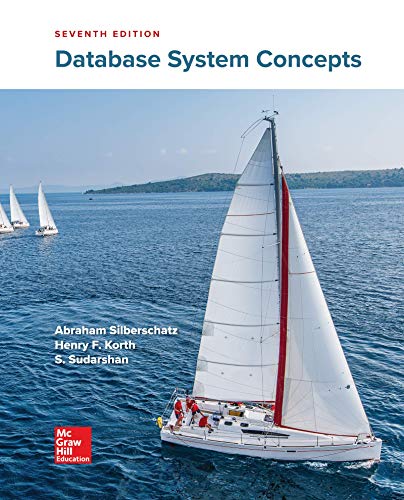
Solved in Java
Write a method maxMagnitude() with two integer input parameters that returns the largest magnitude value. Use the method in a program that takes two integer inputs, and outputs the largest magnitude value.
Ex: If the inputs are:
5 7
the method returns:
7
Ex: If the inputs are:
-8 -2
the method returns:
-8
Note: The method does not just return the largest value, which for -8 -2 would be -2. Though not necessary, you may use the absolute-value built-in math method.
Your program must define and call a method:
public static int maxMagnitude(int userVal1, int userVal2)
import java.util.Scanner;
public class LabProgram {
/* Define your method here */
public static void main(String[] args) {
/* Type your code here */
}
}

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 2 images

- Complete the convert() method that casts the parameter from a double to an integer and returns the result.Note that the main() method prints out the returned value of the convert() method. Ex: If the double value is 19.9, then the output is: 19 Ex: If the double value is 3.1, then the output is: 3 code: public class LabProgram { public static int convert(double d){ /* Type your code here */ } public static void main(String[] args) { System.out.println(convert(19.9)); System.out.println(convert(3.1)); }}arrow_forwardFor the code in java below it shows a deck of 52 cards and asks the name of the two players and makes both players draw five cards from the deck. What I want to be added into the code is that both Players are people that can select what card they pick and Player A starts. Player A picks a card in his/her hand. Player B gets to choose the two cards which add to the value of Player A’s card, if player B lies and the two cards they choose do not add up to A's card, player B loses a point, if player B does not have two cards whose value adds to the value of Player A’s card, then no one gets a point. Player’s A card (if selected) and the two cards from Player B are discarded both players draw back to 5 cards from the deck. Main class code: import java.util.ArrayList; import java.util.Scanner; import java.util.List; import java.util.Random; class Main { publicstaticvoid main(String[] args) { // card game, two players, take turns. String[] suits = {"Hearts", "Clubs", "Spades",…arrow_forwardJava Scriptarrow_forward
- Write a value returning method that accepts two integer values as arguments and returns the value that is the greater of the two. For example, if 6 and 10 are passed as arguments to the method, the method should return 10. Your program should firstly prompt the user to enter two integers, and display the value that is the greater of the two. using Java programmingarrow_forwardFind the error of the following method definition: public static void timesTwo( int x, int y) { int sum=x + y; return sum; }arrow_forwardUnderstanding ifStatements Summary In this lab, you complete a prewritten Java program for a carpenter who creates personalized house signs. The program is supposed to compute the price of any sign a customer orders, based on the following facts: The charge for all signs is a minimum of $35.00. The first five letters or numbers are included in the minimum charge; there is a $4 charge for each additional character. If the sign is made of oak, add $20.00. No charge is added for pine. Black or white characters are included in the minimum charge; there is an additional $15 charge for gold-leaf lettering. Instructions 1. Ensure the file named HouseSign.java is open. 2. You need to declare variables for the following, and initialize them where specified: A variable for the cost of the sign initialized to 0.00 (charge). A variable for the number of characters initialized to 8 (numChars). A variable for the color of the characters initialized to "gold" (color). A variable for the…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
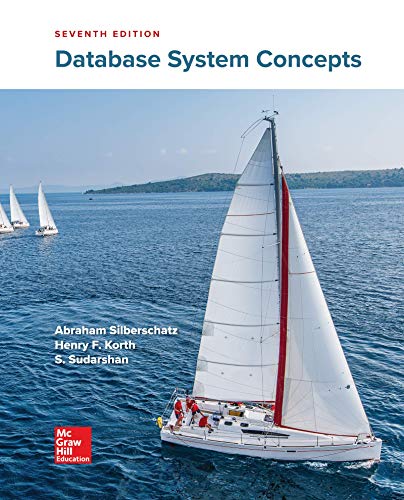
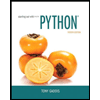
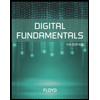
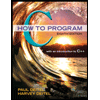
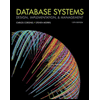
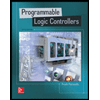