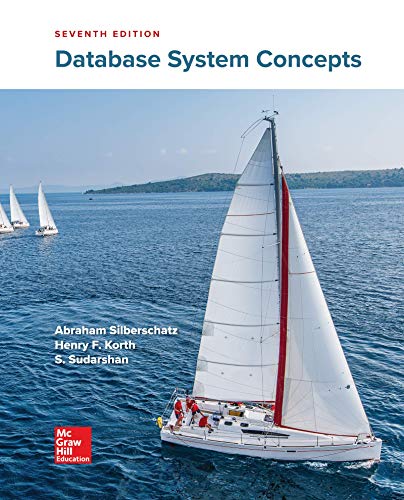
Hello there: I must follow the below instructions very carefully to complete the code. My code is not working. Can you help me to fix it? In the def reverse function, I'm stuck there with the instructions.
Thanks in advanced!
The instructions are in the code comments. Also, you can see some output examples.
import numpy as np
# SIZE is a global constant, use this throughout your
# you should be able to change this to any int and your
# whole program will still work correctly. TEST THIS!
SIZE = 10
STATS_SIZE = 4
MULTIPLIER = 10
# initialize the array with 10, 20, 30, etc.
def make_array():
# creates a numpy array of size SIZE filled with 0s.
nums = np.array([0] * SIZE, dtype=int)
# now fill it with 10,20,30, etc ***using a loop*** and return it
def calc_stats(nums):
stats = np.array([0] * STATS_SIZE, dtype = int)
stats[0] = np.min(nums)
stats[1] = np.max(nums)
stats[2] = np.sum(nums)
stats[3] = int(np.mean(nums))
# make an integer array of size 4 to hold stats
# calculate four basic stats and put them in the size 4 array in this order:
# min, max, total, average
# min and max will just be the first and last numbers from the nums array
# average may calculate as a float, make it an int to store it in the stats array
# return the stats array with all the correct stats in order
def display_stats(stats):
print("Min: ", stats[0])
print("Max; ", stats[1])
print("Total: ", stats[2])
print("Average: ", stats[3])
print()
# make this display everything as shown in the examples
# you can hard code the indexes, you don't need a loop
def reverse_array(arr):
for i in range(SIZE):
nums[i] = (i + 1) * MULTIPLIER
# this will accept an integer array
# do NOT alter the array passed in. use it as-is
# put the numbers from arr in another array in reverse order
# you MUST use a loop
# and return the reversed array
def main():
# this function is complete, DO NOT MODIFY IT
# you may modify it while you program, but when you turn in for grading
# it must be returned to EXACTLY this state
# this will initialize the array with 10,20,30... etc
numbers = make_array()
# show the array
print("All the numbers...")
print(numbers)
print()
# this will calculate 4 stats, min, max, total, average
statistics = calc_stats(numbers)
# this will initialize the array with 10,20,30... etc
display_stats(statistics)
# this will reverse the array
numbers = reverse_array(numbers)
# show the array again
print("All the numbers in revered order...")
print(numbers)
print()
# this starts the program
# do not change this line
main()
Hints
● You can put the line pass in your empty functions as a temporary placeholder to make Python ignore the fact there is no code inside the function. This is so your program won't crash while you work on it. It can be like this: def some_function(): pass
● To start, put pass in your empty functions and comment out all the code in main() except for this part: Then work on just the make_array() function. Get that to work by itself first.
● Similarly, then uncomment the line statistics= calc_stats(numbers) and work on just that function. You can add a debug line below it like this: print(statistics) so you can see what calc_stats() is doing.
● Continue in that way. Uncomment just the next part you want to work on, and add debug statements as needed. Make sure you remove all debug statements before you submit.
● Make sure you return main() back to its original state
![SIZE = 10
All the numbers...
[ 10 20
30 40 50 60
70 80 90 100]
Min: 10
Max:
Total: 550
Average: 55
100
All the numbers in reversed order...
[100 90 80 70 60 50 40 30
20 10]](https://content.bartleby.com/qna-images/question/35aa2439-3fb3-42fb-bba6-4af8bd2f0727/231d4554-a37d-4f4b-8d19-19983818c549/1kswvc.png)
![SIZE = 100
All the numbers...
[ 10
20
30
40
50
60
70
80
90 100 110 120 130 140
150 160 170
180
190 200 210 220 230 240
250 260 270 280
290 300 310 320
330 340 350 360 370 380 390 400 410 420
430 440 450 460 470 480 490 500 510 520 530 540 550 560
570 580 590 600 610 620 630 640 650 660 670 680 690 700
710 720 730 740 750 760 770 780 790 800 810 820 830 840
850 860 870 880 890
900 910 920 930 940 950 960 970 980
990 1000]
Min: 10
Max: 1000
Total: 50500
Average: 505
All the numbers in reversed order...
[1000 990 980 970 960 950 940 930 920 910 900 890 880 870
860 850 840 830 820 810 800 790 780 770 760 750 740 730
720 710 700 690 680 670 660 650 640 630 620 610 600 590
580 570 560 550 540 530 520 510 500 490 480 470 460
450
440 430 420 410 400 390 380 370 360 350 340 330 320 310
190 180 170
30
300 290 280 270 260 250 240 230 220 210 200
160 150 140
130
120 110 100
90
80
70
60
50
40
20
10]](https://content.bartleby.com/qna-images/question/35aa2439-3fb3-42fb-bba6-4af8bd2f0727/231d4554-a37d-4f4b-8d19-19983818c549/1c6cne.png)

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- Hello, can anyone help me with this program please? I need it in C++. I already have a bit of code that was given to me but I'm not sure how to go forward with it. Please help me. Modify the vehicle management program to allow an automobile rental company to manage its fleet of automobiles. First, define a class called CityCar that contains an array of pointers to the 100 objects in the Car class. This also allows you to store pointers to objects of the derived class types PassCar and Truck. The objects themselves will be created dynamically at runtime. Define a class CityCar with an array of pointers to the Car class and an int variable for the current number of elements in the array. The constructor will set the current number of array elements to 0. The destructor must release memory allocated dynamically for the remaining objects. Make sure that you use a virtual destructor definition in the base class Car to allow correct releasing of memory for trucks and passenger vehicles.…arrow_forwardplease anser the practice assgnment questions in th images attached. please leave your answer in C++ code ONLYarrow_forwardIn this lab, you use what you have learned about parallel arrays to complete a partially completed C++ program. The program should: Either print the name and price for a coffee add-in from the Jumpin’ Jive Coffee Shop Or it should print the message Sorry, we do not carry that. Read the problem description carefully before you begin. The file provided for this lab includes the necessary variable declarations and input statements. You need to write the part of the program that searches for the name of the coffee add-in(s) and either prints the name and price of the add-in or prints the error message if the add-in is not found. Comments in the code tell you where to write your statements. Instructions Study the prewritten code to make sure you understand it. Write the code that searches the array for the name of the add-in ordered by the customer. Write the code that prints the name and price of the add-in or the error message, and then write the code that prints the cost of the total…arrow_forward
- I need help writing a C++ code. I need the code to be a class called date that has an integer data members to store month, day, and year. The class should have a three-parameter default constructor that allows the date to be set at the time a new Date Object is created. If the user creates a Date object without passing any arguments, or the values passed are invalid, the default values of 1,1,2001 (i.e, January 1, 2001) should be used. The class should have member functions to print the date in the following formats: 3/15/2020 March 15, 2020 15 March 2020 I also need the program to only accept real values for month and day. By this I mean month 1-12 and date 1- how ever long the month is.arrow_forwardin c++ 1. Write a function that takes a 1 Dimensional array and an integer n andreturns the number of times ‘n’ appears in the array. If ‘n’ does not appearin the array, return -1.2. Write a function that takes a 2 Dimensional array and returns the positionof the first row with an odd sum. Assume that the column size is fixed at 4.If no sum is odd, return -13. Write a class, “pie”, that has a number of slices (int slices) as a privateproperty. Construct the pie with a number of slices and remove a slice witha function. Tell the user how many slices are in the pie.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
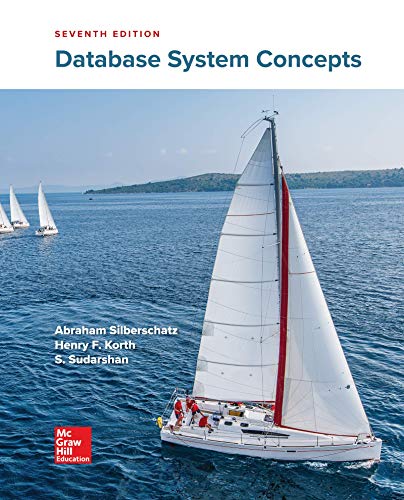
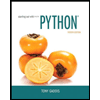
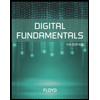
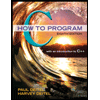
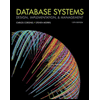
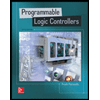