public Student(String firstName, String lastName, StudentClassification eStudent, Address stuAddress) {
setId();
if ((!firstName.equals("")) && (firstName.matches("^[-a-zA-Z\\s]*$"))) {
setFirstName(firstName);
} else {
setFirstName("INVALID FIRST NAME");
}
setLastName(lastName);
setClassification(eStudent);
_stuAddress = stuAddress;
}
private void setId() {
Id = StudentIdGenerator.getNextId();
}
public long getId() {
return Id;
}
public String getLastName() {
return _lastName;
}
public void setLastName(String pName) {
_lastName = pName;
}
public String getFirstName() {
return _firstName;
}
public void setFirstName(String pName) {
_firstName = pName;
}
public double getTuition() {
return tuition;
}
public StudentClassification getClassification() {
return eStudentClass;
}
public abstract void setTuition();
@Override
public String toString() {
StringBuilder studentInfo = new StringBuilder("Name: " + getFirstName() + " " + getLastName() + "\n");
studentInfo.append("ID: " + getId() + " Tuition: " + getTuition() + "\n");
studentInfo.append("Address: " + _stuAddress.getCity() + ", " + _stuAddress.getState());
studentInfo.append(" " + _stuAddress.getZip());
studentInfo.append(" " + _stuAddress.getStreetName() + ", " + _stuAddress.getStreetNumber());
studentInfo.append(", Apt " + _stuAddress.getApartmentNumber());
return studentInfo.toString();
}
}
StudentDemo3.java
import java.util.ArrayList;
public class StudentDemo3 {
public static void main(String[] args) {
ArrayList<Student> students = new ArrayList<>();
StringBuilder studentOutput = new StringBuilder("");
// Use the setStartingID() method in 'StudentIdGenerator' Singleton class to start at 500
StudentIdGenerator.setStartingID(500);
students.add(new UndergraduateStudent("Harry Muggle", "Potter", StudentClassification.FRESHMAN,
new Address("Atlanta", "Georgia", 30305, "Main St", 123, 101)));
students.add(new UndergraduateStudent("Diana-Wonder-Woman", "Prince", StudentClassification.JUNIOR,
new Address("Washington D.C.", "District of Columbia", 30318, "Broadway Ave", 456, 202)));
// Add two GraduateStudent objects to the 'students' collection (ArrayList)
students.add(new GraduateStudent("John", "Doe", StudentClassification.MASTERS,
new Address("New York", "New York", 10001, "5th Ave", 789, 303)));
students.add(new GraduateStudent("Jane", "Smith", StudentClassification.DOCTORATE,
new Address("Los Angeles", "California", 90001, "Sunset Blvd", 987, 505)));
// Loop to print student information
for (Student s : students) {
studentOutput.append("\n-----------------------------\n").append(s.toString());
if (s instanceof UndergraduateStudent) {
studentOutput.append("\nUndergraduate student of status: ").append(s.getClassification().toString());
} else if (s instanceof GraduateStudent) {
studentOutput.append("\nGraduate student of status: ").append(s.getClassification().toString());
}
System.out.println(studentOutput.toString() + "\n");
studentOutput.setLength(0);
}
}
}
please run the code such that it has the appropriate expected outcome of
-----------------------------
Name: Harry Muggle Potter
ID: 500 Tuition: 4000.0
Address: St. Louis, Georgia 30305
Undergraduate student of status: FRESHMAN
-----------------------------



Algorithm: Student Information Management System
1. Create an empty ArrayList to store student objects.
2. Create a StringBuilder to build the student information output.
3. Set the starting ID for student generation using the StudentIdGenerator Singleton.
4. Create four student objects:
a. Create an UndergraduateStudent object "Harry Potter" with the following details:
- Name: Harry Potter
- Student Classification: FRESHMAN
- Address: Atlanta, Georgia, 30305, Main St, 123, Apt 101
b. Create an UndergraduateStudent object "Diana-Wonder-Woman Prince" with the following details:
- Name: Diana-Wonder-Woman Prince
- Student Classification: JUNIOR
- Address: Washington D.C., District of Columbia, 30318, Broadway Ave, 456, Apt 202
c. Create a GraduateStudent object "John Doe" with the following details:
- Name: John Doe
- Student Classification: MASTERS
- Address: New York, New York, 10001, 5th Ave, 789, Apt 303
d. Create a GraduateStudent object "Jane Smith" with the following details:
- Name: Jane Smith
- Student Classification: DOCTORATE
- Address: Los Angeles, California, 90001, Sunset Blvd, 987, Apt 505
5. Loop through each student in the ArrayList:
a. Build student information as follows:
- Name
- ID
- Tuition
- Address (City, State, Zip, Street, Street Number, Apartment Number)
- Student Classification (Undergraduate or Graduate)
b. Print the student information.
6. End the loop.
7. End the program.
Step by step
Solved in 5 steps with 8 images

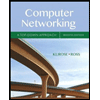
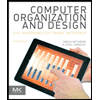
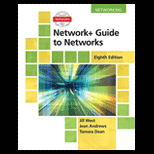
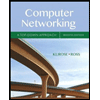
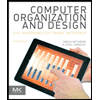
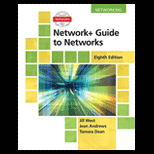
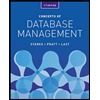
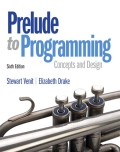
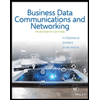