Write a for loop to print all elements in courseGrades, following each element with a space (including the last). Print forwards, then backwards. End each loop with a newline. Ex: If courseGrades = {7, 9, 11, 10}, print: 7 9 11 10 10 11 9 7 Hint: Use two for loops. Second loop starts with i = courseGrades.length - 1. import java.util.Scanner; public class CourseGradePrinter {public static void main (String [ ] args) {Scanner scnr = new Scanner(System.in);final int NUM_VALS = 4;int [ ] courseGrades = new int[NUM_VALS];int i; for (i = 0; i < courseGrades.length; ++i) {courseGrades[i] = scnr.nextInt();} /* Your solution goes here */ }}
Write a for loop to print all elements in courseGrades, following each element with a space (including the last). Print forwards, then backwards. End each loop with a newline. Ex: If courseGrades = {7, 9, 11, 10}, print: 7 9 11 10 10 11 9 7 Hint: Use two for loops. Second loop starts with i = courseGrades.length - 1. import java.util.Scanner; public class CourseGradePrinter {public static void main (String [ ] args) {Scanner scnr = new Scanner(System.in);final int NUM_VALS = 4;int [ ] courseGrades = new int[NUM_VALS];int i; for (i = 0; i < courseGrades.length; ++i) {courseGrades[i] = scnr.nextInt();} /* Your solution goes here */ }}
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Write a for loop to print all elements in courseGrades, following each element with a space (including the last). Print forwards, then backwards. End each loop with a newline. Ex: If courseGrades = {7, 9, 11, 10}, print:
7 9 11 10
10 11 9 7
Hint: Use two for loops. Second loop starts
with i = courseGrades.length - 1.
import java.util.Scanner;
public class CourseGradePrinter {
public static void main (String [ ] args) {
Scanner scnr = new Scanner(System.in);
final int NUM_VALS = 4;
int [ ] courseGrades = new int[NUM_VALS];
int i;
for (i = 0; i < courseGrades.length; ++i) {
courseGrades[i] = scnr.nextInt();
}
/* Your solution goes here */
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
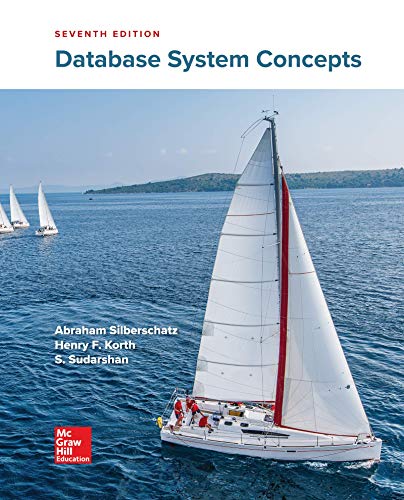
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
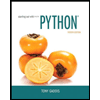
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
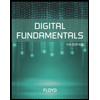
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
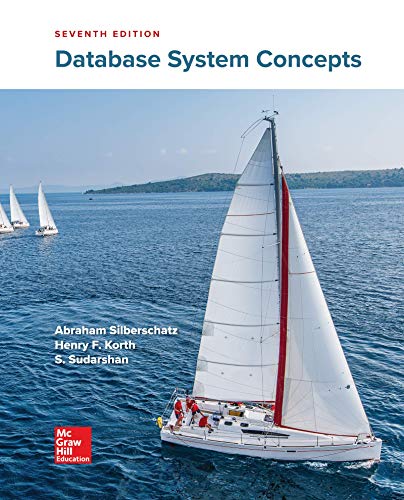
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
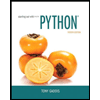
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
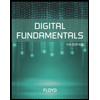
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
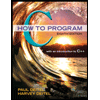
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
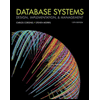
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
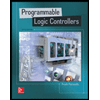
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education