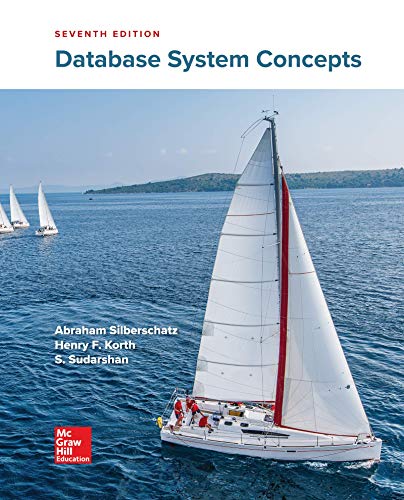
Concept explainers
Implement the following function that returns a vector of
/* if a is {1,2,3}, first=0, last=2, the function shall returns a vector of the following vectors:
{}, {1}, {2}, {3}, {1,2},{1,3}, {2,3},{1,2,3}, all subsets of a[0…2].
Precondition: last-first+1>=1, i.e., there is at least one element in the a[first…last]
Note 1)if the length of a[first…last] is n, then the function should return a vector of 2n vectors
2) The order of these subsets does not need to match what’s listed here…
*/
vector<vector<int>> subsets (const vector<int> & a, int first, int last)
code

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

this didnt answer the question at all
this didnt answer the question at all
- Given an array of integers, find the maximum sum of a contiguous subarray. Write a function called maxSubarraySum that takes an array of integers as input and returns the maximum sum of a contiguous subarray. Example: Input: [1, -3, 2, 1, -1] Output: 3 Explanation: The subarray with the maximum sum is [2, 1], and the sum is 3. You need to implement the maxSubarraySum function. You can use any programming language of your choice.arrow_forward6) While there is a built-in pop_back() method for vectors, there is no built-in pop_front method. Suppose a program needs a pop_front() method that will remove the first element from the vector. For example if the original vector is [1, 2, 3, 4, 5], then after passing in this vector to pop_front() the new resulting vector will be [2, 3, 4, 5]. Which of the options below is the correct implementation of pop_front()? Group of answer choices D-) void pop_front(vector<int> &v){ for(int i=0; i<(v.size()-1); i++)v[i+1] = v[i]; v.pop_back(); } C-) void pop_front(vector<int> &v){ for(int i=0; i<(v.size()-1); i++)v[i] = v[i+1]; v.pop_back(); } B-) void pop_front(vector<int> &v){ for(int i=0; i<v.size(); i++)v[i+1] = v[i]; v.pop_back(); } A-) void pop_front(vector<int> &v){ for(int i=0; i<v.size(); i++)v[i] = v[i+1]; v.pop_back(); } 7) Suppose a program has the following vector: [99, 23, 55, 71, 87, 64, 35, 42] The goal is to…arrow_forwardUse iterators to add up all of the numbers in a vector<double>. #include <vector>using namespace std; double accumulate(const vector<double>& v){ (...) }arrow_forward
- Write a function named addStars that accepts as a parameter a reference to a vector of strings, and modifies the vector by placing a "*" element between elements, as well as at the start and end of the vector. For example, if the vector v contains {"the", "quick", "brown", "fox"}, the call of addStars(v); should modify it to store {"*", "the", "*", "quick", "*", "brown", "*", "fox", "*"}.arrow_forwardTo compute the average of the squares of the elements of a vector V, aka the mean square, we (Note: use randn to create a random vector) Select one: a. We use polyfit which does least squares and skip the minimization part b.we use sum(V.^2)./length(V*V) c.we use sum(V.*V)/length(V) d.we use mean(V) e.we use sum(V^2)/length(V)arrow_forwardFinding the Minimum of a Vector Write a function to return the index of the minimum value in a vector, or -1 if the vector doesn't have any elements. In this problem you will implement the IndexOfMinimumElement function in minimum.cc and then call that function from main.cc. You do not need to edit minimum.h. Index of minimum value with std::vector Complete IndexOfMinimumElement in minimum.cc. This function takes a std::vector containing doubles as input and should return the index of the smallest value in the vector, or -1 if there are no elements. Complete main.cc Your main function asks the user how many elements they want, construct a vector, then prompts the user for each element and fills in the vector with values. Your only task in main is to call the IndexOfMinimumElement function declared in minimum.h, and print out the minimum element's index. Here's an example of how this might look: How many elements? 3 Element 0: 7 Element 1: -4 Element 2: 2 The minimum value in your…arrow_forward
- An array is positive dominant if it contains strictly more unique positive values than unique negative values. Write a function that returns true if an array is positive dominant. Examples ispositiveDominant([1, 1, 1, 1, -3, -4]) // There is only 1 unique positive value (1). // There are 2 unique negative values (-3, -4). ispositiveDominant([5, 99, 832, -3, -4]) → true ispositiveDominant([5, 0]) → true ispositive Dominant([0, -4, -1]) → falsearrow_forwardWrite a function to find the maximum sum of a subarray within a given array of integers. The subarray should be contiguous, meaning the elements are adjacent to each other in the array. For example, given the array [-2, 1, -3, 4, -1, 2, 1, -5, 4], the maximum sum of a subarray is 6, which corresponds to the subarray [4, -1, 2, 1]. Write a function named `maxSubarraySum` that takes an array of integers as input and returns the maximum sum of a subarray. Note: If all the elements in the array are negative, the function should return O. Example: Input: [-2, 1, -3, 4, -1, 2, 1, -5, 4] Output: 6 Input: [-1, -2, -3, -4] Output: 0 Write the 'maxSubarraySum` function that solves this problem efficiently.arrow_forwardCreate a function append(v, a, n) that adds to the end of vector v a copy of all the elements of array a. For example, if v contains 1,2, 3, and a contains 4, 5, 6, then v will end up containing 1, 2, 3, 4, 5,6. The argument n is the size of the array. The argument v is a vector ofintegers and a is an array of integers. Write a test driver.arrow_forward
- Integers are read from input and stored into a vector until 0 is read. If the vector's last element is odd, output the odd elements in the vector. Otherwise, output the even elements in the vector. End each number with a newline. Ex: If the input is -9 12 -6 1 0, the vector's last element is 1. Thus, the output is: -9 1 Note: (x % 2 != 0) returns true if x is odd. int value; int i; bool isodd; 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 } cin >> value; while (value != 0) { } for (i = 0; i > value; Check return 0; 1 Next level 2 X 1: Compare output 3 4 For input -9 12 -6 1 0, the vector elements are -9, 12, -6, and 1. The last element, 1, is odd. The odd elements in the vector, -9 and 1, are output, each on a new line. Not all tests passed. 2 V 3arrow_forwardGiven a budget (type long) and a list of prices represented as entries of a container of type vector<long>, find out how many items on the price_list you can afford at most. This is the return value of the function. Here is its prototype: int get_most_items(std::vector<long>price_list, long budget) To solve this homework, it is a good idea to first sort the price_list in ascending order. For sorting in ascending order, you can use the function std::sort(). It can be called as std::sort(v.begin(), v.end()) .Note that there may be items on the price list whose prices are negative to make the problem a bit more interesting. If the size of price_list is zero, the function returns 0. All necessary libraries have been included. All numbers are expressed in Cents to avoid rounding errors. Here is a simple example:std::vector<long> price_list = { 10, 199, 1}; long budget = 11; int no_items = get_most_items(price_list, budget);The variable no_items will then have the…arrow_forwardHi, OCaml programming 1. Create a type slidingTile, consisting of a char matrix and two integers xand y. The integers x and y are the coordinates of an empty tile. 2. Create a function val slide : slidingTile -> int -> int -> slidingTile = <fun> that given a slidingTile and two integers, that represent a location in thematrix, it slides the tile from the specified location to the empty tile, thenreturns the altered slidingTile. If provided location is not adjacent to theempty tile, or out of bounds, then return the slidingTile unaltered. 3. Create a function val print_tile : slidingTile -> unit = <fun> that prints a slidingTile on screen with the corresponding characters fromthe matrix. However, print an empty space where the empty tile is instead.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
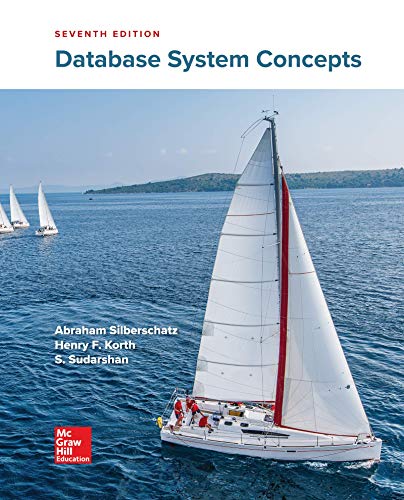
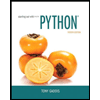
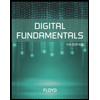
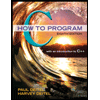
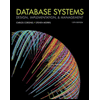
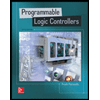