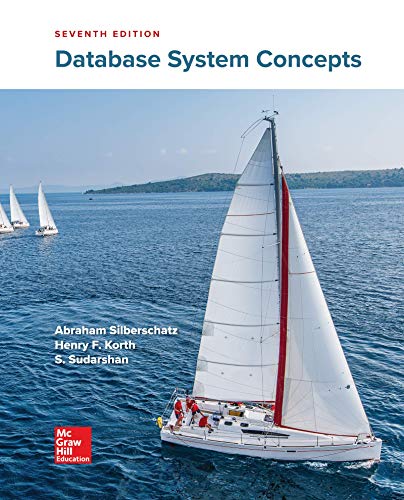
In this lab, you use what you have learned about parallel arrays to complete a partially completed C++
- Either print the name and price for a coffee add-in from the Jumpin’ Jive Coffee Shop
- Or it should print the message Sorry, we do not carry that.
Read the problem description carefully before you begin. The file provided for this lab includes the necessary variable declarations and input statements. You need to write the part of the program that searches for the name of the coffee add-in(s) and either prints the name and price of the add-in or prints the error message if the add-in is not found. Comments in the code tell you where to write your statements.
Instructions
- Study the prewritten code to make sure you understand it.
- Write the code that searches the array for the name of the add-in ordered by the customer.
- Write the code that prints the name and price of the add-in or the error message, and then write the code that prints the cost of the total order.
-
Execute the program by clicking the Run button at the bottom of the screen. Use the following data:
Cream
Caramel
Whiskey
chocolate
Chocolate
Cinnamon
Vanilla
/ JumpinJava.cpp - This program looks up and pr/ints the names and prices of coffee orders.// Input: Interactive// Output: Name and price of coffee orders or error message if add-in is not found
#include <iostream>#include <string>using namespace std;
int main(){// Declare variables.string addIn; // Add-in orderedconst int NUM_ITEMS = 5; // Named constant// Initialized array of add-insstring addIns[] = {"Cream", "Cinnamon", "Chocolate", "Amaretto", "Whiskey"};// Initialized array of add-in pricesdouble addInPrices[] = {.89, .25, .59, 1.50, 1.75};bool foundIt = false; // Flag variableint x; // Loop control variabledouble orderTotal = 2.00; // All orders start with a 2.00 charge
// Get user inputcout << "Enter coffee add-in or XXX to quit: ";cin >> addIn;// Write the rest of the program here.return 0;} // End of main()

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 2 images

- MUST BE WRITTEN IN C++ Write a program so that user can use it to compute and display various statistics for a list of at least 3 values and up to 20 positive real values. The main objectives of this project are to work with menu, arrays, file input, and modules/functions. A menu is available and user can select one of the 4 options from the menu. The program will continue until option 4 is selected. For option 1, process a predefined array in the code with 3 values (73.3 83.4 58.0). User will be able to input an array from the keyboard with a sentinel loop with option 2. Use the following values (73.3 83.4 58.0 11.9 25.1 69.9 45.7 95.0 44.4)as one of your test cases (use-1.0 as a sentinel value). When option 3 is selected, input data from a text file. Use the given p2input.txt Download p2input.txt as a test case. The values in the file are shown below. 73.399.0 83.4 58.0 25.1 69.0 11.9 95.0 74.8 55.0 43.5 47.4 95.6 58.9 52.6 37.7 93.8 19.9 23.9 77.2 You can assume that the user will…arrow_forwardHello, can anyone help me with this program please? I need it in C++. I already have a bit of code that was given to me but I'm not sure how to go forward with it. Please help me. Modify the vehicle management program to allow an automobile rental company to manage its fleet of automobiles. First, define a class called CityCar that contains an array of pointers to the 100 objects in the Car class. This also allows you to store pointers to objects of the derived class types PassCar and Truck. The objects themselves will be created dynamically at runtime. Define a class CityCar with an array of pointers to the Car class and an int variable for the current number of elements in the array. The constructor will set the current number of array elements to 0. The destructor must release memory allocated dynamically for the remaining objects. Make sure that you use a virtual destructor definition in the base class Car to allow correct releasing of memory for trucks and passenger vehicles.…arrow_forwardIn Between Code in C languagearrow_forward
- in the C++ version please suppose to have a score corresponding with probabilities at the end and do not use the count[] function. Please explain the detail when coding. DO NOT USE ARRAY. The game of Pig The game of Pig is a dice game with simple rules: Two players race to reach 100 points. Each turn, a player repeatedly rolls a die until either a 1 ("pig") is rolled or the player holds and scores the sum of the rolls (i.e. the turn total). At any time during a player's turn, the player is faced with two decisions: roll - if the player rolls 1: the player scores nothing and it becomes the opponents turn. 2 - 6: the number is added to the player's turn total and the player's turn continues. hold - The turn total is added to the player's score and it becomes the opponent's turn. This game is a game of probability. Players can use their knowledge of probabilities to make an educated game decision. Assignment specifications Hold-at-20 means that the player will choose to roll…arrow_forwardC++ ONLY PLEASE, and please read carefully, i have had people submit wrong answers Assignment 7 A: Rare Collection. We can make arrays of custom objects just like we’ve done with ints and strings. While it’s possible to make both 1D and 2D arrays of objects (and more), for this assignment we’ll start you out with just one dimensional arrays. Your parents have asked you to develop a program to help them organize the collection of rare CDs they currently have sitting in their car’s glove box. To do this, you will first create an AudioCD class. It should have the following private attributes. String cdTitle String[4] artists int releaseYear String genre float condition Your class should also have the following methods: Default Constructor: Initializes the five attributes to the following default values: ◦ cdTitle = “” ◦ artists = {“”, “”, “”, “”} ◦ releaseYear = 1980 ◦ genre = “” ◦ condition = 0.0 Overloaded Constructor: Initializes the five attributes based on values passed…arrow_forwardattached is sample output needed to complete this C++ assignment. Function definitions needed with the //. please do not use 2d arrays either. Project Description The Lo Shu Magic Square is a grid with 3 rows and 3 columns shown below Write a program that simulates a magic square using 3 one dimensional parallel arrays of integer type. Each one the arrays corresponds to a row of the magic square. The program asks the user to enter the values of the magic square row by row and informs the user if the grid is a magic square or not. ----------------------------------------------------------------- the code template is as follows ---------------------------------------------- #include<iostream> using namespace std; // Global constants const int ROWS = 3; // The number of rows in the array const int COLS = 3; // The number of columns in the array const int MIN = 1; // The value of the smallest number const int MAX = 9; // The value of the largest number // Function…arrow_forward
- In this lab, you use what you have learned about parallel arrays to complete a partially completed C++ program. The program should: Either print the name and price for a coffee add-in from the Jumpin’ Jive Coffee Shop Or it should print the message Sorry, we do not carry that. Read the problem description carefully before you begin. The file provided for this lab includes the necessary variable declarations and input statements. You need to write the part of the program that searches for the name of the coffee add-in(s) and either prints the name and price of the add-in or prints the error message if the add-in is not found. Comments in the code tell you where to write your statements. Instructions Study the prewritten code to make sure you understand it. Write the code that searches the array for the name of the add-in ordered by the customer. Write the code that prints the name and price of the add-in or the error message, and then write the code that prints the cost of the total…arrow_forwardLanguage is C++arrow_forwardIn this lab, you use what you have learned about parallel arrays to complete a partially completed C++ program. The program should: Either print the name and price for a coffee add-in from the Jumpin’ Jive Coffee Shop Or it should print the message Sorry, we do not carry that. Read the problem description carefully before you begin. The file provided for this lab includes the necessary variable declarations and input statements. You need to write the part of the program that searches for the name of the coffee add-in(s) and either prints the name and price of the add-in or prints the error message if the add-in is not found. Comments in the code tell you where to write your statements. Instructions Study the prewritten code to make sure you understand it. Write the code that searches the array for the name of the add-in ordered by the customer. Write the code that prints the name and price of the add-in or the error message, and then write the code that prints the cost of the total…arrow_forward
- C++ HELP In this lab, you're going to be working with partially filled arrays that are parallel with each other. That means that the row index in multiple arrays identifies different pieces of data for the same person. This is a simple payroll system that just calculates gross pay given a set of employees, hours worked for the week and hourly rate. Parallel Arrays First, you have to define several arrays in your main program employee names for each employee hourly pay rate for each employee total hours worked for each employee gross pay for each employee You can use a global SIZE of 50 to initialize these arrays Second, you will need a two dimension (2-D) array to record the hours worked each day for an employee. The number of rows for the 2-D array should be the same as the arrays above since each row corresponds to an employee. The number of columns represents days of the week (7 last I looked) Functions Needed In this lab, you must read in the employee names first because this…arrow_forwardhelp me solve this in c++ please Write a program that asks the user to enter a list of numbers from 1 to 9 in random order, creates and displays the corresponding 3 by 3 square, and determines whether the resulting square is a Lo Shu Magic Square. Notes Create the square by filling the numbers entered from left to right, top to bottom. Input validation - Do not accept numbers outside the range. Do not accept repeats. Must use two-dimensional arrays in the implementation. Functional decomposition — Program should rely on functions that are consistent with the algorithm.arrow_forwardThe Lo Shu Magic Square is a grid with 3 rows and 3 columns shown below. The Lo Shu Magic Square has the following properties: The grid contains the numbers 1 – 9 exactly The sum of each row, each column and each diagonal all add up to the same number. This is shown below: Write a program that simulates a magic square using 3 one dimensional parallel arrays of integer type. Do not use two-dimensional array. Each one the arrays corresponds to a row of the magic square. The program asks the user to enter the values of the magic square row by row and informs the user if the grid is a magic square or not. Processing Requirements - c++ Use the following template to start your project: #include<iostream> using namespace std; // Global constants const int ROWS = 3; // The number of rows in the array const int COLS = 3; // The number of columns in the array const int MIN = 1; // The value of the smallest number const int MAX = 9; // The value of the largest number //…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
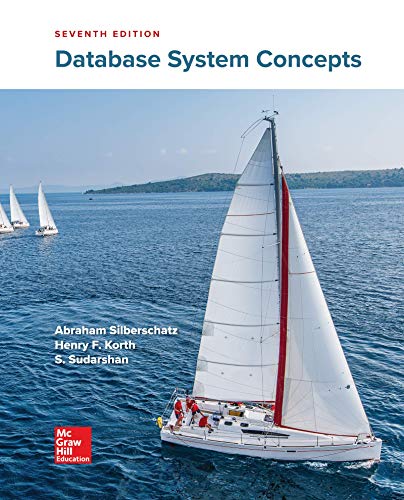
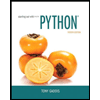
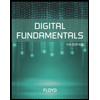
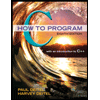
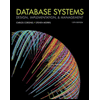
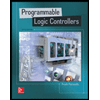