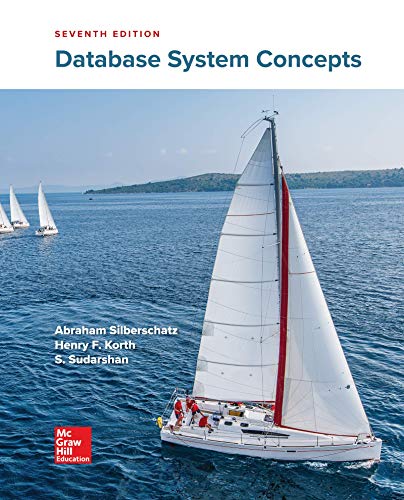
Suppose that you want to implement an
Write an algorithm in pseudocode (following the style of the Minimax pseudocode) that will always make an optimal decision given the knowledge we have about DeepGreen. You are free to use the library function DeepGreenMove(S) in your pseudocode. What advantage would this algorithm have over Minimax? (if none, Justify).

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- You are given an array of integers nums and an integer target. Return the indices of two numbers in the array such that they add up to target. You may assume that each input would have exactly one solution, and you may not use the same element twice. Implement a function in Java to solve this problem using a hash map, and explain the time and space complexity of your solution.arrow_forwardThe sieve of Eratosthenes is a way of computing all the prime numbers below a certain number. (A prime number is a number that is only divisible by itself and 1). Implement this algorithm: Implement a function cross_out_multiples that takes as arguments a list of boolean values (true/false) called is_prime and a number n. The function sets the boolean values at all multiples of n (2*n, 3*n, 4*n ...) that are in the list to false. Implement a function sieve(n) which gives back a list of all primes below n. Put your code in sieve.py This program is tested via unit tests.arrow_forwardIn this problem you will implement a function called triangle_countwhich will take as input a graph object G, representing an undirectedgraph G, and will return the number of triangles in G. Do not use anyimports for this problem. To simplify the problem you may assume thateach edge is stored twice in G. That is if an edge goes from u to v then vwill be in u’s collection and u will be in v’s collection. If you would prefer,you may assume that an edge is only stored once. In either case G willneed to be regarded as undirected.arrow_forward
- solve in C please. Implement the following two functions that get a string, and compute an array of non-emptytokens of the string containing only lower-case letters. For example:● For a string "abc EFaG hi", the list of tokens with only lower-case letters is ["abc", "hi"].● For a string "ab 12 ef hi ", the list of such tokens is ["ab","ef","hi"].● For a string "abc 12EFG hi ", the list of such tokens is ["abc","hi"].● For a string " abc ", the list of such tokens is ["abc"].● For a string "+*abc!! B" the list of such tokens is empty.That is, we break the string using the spaces as delimiters (ascii value 32), and look only at thetokens with lower-case letters only .1. The function count_tokens gets a string str, and returns the number ofsuch tokens.int count_tokens(const char* str);For example● count_tokens("abc EFaG hi") needs to return 2.● count_tokens("ab 12 ef hi") needs to return 3.● count_tokens("ab12ef+") needs to return 0.2. The function get_tokens gets a string str, and…arrow_forwardGiven a string s which consists of lowercase or uppercase letters, return the length of the longest palindrome that can be built with those letters. Letters are case sensitive, for example, "Aa" is not considered a palindrome here. Constraints: 1 <= s.length <= 2000 s consists of lowercase and/or uppercase English letters only. Function definition for Java: public int longestPalindrome(String s) { } Function definition for Python: def longestPalindrome(self, s): #Your code here Announced Test Cases: Example 1: Input: s = "abccccdd" Output: 7 Explanation: One longest palindrome that can be built is "dccaccd", whose length is 7. Example 2: Input: s = "speediskey"Output: 5Explanation: The longest palindrome that can be built is "seees", whose length is 5.arrow_forwardJava languagearrow_forward
- write python program 10x10 grid, agent top right corner, traversing terrain = 1 time unit, rough terrain = 5 time units, and others terain = cannot be reached An A* based search function that takes a list of lists as argument and generates as a result a list of grid cell coordinates starting at the upper right and ending at the lower left and representing the fastest path for the agent to travel. E.g., path = aStarSearch(map). The path can be empty if there is no route.arrow_forwardWithout importing any Lib:arrow_forwardImplement the following function that returns a vector of vectors of Item, each of which is a subset of elements chosen from the given vector a[first…last]: /* if a is {1,2,3}, first=0, last=2, the function shall returns a vector of the following vectors: {}, {1}, {2}, {3}, {1,2},{1,3}, {2,3},{1,2,3}, all subsets of a[0…2]. Precondition: last-first+1>=1, i.e., there is at least one element in the a[first…last] Note 1)if the length of a[first…last] is n, then the function should return a vector of 2n vectors 2) The order of these subsets does not need to match what’s listed here… */ vector<vector<int>> subsets (const vector<int> & a, int first, int last) codearrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
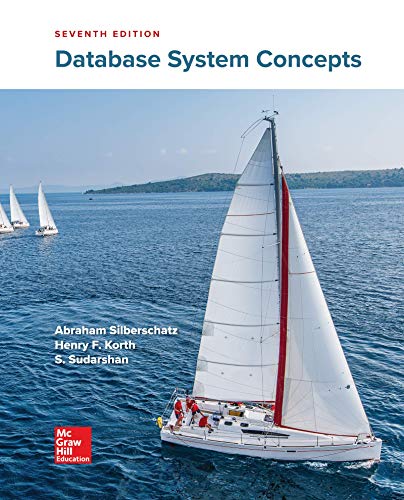
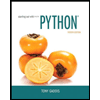
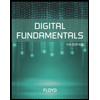
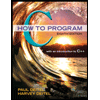
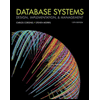
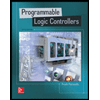