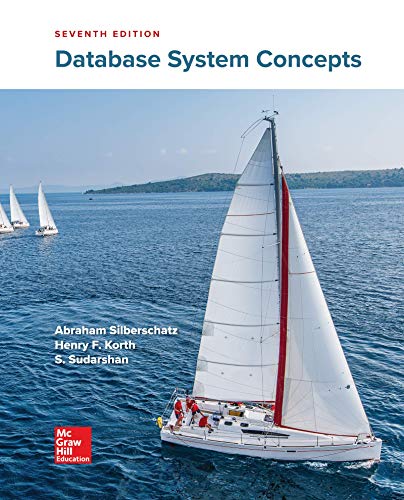
Suppose we add a fixed amount of money into our bank account at the beginning of every year. Modify the
import java.util.Scanner;
/**
This program computes the time required to double an investment
with an annual contribution.
*/
public class DoubleInvestment
{
public static void main(String[] args)
{
final double RATE = 5;
final double INITIAL_BALANCE = 10000;
final double TARGET = 2 * INITIAL_BALANCE;
Scanner in = new Scanner(System.in);
System.out.print("Annual contribution: ");
double contribution = in.nextDouble();
double balance = INITIAL_BALANCE;
int year = 0;
// TODO: Add annual contribution, but not in year 0
while(balance< TARGET) {
year++;
double interest = balance*(RATE/100);
balance = (balance+contribution+interest);
}
System.out.println("Year: " + year);
System.out.printf("Balance: %.2f%n", balance);
}
}

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- import java.util.Scanner;/** This program calculates the geometric and harmonic progression for a number entered by the user.*/public class Progression{ public static void main(String[] args) { Scanner keyboard = new Scanner (System.in); System.out.println("This program will calculate " + "the geometric and harmonic " + "progression for the number " + "you enter."); System.out.print("Enter an integer that is " + "greater than or equal to 1: "); int input = keyboard.nextInt(); // Match the method calls with the methods you write int geomAnswer = geometricRecursive(input); double harmAnswer = harmonicRecursive(input); System.out.println("Using recursion:"); System.out.println("The geometric progression of " + input + " is " + geomAnswer); System.out.println("The harmonic progression of " +…arrow_forwardusing System; class Program { publicstaticvoid Main(string[] args) { int number = 1; while (number <= 88) { Console.WriteLine(number); number = number + 2; } Random r = new Random(); int randNo= r.Next(1,88); Console.WriteLine("Random Number between 1 and 88 is "+randNo); } } how would this program be modified to choose three random numbers instead of just one number between 1-88?arrow_forwardStringFun.java import java.util.Scanner; // Needed for the Scanner class 2 3 /** Add a class comment and @tags 4 5 */ 6 7 public class StringFun { /** * @param args not used 8 9 10 11 12 public static void main(String[] args) { Scanner in = new Scanner(System.in); System.out.print("Please enter your first name: "); 13 14 15 16 17 18 System.out.print("Please enter your last name: "); 19 20 21 //Output the welcome message with name 22 23 24 //Output the length of the name 25 26 27 //Output the username 28 29 30 //Output the initials 31 32 33 //Find and output the first name with switched characters 34 //All Done! } } 35 36 37arrow_forward
- Besides main() this program has one method that computes the amount of raise and the new salary for an employee. The current salary and a performance rating (a String: "Excellent", "Good" or "Poor") are input. import java.util.Scanner; public class Salary { public static void main (String[] args) { Declare variables Prompt for and read current salary and rating For an excellent rating compute raise as 0.06 of current salary For a good rating compute raise as 0.04 of current salary For a poor rating compute raise as 0.015 of current salary You can use abc.equals(“xyz”) to verify the rating read For an invalid rating display an appropriate message and display the salary without change; otherwise, compute and print new salary and raise amount main() must display the message } } Note:- Please type and execute this java program and also need an output for this java program as soon as possible.arrow_forwardJava Questions - (Has 2 Parts). Based on each code, which answer out of the choices "A, B, C, D, E" is correct. Each question has one correct answer. Thank you. Part 1 - Given the following code, the output is __. class Sample{ public static void main (String[] args){ try{ System.out.println(5/0);}catch(ArithmeticException e){ System.out.print("Divide by zero????");}finally{ System.out.println("5/0"); } }} A. Divide by zero????B. 5/0C. Divide by zero????5/0D. 5/0Divide by zero????E. "5/0" Part 2 - Given the following code, the output is __. try{ Integer number = new Integer("1"); System.out.println("An Integer instance.");}catch (Exception e){ System.out.println("An Exception.");} A. An Integer instance.B. An Exception.C. 1D. Error: ExceptionE. None of the optionsarrow_forwardJAVA Language: Transpose Rotate. Question: Modify Transpose.encode() so that it uses a rotation instead of a reversal. That is, a word like “hello” should be encoded as “ohell” with a rotation of one character. (Hint: use a loop to append the letters into a new string) import java.util.*; public class TestTranspose { public static void main(String[] args) { String plain = "this is the secret message"; // Here's the message Transpose transpose = new Transpose(); String secret = transpose.encrypt(plain); System.out.println("\n ********* Transpose Cipher Encryption *********"); System.out.println("PlainText: " + plain); // Display the results System.out.println("Encrypted: " + secret); System.out.println("Decrypted: " + transpose.decrypt(secret));// Decrypt } } abstract class Cipher { public String encrypt(String s) { StringBuffer result = new…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
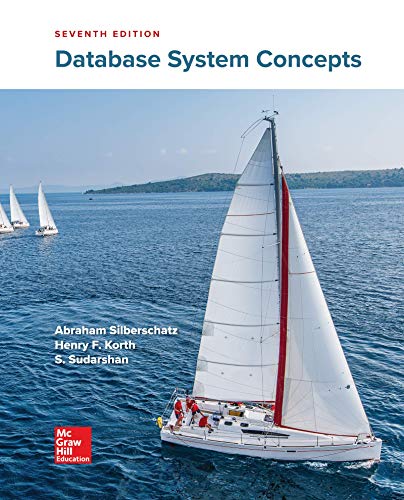
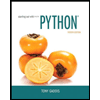
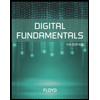
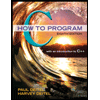
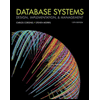
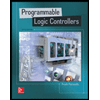