sk 3: Properties (Assignment) You have to write a program for an estate agency. The program must manage renting of properties. Your solution must implement the concepts of encapsulation and inheritance. Information required on all the properties listed to be rented: Type of property (residential (R) or business (B)), name of the owner, contact number of owner, duration of the contract (in months). Residential: Type of property (Flat, Townhouse or House), number of bedrooms, number of bathrooms, number of garages, rent per month. Business: Size in square meters, insurance per month, rent per month. Two separate reports must be displayed for the two types of properties. The output for residential properties must be displayed as follows: Owner Contact number Months Type BedR BathR Rent(pm)
Task 3: Properties (Assignment)
You have to write a program for an estate agency. The program must manage renting of properties. Your solution must implement the
concepts of encapsulation and inheritance.
Information required on all the properties listed to be rented: Type of property (residential (R) or business (B)), name of the owner, contact
number of owner, duration of the contract (in months).
Residential: Type of property (Flat, Townhouse or House), number of bedrooms, number of bathrooms, number of garages, rent per month.
Business: Size in square meters, insurance per month, rent per month.
Two separate reports must be displayed for the two types of properties. The output for residential properties must be displayed as follows:
Owner Contact number Months Type BedR BathR Rent(pm)
Joe Bloom 0879678223 24 T 4 3 R19000
The rent for residential properties depend on the type of property and the number of bedrooms.
Flat – R8000pm + R 500pm per bedroom
Townhouse – R 15000pm + R 1000pm per bedroom
House – R 12000pm + R800pm per bedroom
The output for business properties must be displayed as follows:
Owner Contact number Months Size Insurance(pm) Rent(pm)
Sue Smith 0723456872 12 280 R7000.00 R70000.00
The rent for business properties is calculated based on the size of the property. The rent per square meter is R250.00
1. Write the superclass
Write a superclass Property.
a) Identify the common properties for a property and declare them
as private instance fields.
b) Constructors:
No arguments constructor (also called the default constructor)
Parameterized constructor.
c) Methods:
- Accessor methods (getters) for the instance fields.
- A toString() method to return a string that will neatly display
all the information to describes the object. Example:
Joe Bloom 0879678223 2
2. Write a subclass called ResProperty that inherits from the Property superclass
a) Declare local instance field(s).
b) Two constructors:
No arguments constructor
Parameterized constructor:
- Remember: Call the constructor of the super class using the super keyword.
c) Methods:
-Accessor method for the instance field
-calcMonthRent() method to calculate and return the monthly rent.
-The toString() method must return a string that will neatly display all the information to describes the object.
NOTE: Call the toString method from the super class. Then add salary and the allowance as the next two items to display.
Example:
Joe Bloom 0879678223 24 T 4 3 R19000
3. Write the subclass called BussProperty that inherits from the Property superclass
a)Declare the local instance fields.
b)Two constructors:
No arguments constructor
Parameterized constructor:
- Remember: Call the constructor of the super class using the super keyword.
c)Methods:
-Accessor methods for the instance fields.
- A method calcMonthRent() to calculate and return the monthly rent.
- A method calcMonthInsurance() to calculate and return the monthly insurance amount.
-A toString() method must return a string that will neatly display all the information to describes the object.
NOTE: Call the toString method from the super class..
Example:
Sue Smith 0723456872 12 280 R7000.00 R70000.0
4. Write a testProperties class:
Declare two ArrayLists – one for each type of property –arrResProp and arrBussProp
Create a FileClass class and write a method to read information from a text file called properties.txt into the two ArrayLists.
If the property is a residential property, create the object and add the object to the arrResProp ArrayList. If it is a business property, add the object to the arrBussProp ArrayList.
Example of the content of the text file:
R#Joe Bloom#0879678223#24#T#4#3
R#Sam Xaba#0849558202#12#F#2#1
B#Sue Smith#0723456872#12#280
R#Lee West#0826458288#12#H#3#2
B#John Pule#0723456872#24#320
B#Abe Thiem#0843412573#6#330
R#Craig Moore#0721236285#18#T#3#2
Write get methods to return the ArrayLists to the test class.
In the test class:
Write two static methods to display the information of the employees as two separate lists – one for residential and one for business properties.
The method must receive the ArrayList to display and its counter as parameters.
Also display suitable headings and subheadings.
Hint: Use a for loop and the toString() method in each method.
Call the methods from the main method.


Step by step
Solved in 2 steps

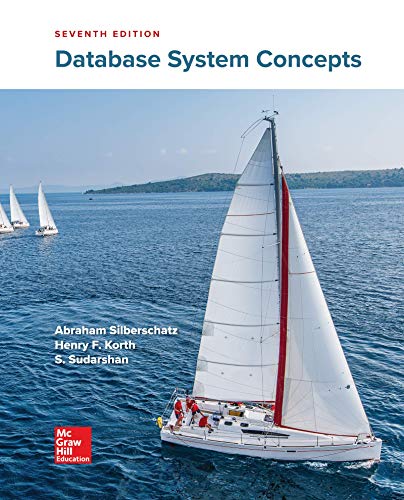
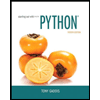
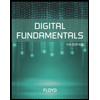
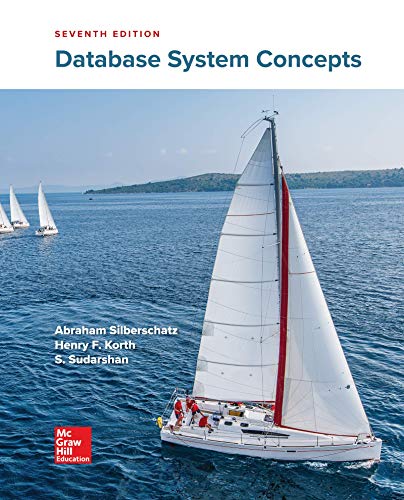
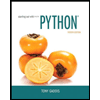
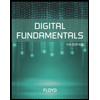
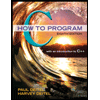
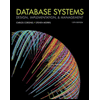
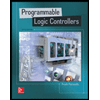