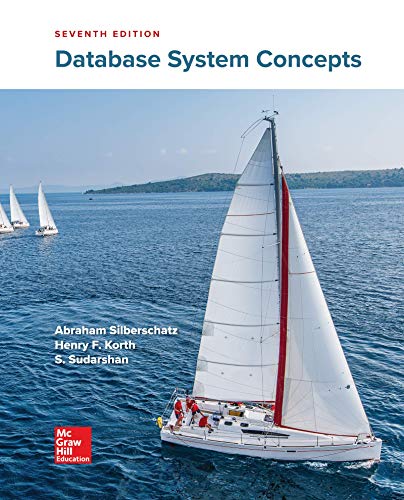
That's enough for you!
def first_preceded_by_smaller(items, k=1):Find and return the 0irst element of the given list of items that is preceded by at least k smaller elements in the list. These required k smaller elements can be positioned anywhere before the current element, not necessarily consecutively immediately before that element. If no element satisfying this requirement exists in the list, this function should return None.
Since the only operation performed for the individual items is their order comparison, and especially no arithmetic occurs at any point during execution, this function should work for lists of any types of elements, as long as those elements are pairwise comparable with each other.
items |
k |
Expected result |
[4, 4, 5, 6] |
2 |
5 |
[42, 99, 16, 55, 7, 32, 17, 18, 73]
|
3 |
18 |
[42, 99, 16, 55, 7, 32, 17, 18, 73]
|
8 |
None |
['bob', 'carol', 'tina', 'alex', 'jack', 'emmy', 'tammy', 'sam', 'ted']
|
4 |
'tammy' |
[9, 8, 7, 6, 5, 4, 3, 2, 1, 10]
|
1 |
10 |
[42, 99, 17, 3, 12]
|
2 |
None |

Step by stepSolved in 3 steps with 1 images

- IntSet unionWith(const IntSet& otherIntSet) const; //Unions the current set with the passed set. IntSet::unionWith(const IntSet& otherIntSet){ for (int i = 0; i < otherIntSet.used; ++i) //For each element in the passed set. { add(otherIntSet.data[i]); //Add it to current set. }}arrow_forwarddef print_categories(main_list): for i in all_categories: main_list = all_categories.split() print(i,":",all_categories[i]) return "" """ Given a list of lists, for each list stored in main_list, output its contents as follows: f"{index of category}. {item[0]} - {item[1]}%". note that indexing must start at 1 for the first item, not 0, which is first item's actual index in main_list. If `main_list` is empty, the function prints "There are no categories." Returns the number of categories.arrow_forwardThis function finds the minimum number in a list. What should be replaced with in order for this function to operate as expected? function min(numList){ numList[0]; for(var i=0; i return min; numList[i] = min; min = numList[i]; min numList; numList min; i Het Which ofthese fu nction s deos this thearrow_forward
- Question 44 Computer Science A list of elements has a size of 100. Choose the operations where an ArrayList would be faster than a LinkedList. (Select all that apply) Question 5 options: removing from index 99 inserting at index 1 removing from index 4 inserting at index 4 Full explain this question and text typing work onlyarrow_forwardstruct insert_at_back_of_sll { // Function takes a constant Book as a parameter, inserts that book at the // back of a singly linked list, and returns nothing. void operator()(const Book& book) { /// TO-DO (3) /// // Write the lines of code to insert "book" at the back of "my_sll". Since // the SLL has no size() function and no tail pointer, you must walk the // list looking for the last node. // // HINT: Do not attempt to insert after "my_sll.end()". // ///// END-T0-DO (3) ||||// } std::forward_list& my_sll; };arrow_forwardAlert dont submit AI generated answer. please explain in details. Please print fixlist function so it would print out both lists a = [1, 5, 3, 6] b = [3, 5, 3 ,2, 4, 6, 8, 5, 2] def printList(list): for i in range(9): print(list[i]) print("End of List" print list(a) printList(b) Expected output: 1 5 3 6 End of List 3 5 3 2 4 6 8 5 2arrow_forward
- True/False Select true or false for the statements below. Explain your answers if you like to receive partial credit. 3) Which of the following is true about the insertBeforeCurrent function for a CircularLinked List (CLL) like you did in programming exercise 1?a. If the CLL is empty, you need to create the new node, set it to current, andhave its next pointer refer to itselfb. The worst case performance of the function is O(n)c. If you insert a new element with the same data value as the current node, theperformance improves to O(log n)arrow_forwarddef reverse_list (1st: List [Any], start: int, end: int) -> None: """Reverse the order of the items in list , between the indexes and (including the item at the index). If start or end are out of the list bounds, then raise an IndexError. The function must be implemented **recursively**. >>> lst ['2', '2', 'v', 'e'] >>>reverse_list (lst, 0, 3) >>> lst ['e', 'v', 'i', '2'] >>> lst [] >>>reverse_list (lst, 0, 0) >>> lst [0] >>> Ist = [] >>> reverse_list (lst, 0, 1) Traceback (most recent call last): IndexError #1 #1arrow_forwardCreate a user-defined function called duplicates. This function will check to see if a singly linked list contains nodes with the same data stored. If any duplicates are found, return the value 1. Otherwise return 0. You may use the following typedef structure. typedef struct node_s{ int data; struct node_s * nextptr; }node_t;arrow_forward
- In the description of bubble sort in the previous question, the sorted section of the list was at the end of the list. In this question, bubble sort will maintain the sorted section of the beginning of the list. Make sure that you are still implementing bubble sort! a) Rewrite the English description of bubble sort from the previous question with the necessary changes so that the sorted elements are at the beginning of the list instead of the end. b) Using your English description of bubble sort, write an outline of the bubble sort algorithm in English. c) Write the bubble_sort_2(L) function. d) Write Nose test cases for bubble_sort_2.arrow_forwardQuestion in image Please explain how to do with the answer. Thank you.arrow_forwardComplete the doctring.def average_daily_temp(high_temps: List[int], low_temps: List[int]) -> List[float]: """high_temps and low_temps are daily high and low temperatures for a series of days. Return a new list of temperatures where each item is the daily average. Precondition: len(high_temps) == len(low_temps) >>> average_daily_temp([26, 27, 27, 28, 27, 26], [20, 20, 20, 20, 21, 21]) [23.0, 23.5, 23.5, 24.0, 24.0, 23.5] """arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
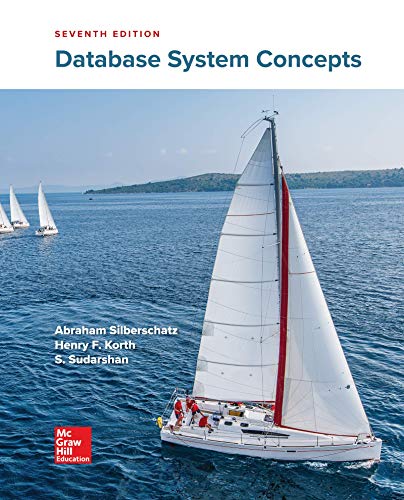
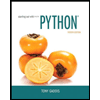
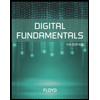
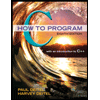
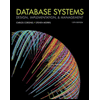
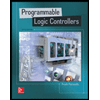